In [1]:
import gitreleases
gitreleases.get_release_info()
gitreleases.clone_releases()
In [3]:
from __future__ import print_function
import os
import json
import re
import sys
import pandas
from datetime import datetime, timedelta
from time import sleep
from subprocess import check_output
try:
from urllib import urlopen
except:
from urllib.request import urlopen
import ssl
#import yaml
context = ssl._create_unverified_context()
In [4]:
with open('package_versions.txt', 'r') as package_list:
packages = dict([line.strip().split() for line in package_list.readlines()])
In [5]:
packages
Out[5]:
{'libpysal': '4.2.1',
'esda': '2.2.0',
'giddy': '2.3.0',
'inequality': '1.0.0',
'pointpats': '2.1.0',
'segregation': '1.1.1',
'spaghetti': '1.4.0',
'mgwr': '2.1.1',
'spglm': '1.0.7',
'spint': '1.0.6',
'spreg': '1.0.4',
'spvcm': '0.2.1.post1',
'tobler': '0.2.0',
'mapclassify': '2.2.0',
'splot': '1.1.2'}
In [6]:
release_date = '2020-01-31'
start_date = '2019-07-31'
In [7]:
since_date = '--since="{start}"'.format(start=start_date)
since_date
since = datetime.strptime(start_date+" 0:0:0", "%Y-%m-%d %H:%M:%S")
since
Out[7]:
datetime.datetime(2019, 7, 31, 0, 0)
In [ ]:
In [8]:
cmd = ['git', 'log', '--format=* %aN', since_date]
In [9]:
CWD = os.path.abspath(os.path.curdir)
In [10]:
CWD
Out[10]:
'/home/serge/Dropbox/p/pysal/src/pysal/tools'
In [11]:
os.chdir('tmp/esda')
os.getcwd()
Out[11]:
'/home/serge/Dropbox/p/pysal/src/pysal/tools/tmp/esda'
In [12]:
ncommits = len(check_output(cmd).splitlines())
In [13]:
ncommits
Out[13]:
17
In [14]:
identities = {'Levi John Wolf': ('ljwolf', 'Levi John Wolf'),
'Serge Rey': ('Serge Rey', 'Sergio Rey', 'sjsrey', 'serge'),
'Wei Kang': ('Wei Kang', 'weikang9009'),
'Dani Arribas-Bel': ('Dani Arribas-Bel', 'darribas')
}
def regularize_identity(string):
string = string.decode()
for name, aliases in identities.items():
for alias in aliases:
if alias in string:
string = string.replace(alias, name)
if len(string.split(' '))>1:
string = string.title()
return string.lstrip('* ')
In [15]:
from collections import Counter
author_cmd = ['git', 'log', '--format=* %aN', since_date]
In [16]:
ncommits = len(check_output(cmd).splitlines())
all_authors = check_output(author_cmd).splitlines()
counter = Counter([regularize_identity(author) for author in all_authors])
# global_counter += counter
# counters.update({'.'.join((package,subpackage)): counter})
unique_authors = sorted(set(all_authors))
In [17]:
unique_authors
Out[17]:
[b'* James Gaboardi',
b'* Serge Rey',
b'* Sergio Rey',
b'* Wei Kang',
b'* ljwolf']
In [18]:
counter.keys()
Out[18]:
dict_keys(['Serge Rey', 'Levi John Wolf', 'James Gaboardi', 'Wei Kang'])
In [ ]:
In [ ]:
In [19]:
from datetime import datetime, timedelta
ISO8601 = "%Y-%m-%dT%H:%M:%SZ"
PER_PAGE = 100
element_pat = re.compile(r'<(.+?)>')
rel_pat = re.compile(r'rel=[\'"](\w+)[\'"]')
In [20]:
def parse_link_header(headers):
link_s = headers.get('link', '')
urls = element_pat.findall(link_s)
rels = rel_pat.findall(link_s)
d = {}
for rel,url in zip(rels, urls):
d[rel] = url
return d
def get_paged_request(url):
"""get a full list, handling APIv3's paging"""
results = []
while url:
#print("fetching %s" % url, file=sys.stderr)
f = urlopen(url)
results.extend(json.load(f))
links = parse_link_header(f.headers)
url = links.get('next')
return results
def get_issues(project="pysal/pysal", state="closed", pulls=False):
"""Get a list of the issues from the Github API."""
which = 'pulls' if pulls else 'issues'
url = "https://api.github.com/repos/%s/%s?state=%s&per_page=%i" % (project, which, state, PER_PAGE)
return get_paged_request(url)
def _parse_datetime(s):
"""Parse dates in the format returned by the Github API."""
if s:
return datetime.strptime(s, ISO8601)
else:
return datetime.fromtimestamp(0)
def issues2dict(issues):
"""Convert a list of issues to a dict, keyed by issue number."""
idict = {}
for i in issues:
idict[i['number']] = i
return idict
def is_pull_request(issue):
"""Return True if the given issue is a pull request."""
return 'pull_request_url' in issue
def issues_closed_since(period=timedelta(days=365), project="pysal/pysal", pulls=False):
"""Get all issues closed since a particular point in time. period
can either be a datetime object, or a timedelta object. In the
latter case, it is used as a time before the present."""
which = 'pulls' if pulls else 'issues'
if isinstance(period, timedelta):
period = datetime.now() - period
url = "https://api.github.com/repos/%s/%s?state=closed&sort=updated&since=%s&per_page=%i" % (project, which, period.strftime(ISO8601), PER_PAGE)
allclosed = get_paged_request(url)
# allclosed = get_issues(project=project, state='closed', pulls=pulls, since=period)
filtered = [i for i in allclosed if _parse_datetime(i['closed_at']) > period]
# exclude rejected PRs
if pulls:
filtered = [ pr for pr in filtered if pr['merged_at'] ]
return filtered
def sorted_by_field(issues, field='closed_at', reverse=False):
"""Return a list of issues sorted by closing date date."""
return sorted(issues, key = lambda i:i[field], reverse=reverse)
def report(issues, show_urls=False):
"""Summary report about a list of issues, printing number and title.
"""
# titles may have unicode in them, so we must encode everything below
if show_urls:
for i in issues:
role = 'ghpull' if 'merged_at' in i else 'ghissue'
print('* :%s:`%d`: %s' % (role, i['number'],
i['title'].encode('utf-8')))
else:
for i in issues:
print('* %d: %s' % (i['number'], i['title'].encode('utf-8')))
In [23]:
since
Out[23]:
datetime.datetime(2019, 7, 31, 0, 0)
In [24]:
all_issues = {}
all_pulls = {}
total_commits = 0
issue_details = {}
pull_details = {}
for package in packages:
subpackage = package
subpackages = packages[package].split()
print(package)
prj = 'pysal/{subpackage}'.format(subpackage=package)
os.chdir(CWD)
os.chdir('tmp/{subpackage}'.format(subpackage=package))
issues = issues_closed_since(since, project=prj,pulls=False)
pulls = issues_closed_since(since, project=prj,pulls=True)
issues = sorted_by_field(issues, reverse=True)
pulls = sorted_by_field(pulls, reverse=True)
issue_details[subpackage] = issues
pull_details[subpackage] = pulls
n_issues, n_pulls = map(len, (issues, pulls))
n_total = n_issues + n_pulls
all_issues[subpackage] = n_total, n_pulls
os.chdir(CWD)
libpysal
esda
giddy
inequality
pointpats
segregation
spaghetti
mgwr
spglm
spint
spreg
spvcm
tobler
mapclassify
splot
In [25]:
issue_details
Out[25]:
{'libpysal': [{'url': 'https://api.github.com/repos/pysal/libpysal/issues/230',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/230/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/230/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/230/events',
'html_url': 'https://github.com/pysal/libpysal/pull/230',
'id': 545322537,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjQwMTk5',
'number': 230,
'title': 'raise warning when islands are used in to_adjlist',
'user': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-04T19:25:12Z',
'updated_at': '2020-01-04T20:29:26Z',
'closed_at': '2020-01-04T20:29:26Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/230',
'html_url': 'https://github.com/pysal/libpysal/pull/230',
'diff_url': 'https://github.com/pysal/libpysal/pull/230.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/230.patch'},
'body': 'This addresses issue #218 '},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/113',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/113/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/113/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/113/events',
'html_url': 'https://github.com/pysal/libpysal/issues/113',
'id': 367577847,
'node_id': 'MDU6SXNzdWUzNjc1Nzc4NDc=',
'number': 113,
'title': 'Some example datasets are missing documentation',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."},
{'id': 1376367312,
'node_id': 'MDU6TGFiZWwxMzc2MzY3MzEy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/docs',
'name': 'docs',
'color': 'ffc8b2',
'default': False,
'description': ''},
{'id': 1685679755,
'node_id': 'MDU6TGFiZWwxNjg1Njc5NzU1',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/examples',
'name': 'examples',
'color': '135699',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/2',
'html_url': 'https://github.com/pysal/libpysal/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/2/labels',
'id': 3717949,
'node_id': 'MDk6TWlsZXN0b25lMzcxNzk0OQ==',
'number': 2,
'title': '2.0',
'description': None,
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 2,
'state': 'closed',
'created_at': '2018-10-07T19:19:51Z',
'updated_at': '2020-01-04T19:54:39Z',
'due_on': None,
'closed_at': '2019-09-09T11:43:51Z'},
'comments': 4,
'created_at': '2018-10-07T18:00:21Z',
'updated_at': '2020-01-04T19:52:30Z',
'closed_at': '2020-01-04T19:52:30Z',
'author_association': 'MEMBER',
'body': '- tokyo\r\n- berlin\r\n- georgia\r\n- nyc_bikes\r\n- clearwater'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/229',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/229/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/229/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/229/events',
'html_url': 'https://github.com/pysal/libpysal/pull/229',
'id': 545321825,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjM5NjU3',
'number': 229,
'title': 'DOC: Cleaning up docs and docsr for tutorial',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-04T19:17:54Z',
'updated_at': '2020-01-04T19:35:54Z',
'closed_at': '2020-01-04T19:35:54Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/229',
'html_url': 'https://github.com/pysal/libpysal/pull/229',
'diff_url': 'https://github.com/pysal/libpysal/pull/229.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/229.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/165',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/165/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/165/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/165/events',
'html_url': 'https://github.com/pysal/libpysal/issues/165',
'id': 466350029,
'node_id': 'MDU6SXNzdWU0NjYzNTAwMjk=',
'number': 165,
'title': '`to_adjlist(remove_symmetric=True)` fails on string-indexed weights. ',
'user': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1000799086,
'node_id': 'MDU6TGFiZWwxMDAwNzk5MDg2',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/good%20first%20contribution',
'name': 'good first contribution',
'color': 'a2eaef',
'default': False,
'description': ''},
{'id': 536186560,
'node_id': 'MDU6TGFiZWw1MzYxODY1NjA=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/help%20wanted',
'name': 'help wanted',
'color': '128A0C',
'default': True,
'description': None},
{'id': 1685673071,
'node_id': 'MDU6TGFiZWwxNjg1NjczMDcx',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/weights',
'name': 'weights',
'color': 'e2ab73',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2019-07-10T14:41:08Z',
'updated_at': '2020-01-04T19:26:54Z',
'closed_at': '2020-01-04T19:26:54Z',
'author_association': 'MEMBER',
'body': "# to replicate\r\n```python\r\nimport libpysal\r\nw = libpysal.weights.lat2W(4,4, id_type='string')\r\nw.to_adjlist(remove_symmetric=True)\r\n```\r\n# to fix\r\nfigure out how to conduct that removal query in line 188 of `adjtools.py`. I think this might be fixed using:\r\n```python\r\nto_drop_mask = (adjlist.focal == tupe[0]) & (adjlist.neighbor == tupe[-1])\r\nto_drop = adjlist[to_drop_mask]\r\n```\r\nthat's probably both faster & better. "},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/204',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/204/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/204/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/204/events',
'html_url': 'https://github.com/pysal/libpysal/issues/204',
'id': 516321706,
'node_id': 'MDU6SXNzdWU1MTYzMjE3MDY=',
'number': 204,
'title': "AttributeError: 'Queen' object has no attribute 'silent_island_warning'",
'user': {'login': 'TransUMD',
'id': 29628412,
'node_id': 'MDQ6VXNlcjI5NjI4NDEy',
'avatar_url': 'https://avatars0.githubusercontent.com/u/29628412?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/TransUMD',
'html_url': 'https://github.com/TransUMD',
'followers_url': 'https://api.github.com/users/TransUMD/followers',
'following_url': 'https://api.github.com/users/TransUMD/following{/other_user}',
'gists_url': 'https://api.github.com/users/TransUMD/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/TransUMD/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/TransUMD/subscriptions',
'organizations_url': 'https://api.github.com/users/TransUMD/orgs',
'repos_url': 'https://api.github.com/users/TransUMD/repos',
'events_url': 'https://api.github.com/users/TransUMD/events{/privacy}',
'received_events_url': 'https://api.github.com/users/TransUMD/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1685673071,
'node_id': 'MDU6TGFiZWwxNjg1NjczMDcx',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/weights',
'name': 'weights',
'color': 'e2ab73',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 11,
'created_at': '2019-11-01T20:09:44Z',
'updated_at': '2020-01-04T19:22:08Z',
'closed_at': '2020-01-04T19:22:08Z',
'author_association': 'NONE',
'body': "Hello,\r\n\r\nI was using libpysal to conduct esda. I was doing the spatial weights using continuity weights but it pops up the following errors:\r\n\r\n---------------------------------------------------------------------------\r\nAttributeError Traceback (most recent call last)\r\n<ipython-input-189-9a78d6d6df16> in <module>\r\n 13 break\r\n 14 c_type = crime_type[i*2+j]\r\n---> 15 li_bg = esda.moran.Moran_Local(blgp_crime[c_type], qW_blgp)\r\n 16 mi_bg = esda.moran.Moran(blgp_crime[c_type], qW_blgp)\r\n 17 sig = 1 * (li_bg.p_sim < 0.05)\r\n\r\nE:\\Anaconda\\envs\\incenTrip\\lib\\site-packages\\pysal\\explore\\esda\\moran.py in __init__(self, y, w, transformation, permutations, geoda_quads)\r\n 870 np.seterr(**orig_settings)\r\n 871 self.z = z\r\n--> 872 w.transform = transformation\r\n 873 self.w = w\r\n 874 self.permutations = permutations\r\n\r\nE:\\Anaconda\\envs\\incenTrip\\lib\\site-packages\\libpysal\\weights\\weights.py in set_transform(self, value)\r\n 943 row_sum = sum(wijs) * 1.0\r\n 944 if row_sum == 0.0:\r\n--> 945 if not self.silent_island_warning:\r\n 946 print(('WARNING: ', i, ' is an island (no neighbors)'))\r\n 947 weights[i] = [wij / row_sum for wij in wijs]\r\n\r\nAttributeError: 'Queen' object has no attribute 'silent_island_warning'\r\n\r\nI check the raw code and can't find things about silent_island_warning.\r\n\r\nThank you.\r\n\r\nBest,\r\n"},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/226',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/226/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/226/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/226/events',
'html_url': 'https://github.com/pysal/libpysal/pull/226',
'id': 545316618,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjM1ODQx',
'number': 226,
'title': '4.2.1',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-04T18:37:02Z',
'updated_at': '2020-01-04T19:21:39Z',
'closed_at': '2020-01-04T19:21:39Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/226',
'html_url': 'https://github.com/pysal/libpysal/pull/226',
'diff_url': 'https://github.com/pysal/libpysal/pull/226.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/226.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/228',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/228/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/228/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/228/events',
'html_url': 'https://github.com/pysal/libpysal/pull/228',
'id': 545321444,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjM5Mzc3',
'number': 228,
'title': 'Revert "4.2.1"',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-04T19:14:13Z',
'updated_at': '2020-01-04T19:14:22Z',
'closed_at': '2020-01-04T19:14:22Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/228',
'html_url': 'https://github.com/pysal/libpysal/pull/228',
'diff_url': 'https://github.com/pysal/libpysal/pull/228.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/228.patch'},
'body': 'Reverts pysal/libpysal#227'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/227',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/227/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/227/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/227/events',
'html_url': 'https://github.com/pysal/libpysal/pull/227',
'id': 545321304,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjM5Mjc3',
'number': 227,
'title': '4.2.1',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-04T19:13:02Z',
'updated_at': '2020-01-04T19:13:18Z',
'closed_at': '2020-01-04T19:13:18Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/227',
'html_url': 'https://github.com/pysal/libpysal/pull/227',
'diff_url': 'https://github.com/pysal/libpysal/pull/227.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/227.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/225',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/225/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/225/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/225/events',
'html_url': 'https://github.com/pysal/libpysal/pull/225',
'id': 545315262,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjM0OTE1',
'number': 225,
'title': 'DOC: images for notebooks',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-04T18:22:50Z',
'updated_at': '2020-01-04T18:22:59Z',
'closed_at': '2020-01-04T18:22:59Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/225',
'html_url': 'https://github.com/pysal/libpysal/pull/225',
'diff_url': 'https://github.com/pysal/libpysal/pull/225.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/225.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/224',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/224/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/224/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/224/events',
'html_url': 'https://github.com/pysal/libpysal/pull/224',
'id': 545314858,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjM0NjQx',
'number': 224,
'title': '4.2.1',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-04T18:19:15Z',
'updated_at': '2020-01-04T18:19:30Z',
'closed_at': '2020-01-04T18:19:30Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/224',
'html_url': 'https://github.com/pysal/libpysal/pull/224',
'diff_url': 'https://github.com/pysal/libpysal/pull/224.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/224.patch'},
'body': 'Getting docs and docsrc in sync with notebooks'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/223',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/223/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/223/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/223/events',
'html_url': 'https://github.com/pysal/libpysal/pull/223',
'id': 545312891,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjMzMzI3',
'number': 223,
'title': '4.2.1',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-04T18:00:09Z',
'updated_at': '2020-01-04T18:00:19Z',
'closed_at': '2020-01-04T18:00:19Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/223',
'html_url': 'https://github.com/pysal/libpysal/pull/223',
'diff_url': 'https://github.com/pysal/libpysal/pull/223.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/223.patch'},
'body': 'Fixing notebooks for tutorial'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/221',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/221/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/221/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/221/events',
'html_url': 'https://github.com/pysal/libpysal/issues/221',
'id': 545306177,
'node_id': 'MDU6SXNzdWU1NDUzMDYxNzc=',
'number': 221,
'title': 'duplicate pypi package badge',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1768401718,
'node_id': 'MDU6TGFiZWwxNzY4NDAxNzE4',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/discussion',
'name': 'discussion',
'color': '0e3075',
'default': False,
'description': ''},
{'id': 1376367312,
'node_id': 'MDU6TGFiZWwxMzc2MzY3MzEy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/docs',
'name': 'docs',
'color': 'ffc8b2',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 2,
'created_at': '2020-01-04T16:54:14Z',
'updated_at': '2020-01-04T17:46:59Z',
'closed_at': '2020-01-04T17:46:59Z',
'author_association': 'MEMBER',
'body': 'The `pypi package` badge is [`README.rst`](https://github.com/pysal/libpysal/blob/master/README.rst) is duplicated.\r\n\r\nChoices here include:\r\n* Replacing with a badge from...\r\n * conda-forge/Anaconda, i.e. [](https://anaconda.org/conda-forge/libpysal)\r\n * pypi/conda-forge downloads, i.e. [](https://pepy.tech/project/libpysal)\r\n * as a side note the current `pip install pysal` downloads are: [](https://pepy.tech/project/pysal)\r\n * AppVeyor/Circle CI following #219 \r\n* Simply removing because not everyone is as big a fan of badges as I am.'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/222',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/222/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/222/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/222/events',
'html_url': 'https://github.com/pysal/libpysal/pull/222',
'id': 545308995,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjMwNTM2',
'number': 222,
'title': '4.2.1',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1376367312,
'node_id': 'MDU6TGFiZWwxMzc2MzY3MzEy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/docs',
'name': 'docs',
'color': 'ffc8b2',
'default': False,
'description': ''},
{'id': 536186559,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTk=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2020-01-04T17:20:56Z',
'updated_at': '2020-01-04T17:46:31Z',
'closed_at': '2020-01-04T17:46:31Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/222',
'html_url': 'https://github.com/pysal/libpysal/pull/222',
'diff_url': 'https://github.com/pysal/libpysal/pull/222.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/222.patch'},
'body': 'This is adding a tutorial to the docs.'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/220',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/220/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/220/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/220/events',
'html_url': 'https://github.com/pysal/libpysal/pull/220',
'id': 545301757,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjI1NDU1',
'number': 220,
'title': 'REL: 4.2.1',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1768348272,
'node_id': 'MDU6TGFiZWwxNzY4MzQ4Mjcy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/release',
'name': 'release',
'color': '96f7ef',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-04T16:11:16Z',
'updated_at': '2020-01-04T16:16:49Z',
'closed_at': '2020-01-04T16:16:49Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/220',
'html_url': 'https://github.com/pysal/libpysal/pull/220',
'diff_url': 'https://github.com/pysal/libpysal/pull/220.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/220.patch'},
'body': "# Version 4.2.1 (2020-01-04)\r\n\r\nThis is a bug fix release.\r\n\r\nWe closed a total of 14 issues (enhancements and bug fixes) through 5 pull requests, since our last release on 2019-12-14.\r\n\r\n## Issues Closed\r\n - libpysal 4.2.0 won't import on Windows (#214)\r\n - libpysal 4.2.0 Windows import issue (#215)\r\n - Constructing contiguity spatial weights using from_dataframe and from_shapefile could give different results (#212)\r\n - fix bug 212 (#213)\r\n - alpha_shapes docs not rendering (#216)\r\n - corrected docstrings in cg.alpha_shapes.py (#217)\r\n - Updating requirements (#211)\r\n - Big tarball (#174)\r\n - Fetch (#176)\r\n\r\n## Pull Requests\r\n - libpysal 4.2.0 Windows import issue (#215)\r\n - fix bug 212 (#213)\r\n - corrected docstrings in cg.alpha_shapes.py (#217)\r\n - Updating requirements (#211)\r\n - Fetch (#176)\r\n\r\nThe following individuals contributed to this release:\r\n\r\n - Serge Rey\r\n - James Gaboardi\r\n - Levi John Wolf"},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/214',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/214/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/214/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/214/events',
'html_url': 'https://github.com/pysal/libpysal/issues/214',
'id': 543571049,
'node_id': 'MDU6SXNzdWU1NDM1NzEwNDk=',
'number': 214,
'title': "libpysal 4.2.0 won't import on Windows",
'user': {'login': 'martinfleis',
'id': 36797143,
'node_id': 'MDQ6VXNlcjM2Nzk3MTQz',
'avatar_url': 'https://avatars2.githubusercontent.com/u/36797143?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/martinfleis',
'html_url': 'https://github.com/martinfleis',
'followers_url': 'https://api.github.com/users/martinfleis/followers',
'following_url': 'https://api.github.com/users/martinfleis/following{/other_user}',
'gists_url': 'https://api.github.com/users/martinfleis/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/martinfleis/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/martinfleis/subscriptions',
'organizations_url': 'https://api.github.com/users/martinfleis/orgs',
'repos_url': 'https://api.github.com/users/martinfleis/repos',
'events_url': 'https://api.github.com/users/martinfleis/events{/privacy}',
'received_events_url': 'https://api.github.com/users/martinfleis/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 2,
'created_at': '2019-12-29T21:11:11Z',
'updated_at': '2020-01-04T15:28:48Z',
'closed_at': '2020-01-04T15:28:47Z',
'author_association': 'CONTRIBUTOR',
'body': 'Hi, since the latest release libpysal will not import on windows due to encoding error. It raises `UnicodeDecodeError: \'charmap\' codec can\'t decode byte 0x9d in position 503: character maps to <undefined>`. \r\n\r\nDetails: https://ci.appveyor.com/project/martinfleis/momepy/builds/29802733\r\n\r\nHighly likely caused by #176. I assume that adding `encoding="utf8"` to `open()` on lines 50 and 56 will fix the issue. I am unable to try that locally as I do not have windows machine until 6th of January.'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/215',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/215/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/215/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/215/events',
'html_url': 'https://github.com/pysal/libpysal/pull/215',
'id': 543649119,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU3OTEwODU0',
'number': 215,
'title': 'libpysal 4.2.0 Windows import issue',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."},
{'id': 1685679755,
'node_id': 'MDU6TGFiZWwxNjg1Njc5NzU1',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/examples',
'name': 'examples',
'color': '135699',
'default': False,
'description': ''},
{'id': 1685690032,
'node_id': 'MDU6TGFiZWwxNjg1NjkwMDMy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/io',
'name': 'io',
'color': 'e8ac35',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 6,
'created_at': '2019-12-30T00:50:12Z',
'updated_at': '2020-01-04T15:28:09Z',
'closed_at': '2020-01-04T15:28:09Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/215',
'html_url': 'https://github.com/pysal/libpysal/pull/215',
'diff_url': 'https://github.com/pysal/libpysal/pull/215.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/215.patch'},
'body': 'This PR addresses:\r\n * pysal/libpysal#214\r\n\r\nWith the following machine `libpysal` can be read in:\r\n```python\r\nIn [1]: %load_ext watermark \r\nIn [2]: %watermark\r\n2019-12-29T19:35:00-05:00\r\n\r\nCPython 3.7.5\r\nIPython 7.10.2\r\n\r\ncompiler : MSC v.1916 64 bit (AMD64)\r\nsystem : Windows\r\nrelease : 10\r\nmachine : AMD64\r\nprocessor : Intel64 Family 6 Model 61 Stepping 4, GenuineIntel\r\nCPU cores : 1\r\ninterpreter: 64bit\r\n```\r\n\r\n**However**, the following errors/failures occur ***in windows*** during local `nosetests` (three of which also occur ***in macOS***[see bottom]):\r\n\r\n```\r\n\r\n======================================================================\r\nERROR: test_casting (libpysal.io.iohandlers.tests.test_csvWrapper.test_csvWrapper)\r\n----------------------------------------------------------------------\r\nTraceback (most recent call last):\r\n File "C:\\Users\\200059719\\libpysal\\libpysal\\io\\iohandlers\\tests\\test_csvWrapper.py", line 47, in test_casting\r\n self.obj.cast(\'WKT\', WKTParser())\r\n File "C:\\Users\\200059719\\libpysal\\libpysal\\io\\fileio.py", line 230, in cast\r\n raise KeyError("%s" % key)\r\nKeyError: \'WKT\'\r\n\r\n======================================================================\r\nERROR: test_deserialize (libpysal.io.iohandlers.tests.test_db.Test_sqlite_reader)\r\n----------------------------------------------------------------------\r\nTraceback (most recent call last):\r\n File "C:\\Users\\200059719\\libpysal\\libpysal\\io\\iohandlers\\tests\\test_db.py", line 54, in tearDown\r\n os.remove(\'test.db\')\r\nPermissionError: [WinError 32] The process cannot access the file because it is being used by another process: \'test.db\'\r\n\r\n======================================================================\r\nERROR: test_writeNones (libpysal.io.iohandlers.tests.test_pyDbfIO.test_DBF)\r\n----------------------------------------------------------------------\r\nTraceback (most recent call last):\r\n File "C:\\Users\\200059719\\libpysal\\libpysal\\io\\iohandlers\\tests\\test_pyDbfIO.py", line 115, in test_writeNones\r\n os.remove(fname)\r\nPermissionError: [WinError 32] The process cannot access the file because it is being used by another process: \'C:\\\\Users\\\\200059~1\\\\AppData\\\\Local\\\\Temp\\\\tmpzax2d812.dbf\'\r\n\r\n======================================================================\r\nERROR: test_true_rook2 (libpysal.weights.tests.test__contW_lists.TestContiguityWeights)\r\n----------------------------------------------------------------------\r\nTraceback (most recent call last):\r\n File "C:\\Users\\200059719\\libpysal\\libpysal\\weights\\tests\\test__contW_lists.py", line 75, in test_true_rook2\r\n \'stl_hom.shp\'), ROOK, \'POLY_ID_OG\')\r\n File "C:\\Users\\200059719\\libpysal\\libpysal\\weights\\tests\\test__contW_lists.py", line 120, in build_W\r\n ids = db.by_col[idVariable]\r\n File "C:\\Users\\200059719\\libpysal\\libpysal\\io\\tables.py", line 21, in __getitem__\r\n return self.p._get_col(key)\r\n File "C:\\Users\\200059719\\libpysal\\libpysal\\io\\iohandlers\\pyDbfIO.py", line 110, in _get_col\r\n raise AttributeError(\'Field: % s does not exist in header\' % key)\r\nAttributeError: Field: POLY_ID_OG does not exist in header\r\n\r\n======================================================================\r\nFAIL: Doctest: libpysal.cg.shapely_ext.buffer\r\n----------------------------------------------------------------------\r\nTraceback (most recent call last):\r\n File "C:\\Users\\200059719\\AppData\\Local\\conda\\conda\\envs\\test_momepy\\lib\\doctest.py", line 2196, in runTest\r\n raise self.failureException(self.format_failure(new.getvalue()))\r\nAssertionError: Failed doctest test for libpysal.cg.shapely_ext.buffer\r\n File "C:\\Users\\200059719\\libpysal\\libpysal\\cg\\shapely_ext.py", line unknown line number, in buffer\r\n\r\n----------------------------------------------------------------------\r\nFile "C:\\Users\\200059719\\libpysal\\libpysal\\cg\\shapely_ext.py", line ?, in libpysal.cg.shapely_ext.buffer\r\nFailed example:\r\n g.buffer(1.0).area # 16-gon approx of a unit radius circle\r\nExpected:\r\n 3.1365484905459389\r\nGot:\r\n 3.1365484905459384\r\n----------------------------------------------------------------------\r\nFile "C:\\Users\\200059719\\libpysal\\libpysal\\cg\\shapely_ext.py", line ?, in libpysal.cg.shapely_ext.buffer\r\nFailed example:\r\n g.buffer(1.0, 128).area # 128-gon approximation\r\nExpected:\r\n 3.1415138011443009\r\nGot:\r\n 3.141513801144299\r\n----------------------------------------------------------------------\r\nFile "C:\\Users\\200059719\\libpysal\\libpysal\\cg\\shapely_ext.py", line ?, in libpysal.cg.shapely_ext.buffer\r\nFailed example:\r\n list(g.buffer(1.0, cap_style=\'square\').exterior.coords)\r\nException raised:\r\n Traceback (most recent call last):\r\n File "C:\\Users\\200059719\\AppData\\Local\\conda\\conda\\envs\\test_momepy\\lib\\doctest.py", line 1329, in __run\r\n compileflags, 1), test.globs)\r\n File "<doctest libpysal.cg.shapely_ext.buffer[5]>", line 1, in <module>\r\n list(g.buffer(1.0, cap_style=\'square\').exterior.coords)\r\n File "C:\\Users\\200059719\\AppData\\Local\\conda\\conda\\envs\\test_momepy\\lib\\site-packages\\shapely\\geometry\\base.py", line 593, in buffer\r\n mitre_limit))\r\n File "C:\\Users\\200059719\\AppData\\Local\\conda\\conda\\envs\\test_momepy\\lib\\site-packages\\shapely\\topology.py", line 78, in __call__\r\n return self.fn(this._geom, *args)\r\n ctypes.ArgumentError: argument 5: <class \'TypeError\'>: wrong type\r\n----------------------------------------------------------------------\r\nFile "C:\\Users\\200059719\\libpysal\\libpysal\\cg\\shapely_ext.py", line ?, in libpysal.cg.shapely_ext.buffer\r\nFailed example:\r\n g.buffer(1.0, cap_style=\'square\').area\r\nException raised:\r\n Traceback (most recent call last):\r\n File "C:\\Users\\200059719\\AppData\\Local\\conda\\conda\\envs\\test_momepy\\lib\\doctest.py", line 1329, in __run\r\n compileflags, 1), test.globs)\r\n File "<doctest libpysal.cg.shapely_ext.buffer[6]>", line 1, in <module>\r\n g.buffer(1.0, cap_style=\'square\').area\r\n File "C:\\Users\\200059719\\AppData\\Local\\conda\\conda\\envs\\test_momepy\\lib\\site-packages\\shapely\\geometry\\base.py", line 593, in buffer\r\n mitre_limit))\r\n File "C:\\Users\\200059719\\AppData\\Local\\conda\\conda\\envs\\test_momepy\\lib\\site-packages\\shapely\\topology.py", line 78, in __call__\r\n return self.fn(this._geom, *args)\r\n ctypes.ArgumentError: argument 5: <class \'TypeError\'>: wrong type\r\n\r\n\r\n======================================================================\r\nFAIL: Doctest: libpysal.cg.sphere.geogrid\r\n----------------------------------------------------------------------\r\nTraceback (most recent call last):\r\n File "C:\\Users\\200059719\\AppData\\Local\\conda\\conda\\envs\\test_momepy\\lib\\doctest.py", line 2196, in runTest\r\n raise self.failureException(self.format_failure(new.getvalue()))\r\nAssertionError: Failed doctest test for libpysal.cg.sphere.geogrid\r\n File "C:\\Users\\200059719\\libpysal\\libpysal\\cg\\sphere.py", line 438, in geogrid\r\n\r\n----------------------------------------------------------------------\r\nFile "C:\\Users\\200059719\\libpysal\\libpysal\\cg\\sphere.py", line 461, in libpysal.cg.sphere.geogrid\r\nFailed example:\r\n geogrid(pup,pdown,3,lonx=False)\r\nExpected:\r\n [(42.023768, -87.946389), (42.02393997819538, -87.80562679358316), (42.02393997819538, -87.66486420641684), (42.023768, -87.524102), (41.897317, -87.94638900000001), (41.8974888973743, -87.80562679296166), (41.8974888973743, -87.66486420703835), (41.897317, -87.524102), (41.770866000000005, -87.94638900000001), (41.77103781320412, -87.80562679234043), (41.77103781320412, -87.66486420765956), (41.770866000000005, -87.524102), (41.644415, -87.946389), (41.64458672568646, -87.80562679171955), (41.64458672568646, -87.66486420828045), (41.644415, -87.524102)]\r\nGot:\r\n [(42.023768, -87.946389), (42.02393997819538, -87.80562679358316), (42.02393997819538, -87.66486420641684), (42.023768, -87.524102), (41.897317, -87.94638900000001), (41.8974888973743, -87.80562679296166), (41.8974888973743, -87.66486420703835), (41.897317, -87.524102), (41.770866000000005, -87.94638900000001), (41.77103781320412, -87.80562679234043), (41.77103781320412, -87.66486420765956), (41.770866000000005, -87.524102), (41.644415, -87.946389), (41.64458672568647, -87.80562679171955), (41.64458672568647, -87.66486420828045), (41.644415, -87.524102)]\r\n\r\n\r\n======================================================================\r\nFAIL: Doctest: libpysal.io.iohandlers.csvWrapper.csvWrapper.__init__\r\n----------------------------------------------------------------------\r\nTraceback (most recent call last):\r\n File "C:\\Users\\200059719\\AppData\\Local\\conda\\conda\\envs\\test_momepy\\lib\\doctest.py", line 2196, in runTest\r\n raise self.failureException(self.format_failure(new.getvalue()))\r\nAssertionError: Failed doctest test for libpysal.io.iohandlers.csvWrapper.csvWrapper.__init__\r\n File "C:\\Users\\200059719\\libpysal\\libpysal\\io\\iohandlers\\csvWrapper.py", line 15, in __init__\r\n\r\n----------------------------------------------------------------------\r\nFile "C:\\Users\\200059719\\libpysal\\libpysal\\io\\iohandlers\\csvWrapper.py", line 24, in libpysal.io.iohandlers.csvWrapper.csvWrapper.__init__\r\nFailed example:\r\n f.header\r\nExpected:\r\n [\'WKT\', \'NAME\', \'STATE_NAME\', \'STATE_FIPS\', \'CNTY_FIPS\', \'FIPS\', \'FIPSNO\', \'HR7984\', \'HR8488\', \'HR8893\', \'HC7984\', \'HC8488\', \'HC8893\', \'PO7984\', \'PO8488\', \'PO8893\', \'PE77\', \'PE82\', \'PE87\', \'RDAC80\', \'RDAC85\', \'RDAC90\']\r\nGot:\r\n [\'FIPSNO\', \'NAME\', \'STATE_NAME\', \'STATE_FIPS\', \'CNTY_FIPS\', \'FIPS\', \'HC7984\', \'HC8488\', \'HC8893\', \'PO7984\', \'PO8488\', \'PO8893\']\r\n----------------------------------------------------------------------\r\nFile "C:\\Users\\200059719\\libpysal\\libpysal\\io\\iohandlers\\csvWrapper.py", line 26, in libpysal.io.iohandlers.csvWrapper.csvWrapper.__init__\r\nFailed example:\r\n f._spec\r\nExpected:\r\n [<class \'str\'>, <class \'str\'>, <class \'str\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'float\'>, <class \'float\'>, <class \'float\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'float\'>, <class \'float\'>, <class \'float\'>, <class \'float\'>, <class \'float\'>, <class \'float\'>]\r\nGot:\r\n [<class \'int\'>, <class \'str\'>, <class \'str\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>]\r\n\r\n\r\nName Stmts Miss Cover\r\n-------------------------------------------------------------\r\nlibpysal\\__init__.py 5 0 100%\r\nlibpysal\\cg\\__init__.py 8 0 100%\r\nlibpysal\\cg\\alpha_shapes.py 142 55 61%\r\nlibpysal\\cg\\kdtree.py 84 11 87%\r\nlibpysal\\cg\\locators.py 218 12 94%\r\nlibpysal\\cg\\ops\\__init__.py 2 0 100%\r\nlibpysal\\cg\\ops\\_accessors.py 15 3 80%\r\nlibpysal\\cg\\ops\\_shapely.py 59 32 46%\r\nlibpysal\\cg\\ops\\atomic.py 7 0 100%\r\nlibpysal\\cg\\ops\\tabular.py 47 21 55%\r\nlibpysal\\cg\\polygonQuadTreeStructure.py 553 80 86%\r\nlibpysal\\cg\\rtree.py 416 35 92%\r\nlibpysal\\cg\\segmentLocator.py 258 131 49%\r\nlibpysal\\cg\\shapely_ext.py 209 159 24%\r\nlibpysal\\cg\\shapes.py 473 46 90%\r\nlibpysal\\cg\\sphere.py 154 42 73%\r\nlibpysal\\cg\\standalone.py 310 19 94%\r\nlibpysal\\cg\\voronoi.py 98 21 79%\r\nlibpysal\\common.py 51 19 63%\r\nlibpysal\\examples\\__init__.py 21 2 90%\r\nlibpysal\\examples\\base.py 145 68 53%\r\nlibpysal\\examples\\builtin.py 39 4 90%\r\nlibpysal\\examples\\remotes.py 65 9 86%\r\nlibpysal\\io\\__init__.py 5 0 100%\r\nlibpysal\\io\\fileio.py 210 75 64%\r\nlibpysal\\io\\geotable\\__init__.py 1 0 100%\r\nlibpysal\\io\\geotable\\dbf.py 46 30 35%\r\nlibpysal\\io\\geotable\\file.py 42 24 43%\r\nlibpysal\\io\\geotable\\shp.py 13 5 62%\r\nlibpysal\\io\\geotable\\utils.py 30 12 60%\r\nlibpysal\\io\\geotable\\wrappers.py 30 19 37%\r\nlibpysal\\io\\iohandlers\\__init__.py 23 2 91%\r\nlibpysal\\io\\iohandlers\\arcgis_dbf.py 78 6 92%\r\nlibpysal\\io\\iohandlers\\arcgis_swm.py 110 4 96%\r\nlibpysal\\io\\iohandlers\\arcgis_txt.py 60 14 77%\r\nlibpysal\\io\\iohandlers\\csvWrapper.py 61 5 92%\r\nlibpysal\\io\\iohandlers\\dat.py 19 1 95%\r\nlibpysal\\io\\iohandlers\\db.py 58 9 84%\r\nlibpysal\\io\\iohandlers\\gal.py 99 5 95%\r\nlibpysal\\io\\iohandlers\\geobugs_txt.py 84 1 99%\r\nlibpysal\\io\\iohandlers\\geoda_txt.py 59 11 81%\r\nlibpysal\\io\\iohandlers\\gwt.py 137 25 82%\r\nlibpysal\\io\\iohandlers\\mat.py 47 6 87%\r\nlibpysal\\io\\iohandlers\\mtx.py 40 1 98%\r\nlibpysal\\io\\iohandlers\\pyDbfIO.py 237 49 79%\r\nlibpysal\\io\\iohandlers\\pyShpIO.py 116 28 76%\r\nlibpysal\\io\\iohandlers\\stata_txt.py 74 1 99%\r\nlibpysal\\io\\iohandlers\\template.py 77 57 26%\r\nlibpysal\\io\\iohandlers\\wk1.py 91 8 91%\r\nlibpysal\\io\\iohandlers\\wkt.py 46 8 83%\r\nlibpysal\\io\\tables.py 89 16 82%\r\nlibpysal\\io\\util\\__init__.py 3 0 100%\r\nlibpysal\\io\\util\\shapefile.py 380 18 95%\r\nlibpysal\\io\\util\\weight_converter.py 48 13 73%\r\nlibpysal\\io\\util\\wkb.py 78 66 15%\r\nlibpysal\\io\\util\\wkt.py 42 5 88%\r\nlibpysal\\weights\\__init__.py 8 0 100%\r\nlibpysal\\weights\\_contW_lists.py 77 6 92%\r\nlibpysal\\weights\\adjtools.py 89 15 83%\r\nlibpysal\\weights\\contiguity.py 146 34 77%\r\nlibpysal\\weights\\distance.py 250 36 86%\r\nlibpysal\\weights\\set_operations.py 103 34 67%\r\nlibpysal\\weights\\spatial_lag.py 43 3 93%\r\nlibpysal\\weights\\spintW.py 67 6 91%\r\nlibpysal\\weights\\user.py 44 7 84%\r\nlibpysal\\weights\\util.py 465 58 88%\r\nlibpysal\\weights\\weights.py 510 87 83%\r\n-------------------------------------------------------------\r\nTOTAL 7714 1579 80%\r\n----------------------------------------------------------------------\r\nRan 728 tests in 178.610s\r\n\r\nFAILED (SKIP=41, errors=4, failures=3)\r\n```\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n```\r\n======================================================================\r\nERROR: test_casting (libpysal.io.iohandlers.tests.test_csvWrapper.test_csvWrapper)\r\n----------------------------------------------------------------------\r\nTraceback (most recent call last):\r\n File "/Users/user/libpysal/libpysal/io/iohandlers/tests/test_csvWrapper.py", line 47, in test_casting\r\n self.obj.cast(\'WKT\', WKTParser())\r\n File "/Users/user/libpysal/libpysal/io/fileio.py", line 230, in cast\r\n raise KeyError("%s" % key)\r\nKeyError: \'WKT\'\r\n\r\n======================================================================\r\nERROR: test_true_rook2 (libpysal.weights.tests.test__contW_lists.TestContiguityWeights)\r\n----------------------------------------------------------------------\r\nTraceback (most recent call last):\r\n File "/Users/user/libpysal/libpysal/weights/tests/test__contW_lists.py", line 75, in test_true_rook2\r\n \'stl_hom.shp\'), ROOK, \'POLY_ID_OG\')\r\n File "/Users/user/libpysal/libpysal/weights/tests/test__contW_lists.py", line 120, in build_W\r\n ids = db.by_col[idVariable]\r\n File "/Users/user/libpysal/libpysal/io/tables.py", line 21, in __getitem__\r\n return self.p._get_col(key)\r\n File "/Users/user/libpysal/libpysal/io/iohandlers/pyDbfIO.py", line 110, in _get_col\r\n raise AttributeError(\'Field: % s does not exist in header\' % key)\r\nAttributeError: Field: POLY_ID_OG does not exist in header\r\n\r\n======================================================================\r\nFAIL: Doctest: libpysal.io.iohandlers.csvWrapper.csvWrapper.__init__\r\n----------------------------------------------------------------------\r\nTraceback (most recent call last):\r\n File "/Users/user/miniconda3/envs/py3_lps_dev/lib/python3.6/doctest.py", line 2199, in runTest\r\n raise self.failureException(self.format_failure(new.getvalue()))\r\nAssertionError: Failed doctest test for libpysal.io.iohandlers.csvWrapper.csvWrapper.__init__\r\n File "/Users/user/libpysal/libpysal/io/iohandlers/csvWrapper.py", line 15, in __init__\r\n\r\n----------------------------------------------------------------------\r\nFile "/Users/user/libpysal/libpysal/io/iohandlers/csvWrapper.py", line 24, in libpysal.io.iohandlers.csvWrapper.csvWrapper.__init__\r\nFailed example:\r\n f.header\r\nExpected:\r\n [\'WKT\', \'NAME\', \'STATE_NAME\', \'STATE_FIPS\', \'CNTY_FIPS\', \'FIPS\', \'FIPSNO\', \'HR7984\', \'HR8488\', \'HR8893\', \'HC7984\', \'HC8488\', \'HC8893\', \'PO7984\', \'PO8488\', \'PO8893\', \'PE77\', \'PE82\', \'PE87\', \'RDAC80\', \'RDAC85\', \'RDAC90\']\r\nGot:\r\n [\'FIPSNO\', \'NAME\', \'STATE_NAME\', \'STATE_FIPS\', \'CNTY_FIPS\', \'FIPS\', \'HC7984\', \'HC8488\', \'HC8893\', \'PO7984\', \'PO8488\', \'PO8893\']\r\n----------------------------------------------------------------------\r\nFile "/Users/user/libpysal/libpysal/io/iohandlers/csvWrapper.py", line 26, in libpysal.io.iohandlers.csvWrapper.csvWrapper.__init__\r\nFailed example:\r\n f._spec\r\nExpected:\r\n [<class \'str\'>, <class \'str\'>, <class \'str\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'float\'>, <class \'float\'>, <class \'float\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'float\'>, <class \'float\'>, <class \'float\'>, <class \'float\'>, <class \'float\'>, <class \'float\'>]\r\nGot:\r\n [<class \'int\'>, <class \'str\'>, <class \'str\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>, <class \'int\'>]\r\n\r\n\r\nName Stmts Miss Cover\r\n-------------------------------------------------------------\r\nlibpysal/__init__.py 5 0 100%\r\nlibpysal/cg/__init__.py 8 0 100%\r\nlibpysal/cg/alpha_shapes.py 142 46 68%\r\nlibpysal/cg/kdtree.py 84 11 87%\r\nlibpysal/cg/locators.py 218 12 94%\r\nlibpysal/cg/ops/__init__.py 2 0 100%\r\nlibpysal/cg/ops/_accessors.py 15 3 80%\r\nlibpysal/cg/ops/_shapely.py 59 32 46%\r\nlibpysal/cg/ops/atomic.py 7 0 100%\r\nlibpysal/cg/ops/tabular.py 47 21 55%\r\nlibpysal/cg/polygonQuadTreeStructure.py 553 80 86%\r\nlibpysal/cg/rtree.py 416 35 92%\r\nlibpysal/cg/segmentLocator.py 258 131 49%\r\nlibpysal/cg/shapely_ext.py 209 159 24%\r\nlibpysal/cg/shapes.py 473 46 90%\r\nlibpysal/cg/sphere.py 154 34 78%\r\nlibpysal/cg/standalone.py 310 18 94%\r\nlibpysal/cg/voronoi.py 98 16 84%\r\nlibpysal/common.py 51 4 92%\r\nlibpysal/examples/__init__.py 21 1 95%\r\nlibpysal/examples/base.py 145 58 60%\r\nlibpysal/examples/builtin.py 39 4 90%\r\nlibpysal/examples/remotes.py 65 9 86%\r\nlibpysal/io/__init__.py 5 0 100%\r\nlibpysal/io/fileio.py 210 75 64%\r\nlibpysal/io/geotable/__init__.py 1 0 100%\r\nlibpysal/io/geotable/dbf.py 46 30 35%\r\nlibpysal/io/geotable/file.py 42 24 43%\r\nlibpysal/io/geotable/shp.py 13 5 62%\r\nlibpysal/io/geotable/utils.py 30 12 60%\r\nlibpysal/io/geotable/wrappers.py 30 19 37%\r\nlibpysal/io/iohandlers/__init__.py 23 2 91%\r\nlibpysal/io/iohandlers/arcgis_dbf.py 78 6 92%\r\nlibpysal/io/iohandlers/arcgis_swm.py 110 4 96%\r\nlibpysal/io/iohandlers/arcgis_txt.py 60 14 77%\r\nlibpysal/io/iohandlers/csvWrapper.py 61 0 100%\r\nlibpysal/io/iohandlers/dat.py 19 1 95%\r\nlibpysal/io/iohandlers/db.py 58 9 84%\r\nlibpysal/io/iohandlers/gal.py 99 5 95%\r\nlibpysal/io/iohandlers/geobugs_txt.py 84 1 99%\r\nlibpysal/io/iohandlers/geoda_txt.py 59 11 81%\r\nlibpysal/io/iohandlers/gwt.py 137 25 82%\r\nlibpysal/io/iohandlers/mat.py 47 6 87%\r\nlibpysal/io/iohandlers/mtx.py 40 1 98%\r\nlibpysal/io/iohandlers/pyDbfIO.py 237 49 79%\r\nlibpysal/io/iohandlers/pyShpIO.py 116 28 76%\r\nlibpysal/io/iohandlers/stata_txt.py 74 1 99%\r\nlibpysal/io/iohandlers/template.py 77 57 26%\r\nlibpysal/io/iohandlers/wk1.py 91 8 91%\r\nlibpysal/io/iohandlers/wkt.py 46 8 83%\r\nlibpysal/io/tables.py 89 16 82%\r\nlibpysal/io/util/__init__.py 3 0 100%\r\nlibpysal/io/util/shapefile.py 380 18 95%\r\nlibpysal/io/util/weight_converter.py 48 13 73%\r\nlibpysal/io/util/wkb.py 78 66 15%\r\nlibpysal/io/util/wkt.py 42 5 88%\r\nlibpysal/weights/__init__.py 8 0 100%\r\nlibpysal/weights/_contW_lists.py 77 6 92%\r\nlibpysal/weights/adjtools.py 89 14 84%\r\nlibpysal/weights/contiguity.py 146 21 86%\r\nlibpysal/weights/distance.py 250 18 93%\r\nlibpysal/weights/set_operations.py 103 23 78%\r\nlibpysal/weights/spatial_lag.py 43 3 93%\r\nlibpysal/weights/spintW.py 67 5 93%\r\nlibpysal/weights/user.py 44 7 84%\r\nlibpysal/weights/util.py 465 40 91%\r\nlibpysal/weights/weights.py 510 43 92%\r\n-------------------------------------------------------------\r\nTOTAL 7714 1419 82%\r\n----------------------------------------------------------------------\r\nRan 727 tests in 68.534s\r\n\r\nFAILED (SKIP=41, errors=2, failures=1)\r\n```\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/212',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/212/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/212/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/212/events',
'html_url': 'https://github.com/pysal/libpysal/issues/212',
'id': 538770111,
'node_id': 'MDU6SXNzdWU1Mzg3NzAxMTE=',
'number': 212,
'title': 'Constructing contiguity spatial weights using from_dataframe and from_shapefile could give different results',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 2,
'created_at': '2019-12-17T00:55:34Z',
'updated_at': '2020-01-04T15:23:11Z',
'closed_at': '2020-01-04T15:23:11Z',
'author_association': 'MEMBER',
'body': 'Constructing contiguity spatial weights using `from_dataframe` and `from_shapefile` could give different results. An example notebook with the Baltimore example data set is given [here](https://github.com/weikang9009/libpysal/blob/weight/notebooks/weight_issue.ipynb).\r\n\r\nBased on the Baltimore example data set, constructing a Queen or Rook contiguity weight directly from the shapefile will give a (210,210) weight matrix (with `from_shapefile`), while constructing that from the GeoDataFrame will produce a (211,211) weight matrix (with `from_shapefile`).\r\n\r\nIn contrast, KNN constructor gives identical spatial weight matrices (both are (211,211)).\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/213',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/213/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/213/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/213/events',
'html_url': 'https://github.com/pysal/libpysal/pull/213',
'id': 542687836,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU3MDgxMTQ2',
'number': 213,
'title': 'fix bug 212',
'user': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-26T22:48:38Z',
'updated_at': '2020-01-04T15:22:21Z',
'closed_at': '2020-01-04T15:22:21Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/213',
'html_url': 'https://github.com/pysal/libpysal/pull/213',
'diff_url': 'https://github.com/pysal/libpysal/pull/213.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/213.patch'},
'body': "Sorry I didn't see #212 earlier. This fixes the described bug by ensuring that the `try/except` block of `libpysal.weights.util.get_points_array()` is safe for lazy input. Before, the [linked explanation succinctly describes the source of the bug.](https://github.com/pysal/libpysal/issues/212#issuecomment-569144720)."},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/216',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/216/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/216/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/216/events',
'html_url': 'https://github.com/pysal/libpysal/issues/216',
'id': 543797009,
'node_id': 'MDU6SXNzdWU1NDM3OTcwMDk=',
'number': 216,
'title': 'alpha_shapes docs not rendering',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."},
{'id': 1685673930,
'node_id': 'MDU6TGFiZWwxNjg1NjczOTMw',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/cg',
'name': 'cg',
'color': '4363cc',
'default': False,
'description': ''},
{'id': 1376367312,
'node_id': 'MDU6TGFiZWwxMzc2MzY3MzEy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/docs',
'name': 'docs',
'color': 'ffc8b2',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 0,
'created_at': '2019-12-30T08:41:22Z',
'updated_at': '2019-12-30T19:33:17Z',
'closed_at': '2019-12-30T19:33:17Z',
'author_association': 'MEMBER',
'body': 'Currently the [`alpha_shapes` docs](https://pysal.org/libpysal/generated/libpysal.cg.alpha_shape_auto.html#libpysal.cg.alpha_shape_auto) are not rendering properly due to incorrect docstring headings being used (`Arguments` and `Example` vs. `Parameters` and `Examples`).'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/217',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/217/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/217/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/217/events',
'html_url': 'https://github.com/pysal/libpysal/pull/217',
'id': 543797614,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4MDQ0MDQz',
'number': 217,
'title': 'corrected docstrings in cg.alpha_shapes.py',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."},
{'id': 1685673930,
'node_id': 'MDU6TGFiZWwxNjg1NjczOTMw',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/cg',
'name': 'cg',
'color': '4363cc',
'default': False,
'description': ''},
{'id': 1376367312,
'node_id': 'MDU6TGFiZWwxMzc2MzY3MzEy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/docs',
'name': 'docs',
'color': 'ffc8b2',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2019-12-30T08:43:34Z',
'updated_at': '2019-12-30T19:32:06Z',
'closed_at': '2019-12-30T19:32:02Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/217',
'html_url': 'https://github.com/pysal/libpysal/pull/217',
'diff_url': 'https://github.com/pysal/libpysal/pull/217.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/217.patch'},
'body': 'This PR addresses pysal/libpysal#216 and beefs up/corrects the docstrings in `cg.alpha_shapes.py`'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/211',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/211/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/211/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/211/events',
'html_url': 'https://github.com/pysal/libpysal/pull/211',
'id': 537956315,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUzMjA2MTMx',
'number': 211,
'title': 'Updating requirements',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-14T19:42:20Z',
'updated_at': '2019-12-14T19:47:49Z',
'closed_at': '2019-12-14T19:47:49Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/211',
'html_url': 'https://github.com/pysal/libpysal/pull/211',
'diff_url': 'https://github.com/pysal/libpysal/pull/211.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/211.patch'},
'body': '- handle explain when called in terminal shell\r\n- update docs'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/174',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/174/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/174/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/174/events',
'html_url': 'https://github.com/pysal/libpysal/issues/174',
'id': 479428542,
'node_id': 'MDU6SXNzdWU0Nzk0Mjg1NDI=',
'number': 174,
'title': 'Big tarball',
'user': {'login': 'ocefpaf',
'id': 950575,
'node_id': 'MDQ6VXNlcjk1MDU3NQ==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/950575?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ocefpaf',
'html_url': 'https://github.com/ocefpaf',
'followers_url': 'https://api.github.com/users/ocefpaf/followers',
'following_url': 'https://api.github.com/users/ocefpaf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ocefpaf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ocefpaf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ocefpaf/subscriptions',
'organizations_url': 'https://api.github.com/users/ocefpaf/orgs',
'repos_url': 'https://api.github.com/users/ocefpaf/repos',
'events_url': 'https://api.github.com/users/ocefpaf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ocefpaf/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1032340595,
'node_id': 'MDU6TGFiZWwxMDMyMzQwNTk1',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/WIP',
'name': 'WIP',
'color': '9b8cf7',
'default': False,
'description': 'Work in progress, do not merge. Discussion only.'},
{'id': 1685679755,
'node_id': 'MDU6TGFiZWwxNjg1Njc5NzU1',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/examples',
'name': 'examples',
'color': '135699',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 3,
'created_at': '2019-07-31T20:09:14Z',
'updated_at': '2019-12-14T19:14:51Z',
'closed_at': '2019-12-14T19:14:51Z',
'author_association': 'NONE',
'body': 'The PyPI tarball is ~25 MB, most of is the examples folder.\r\nIf the original design is to ship that folder with the tarball please close the issue, if not I can send a PR excluding that folder from the MANIFEST to produce a smaller tarball.'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/176',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/176/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/176/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/176/events',
'html_url': 'https://github.com/pysal/libpysal/pull/176',
'id': 484852713,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwNjM0Mjg0',
'number': 176,
'title': 'Fetch',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186559,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTk=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 5,
'created_at': '2019-08-24T19:04:06Z',
'updated_at': '2019-12-14T18:32:16Z',
'closed_at': '2019-12-14T18:32:16Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/176',
'html_url': 'https://github.com/pysal/libpysal/pull/176',
'diff_url': 'https://github.com/pysal/libpysal/pull/176.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/176.patch'},
'body': 'This would remove some of the larger example datasets out of the source distribution and provide for remote fetching of those examples when needed.\r\n\r\nIt is a start towards addressing #174.\r\n\r\nThis would have implications for any packages that rely on these larger datasets for integration testing. An example of how this could be done is [here](https://github.com/pysal/libpysal/commit/cfd200d0447914b369c16694e218f485d43ad037 ).\r\n\r\nThis has started with the nat and south datasets.\r\n\r\nThere is some refinement that needs to be done, but I wanted to get feedback on the general direction.\r\n\r\n\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/125',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/125/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/125/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/125/events',
'html_url': 'https://github.com/pysal/libpysal/pull/125',
'id': 376626480,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MjI3ODAzMTE3',
'number': 125,
'title': 'metadata for examples ',
'user': {'login': 'TaylorOshan',
'id': 8117709,
'node_id': 'MDQ6VXNlcjgxMTc3MDk=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/8117709?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/TaylorOshan',
'html_url': 'https://github.com/TaylorOshan',
'followers_url': 'https://api.github.com/users/TaylorOshan/followers',
'following_url': 'https://api.github.com/users/TaylorOshan/following{/other_user}',
'gists_url': 'https://api.github.com/users/TaylorOshan/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/TaylorOshan/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/TaylorOshan/subscriptions',
'organizations_url': 'https://api.github.com/users/TaylorOshan/orgs',
'repos_url': 'https://api.github.com/users/TaylorOshan/repos',
'events_url': 'https://api.github.com/users/TaylorOshan/events{/privacy}',
'received_events_url': 'https://api.github.com/users/TaylorOshan/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 2,
'created_at': '2018-11-02T00:58:12Z',
'updated_at': '2019-12-07T18:31:50Z',
'closed_at': '2019-12-07T18:31:49Z',
'author_association': 'CONTRIBUTOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/125',
'html_url': 'https://github.com/pysal/libpysal/pull/125',
'diff_url': 'https://github.com/pysal/libpysal/pull/125.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/125.patch'},
'body': 'The justification for this PR is to satisfy #113 by adding metadata for the georgia, tokyo, clearwater, nyc_bikes, and prenzlauer datasets in the style of the metadata provided for the mexico dataset.\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/209',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/209/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/209/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/209/events',
'html_url': 'https://github.com/pysal/libpysal/pull/209',
'id': 533113728,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ5MjQ1NzE3',
'number': 209,
'title': 'DOC: math rendering in sphinx, and members included for W',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 2,
'created_at': '2019-12-05T04:18:18Z',
'updated_at': '2019-12-12T11:41:39Z',
'closed_at': '2019-12-07T18:21:21Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/209',
'html_url': 'https://github.com/pysal/libpysal/pull/209',
'diff_url': 'https://github.com/pysal/libpysal/pull/209.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/209.patch'},
'body': '@weikang9009 see the use of the `members` option in the [W rst file](https://github.com/pysal/libpysal/compare/master...sjsrey:math?expand=1#diff-b4c964f775631bd479f5aebd399659b9).'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/210',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/210/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/210/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/210/events',
'html_url': 'https://github.com/pysal/libpysal/pull/210',
'id': 533163857,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ5Mjg2OTQ4',
'number': 210,
'title': '(docs) automatically generate docstrings for class members',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1376367312,
'node_id': 'MDU6TGFiZWwxMzc2MzY3MzEy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/docs',
'name': 'docs',
'color': 'ffc8b2',
'default': False,
'description': ''},
{'id': 536186559,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTk=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 2,
'created_at': '2019-12-05T07:00:45Z',
'updated_at': '2019-12-07T18:20:23Z',
'closed_at': '2019-12-07T18:20:23Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/210',
'html_url': 'https://github.com/pysal/libpysal/pull/210',
'diff_url': 'https://github.com/pysal/libpysal/pull/210.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/210.patch'},
'body': 'This PR is to update the configuration file for sphinx to:\r\n* automatically generate docstrings for class members including methods, attributes, properties\r\n* avoid display class members twice \r\n\r\nthis would be a more convenient fix compared with the solution suggested in https://github.com/pysal/libpysal/pull/209'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/207',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/207/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/207/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/207/events',
'html_url': 'https://github.com/pysal/libpysal/pull/207',
'id': 527773641,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ0OTYxMDYy',
'number': 207,
'title': '(docs) keep file .nojekyll in docs when syncing between docs/ and docsrc/_build/html/',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-11-24T23:09:44Z',
'updated_at': '2019-12-05T00:25:43Z',
'closed_at': '2019-12-05T00:25:43Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/207',
'html_url': 'https://github.com/pysal/libpysal/pull/207',
'diff_url': 'https://github.com/pysal/libpysal/pull/207.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/207.patch'},
'body': 'Currently, we update the contents of [docs/](https://github.com/pysal/libpysal/tree/ed098ea900bde6a9913b96e162d64a72a9615e11/docs) with the built html files in docsrc/_build/html/ (after running `make html`). The existing files in docs/ which do not match those from docsrc/_build/html/ are deleted including .nojekyll \r\n\r\nThis PR is to adjust the makefile to keep .nojekyll in docs/ when syncing between docs/ and docsrc/_build/html/\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/206',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/206/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/206/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/206/events',
'html_url': 'https://github.com/pysal/libpysal/pull/206',
'id': 527754264,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ0OTQ2OTQ2',
'number': 206,
'title': '(bug) replace silent_island_warning with silence_warnings for weights',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 2,
'created_at': '2019-11-24T20:47:59Z',
'updated_at': '2019-11-24T22:34:37Z',
'closed_at': '2019-11-24T22:34:36Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/206',
'html_url': 'https://github.com/pysal/libpysal/pull/206',
'diff_url': 'https://github.com/pysal/libpysal/pull/206.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/206.patch'},
'body': '`silent_island_warning` was removed from the attribute list of `libpysal.weights.W` and was replaced with the name `silence_warnings`, but one occurence was not updated to reflect such change, leading to errors reported in https://github.com/pysal/libpysal/issues/204 and https://github.com/pysal/spreg/pull/27\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/205',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/205/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/205/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/205/events',
'html_url': 'https://github.com/pysal/libpysal/issues/205',
'id': 520516235,
'node_id': 'MDU6SXNzdWU1MjA1MTYyMzU=',
'number': 205,
'title': 'Documentation does not work',
'user': {'login': 'martinfleis',
'id': 36797143,
'node_id': 'MDQ6VXNlcjM2Nzk3MTQz',
'avatar_url': 'https://avatars2.githubusercontent.com/u/36797143?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/martinfleis',
'html_url': 'https://github.com/martinfleis',
'followers_url': 'https://api.github.com/users/martinfleis/followers',
'following_url': 'https://api.github.com/users/martinfleis/following{/other_user}',
'gists_url': 'https://api.github.com/users/martinfleis/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/martinfleis/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/martinfleis/subscriptions',
'organizations_url': 'https://api.github.com/users/martinfleis/orgs',
'repos_url': 'https://api.github.com/users/martinfleis/repos',
'events_url': 'https://api.github.com/users/martinfleis/events{/privacy}',
'received_events_url': 'https://api.github.com/users/martinfleis/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."},
{'id': 1376367312,
'node_id': 'MDU6TGFiZWwxMzc2MzY3MzEy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/docs',
'name': 'docs',
'color': 'ffc8b2',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2019-11-09T21:27:32Z',
'updated_at': '2019-11-10T00:07:27Z',
'closed_at': '2019-11-10T00:07:27Z',
'author_association': 'CONTRIBUTOR',
'body': 'Hi,\r\n\r\nhttps://libpysal.readthedocs.io/en/latest/ does not seem to work (for some time already). Is it intentional or not? Pysal.org still links to readthedocs, so it seems to be an issue? Or do you want to keep it within API of pysal meta-package only?'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/203',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/203/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/203/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/203/events',
'html_url': 'https://github.com/pysal/libpysal/pull/203',
'id': 510004258,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzMwNDQwMDM3',
'number': 203,
'title': 'updating cg.standalone.distance_matrix docs',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1376367312,
'node_id': 'MDU6TGFiZWwxMzc2MzY3MzEy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/docs',
'name': 'docs',
'color': 'ffc8b2',
'default': False,
'description': ''},
{'id': 1280158628,
'node_id': 'MDU6TGFiZWwxMjgwMTU4NjI4',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/maintenance',
'name': 'maintenance',
'color': 'c2e0c6',
'default': False,
'description': ''},
{'id': 1000808619,
'node_id': 'MDU6TGFiZWwxMDAwODA4NjE5',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/rough%20edge',
'name': 'rough edge',
'color': '5621dd',
'default': False,
'description': 'Something that\'s not a bug, but that gets in the way of "it just works."'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 0,
'created_at': '2019-10-21T14:34:20Z',
'updated_at': '2019-10-21T17:53:47Z',
'closed_at': '2019-10-21T17:53:44Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/203',
'html_url': 'https://github.com/pysal/libpysal/pull/203',
'diff_url': 'https://github.com/pysal/libpysal/pull/203.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/203.patch'},
'body': 'The justification for this PR is: \r\n\r\n * Fixes #195 \r\n * corrects grammar and spelling in docstring of `cg.standalone.distance_matrix`'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/195',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/195/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/195/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/195/events',
'html_url': 'https://github.com/pysal/libpysal/issues/195',
'id': 507392440,
'node_id': 'MDU6SXNzdWU1MDczOTI0NDA=',
'number': 195,
'title': 'error message in cg.standalone.distance_matrix()',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1280158628,
'node_id': 'MDU6TGFiZWwxMjgwMTU4NjI4',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/maintenance',
'name': 'maintenance',
'color': 'c2e0c6',
'default': False,
'description': ''},
{'id': 1000808619,
'node_id': 'MDU6TGFiZWwxMDAwODA4NjE5',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/rough%20edge',
'name': 'rough edge',
'color': '5621dd',
'default': False,
'description': 'Something that\'s not a bug, but that gets in the way of "it just works."'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 0,
'created_at': '2019-10-15T17:51:20Z',
'updated_at': '2019-10-21T17:53:44Z',
'closed_at': '2019-10-21T17:53:44Z',
'author_association': 'MEMBER',
'body': 'We could use a more informative error message in [`line 904`](https://github.com/pysal/libpysal/blob/574192fca6fc2640f05d9617a80cb2637e00f93b/libpysal/cg/standalone.py#L904) of [`cg.standalone. distance_matrix()`](https://github.com/pysal/libpysal/blob/574192fca6fc2640f05d9617a80cb2637e00f93b/libpysal/cg/standalone.py#L856-L921).'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/202',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/202/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/202/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/202/events',
'html_url': 'https://github.com/pysal/libpysal/pull/202',
'id': 509627999,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzMwMTQwMjAw',
'number': 202,
'title': 'improved docs in io.util.shapefile',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1376367312,
'node_id': 'MDU6TGFiZWwxMzc2MzY3MzEy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/docs',
'name': 'docs',
'color': 'ffc8b2',
'default': False,
'description': ''},
{'id': 1280158628,
'node_id': 'MDU6TGFiZWwxMjgwMTU4NjI4',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/maintenance',
'name': 'maintenance',
'color': 'c2e0c6',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 0,
'created_at': '2019-10-20T17:54:38Z',
'updated_at': '2019-10-20T20:48:49Z',
'closed_at': '2019-10-20T20:47:34Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/202',
'html_url': 'https://github.com/pysal/libpysal/pull/202',
'diff_url': 'https://github.com/pysal/libpysal/pull/202.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/202.patch'},
'body': 'This PR provides minor documentation improvements in [`io.util.shapefile`](https://github.com/pysal/libpysal/blob/master/libpysal/io/util/shapefile.py) for:\r\n* spelling\r\n* capitalization\r\n* grammar '},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/201',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/201/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/201/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/201/events',
'html_url': 'https://github.com/pysal/libpysal/pull/201',
'id': 509501848,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzMwMDUyMjQ4',
'number': 201,
'title': '[ENH] moving jit import to common.py / improve documentation',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186559,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTk=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1280158628,
'node_id': 'MDU6TGFiZWwxMjgwMTU4NjI4',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/maintenance',
'name': 'maintenance',
'color': 'c2e0c6',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 0,
'created_at': '2019-10-19T19:53:00Z',
'updated_at': '2019-10-20T20:44:47Z',
'closed_at': '2019-10-20T20:37:38Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/201',
'html_url': 'https://github.com/pysal/libpysal/pull/201',
'diff_url': 'https://github.com/pysal/libpysal/pull/201.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/201.patch'},
'body': 'This PR moves the import of [`numba.jit`](https://github.com/pysal/libpysal/blob/8c2627f258b5a2ba377f14e80985d8cbcf2b50f1/libpysal/cg/alpha_shapes.py#L11-L25) from `cg.alpha_shapes.py` into `common.py`, which also includes the `HAS_JIT` flag. By switching to this import schema functions within `libpysal` or a federated submodule can benefit from the potential performance improvements (if `numba` is installed).'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/199',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/199/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/199/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/199/events',
'html_url': 'https://github.com/pysal/libpysal/issues/199',
'id': 508474748,
'node_id': 'MDU6SXNzdWU1MDg0NzQ3NDg=',
'number': 199,
'title': 'rearrange shapely import in cg.alpha_shapes',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1000808619,
'node_id': 'MDU6TGFiZWwxMDAwODA4NjE5',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/rough%20edge',
'name': 'rough edge',
'color': '5621dd',
'default': False,
'description': 'Something that\'s not a bug, but that gets in the way of "it just works."'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2019-10-17T13:26:43Z',
'updated_at': '2019-10-17T15:26:43Z',
'closed_at': '2019-10-17T15:26:43Z',
'author_association': 'MEMBER',
'body': "Following my false alarm yesterday (#197, #198), I was wondering if the `shapely` import statement from [`cg.alpha_shapes`](https://github.com/pysal/libpysal/blob/master/libpysal/cg/alpha_shapes.py) could be moved so that it doesn't result in an [allowed failure downstream](https://travis-ci.com/jGaboardi/spaghetti/jobs/246506682).\r\n\r\nThis can be accomplished by:\r\n1. [removing the initial import statement at the top of the script](https://github.com/pysal/libpysal/blob/c01fce5293629f361b5e6f6616cf63104fd1b1b7/libpysal/cg/alpha_shapes.py#L33)\r\n2. [moving the import statement outside the try block of in `alpha_shapes_auto`](https://github.com/pysal/libpysal/blob/c01fce5293629f361b5e6f6616cf63104fd1b1b7/libpysal/cg/alpha_shapes.py#L536)\r\n3. [adding the `geom.` prefix to `Point` in this list comprehension](https://github.com/pysal/libpysal/blob/c01fce5293629f361b5e6f6616cf63104fd1b1b7/libpysal/cg/alpha_shapes.py#L553)"},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/200',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/200/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/200/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/200/events',
'html_url': 'https://github.com/pysal/libpysal/pull/200',
'id': 508476901,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI5MjYyNTUy',
'number': 200,
'title': 'fix quasi-redundant import of shapely',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1000808619,
'node_id': 'MDU6TGFiZWwxMDAwODA4NjE5',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/rough%20edge',
'name': 'rough edge',
'color': '5621dd',
'default': False,
'description': 'Something that\'s not a bug, but that gets in the way of "it just works."'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2019-10-17T13:30:08Z',
'updated_at': '2019-10-17T15:26:28Z',
'closed_at': '2019-10-17T15:25:45Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/200',
'html_url': 'https://github.com/pysal/libpysal/pull/200',
'diff_url': 'https://github.com/pysal/libpysal/pull/200.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/200.patch'},
'body': 'The justification for this PR is:\r\n- Addressing #199 \r\n- ~~Adjusting docstring examples~~\r\n- Removing extra spaces\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/196',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/196/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/196/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/196/events',
'html_url': 'https://github.com/pysal/libpysal/pull/196',
'id': 507922686,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI4ODE4ODQ2',
'number': 196,
'title': 'Remove more relics (from pre-reorg PySAL)',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1280158628,
'node_id': 'MDU6TGFiZWwxMjgwMTU4NjI4',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/maintenance',
'name': 'maintenance',
'color': 'c2e0c6',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 0,
'created_at': '2019-10-16T15:20:39Z',
'updated_at': '2019-10-17T11:59:36Z',
'closed_at': '2019-10-17T02:12:01Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/196',
'html_url': 'https://github.com/pysal/libpysal/pull/196',
'diff_url': 'https://github.com/pysal/libpysal/pull/196.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/196.patch'},
'body': "The justification for this PR is: \r\n - related to #193 (removing relics from pre-reorg PySAL)\r\n - `INSTALL.txt`: refers to Python 2.5 and archaic install methods (`easy_install`)\r\n - `tools/*` (***except `gitcount.ipynb`***): mostly old test code, some with hard code paths for a particular dev's machine."},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/197',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/197/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/197/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/197/events',
'html_url': 'https://github.com/pysal/libpysal/issues/197',
'id': 508181726,
'node_id': 'MDU6SXNzdWU1MDgxODE3MjY=',
'number': 197,
'title': '[BUG] alpha_shapes/shapely import error',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2019-10-17T01:34:53Z',
'updated_at': '2019-10-17T01:55:13Z',
'closed_at': '2019-10-17T01:55:13Z',
'author_association': 'MEMBER',
'body': 'Although tests passed locally and on Travis CI for #190 a bug somehow snuck through causing Travis failures downstream (i.e. [`spaghetti`](https://travis-ci.com/jGaboardi/spaghetti/jobs/246506682)). The offender in from line 33 in [`cg.alpha_shapes.py`](https://github.com/pysal/libpysal/blob/d0ff1e0b462bc4df109a1530649c9364e9f6c1c7/libpysal/cg/alpha_shapes.py#L33):\r\n\r\n```python\r\nfrom shapely.geometry.point import Point\r\n```\r\n\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/198',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/198/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/198/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/198/events',
'html_url': 'https://github.com/pysal/libpysal/pull/198',
'id': 508184429,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI5MDI3OTE1',
'number': 198,
'title': '[BUG] correcting shapely import bug',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2019-10-17T01:47:01Z',
'updated_at': '2019-10-17T01:55:55Z',
'closed_at': '2019-10-17T01:54:29Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/198',
'html_url': 'https://github.com/pysal/libpysal/pull/198',
'diff_url': 'https://github.com/pysal/libpysal/pull/198.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/198.patch'},
'body': 'The justification for this PR is: \r\n - Patches the minor bug introduced in #190 \r\n - Addresses #197 \r\n - The bug causes [Travis failures in `spaghetti`](https://travis-ci.com/jGaboardi/spaghetti/jobs/246506682), but was not caught when originally merged.'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/194',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/194/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/194/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/194/events',
'html_url': 'https://github.com/pysal/libpysal/issues/194',
'id': 507265216,
'node_id': 'MDU6SXNzdWU1MDcyNjUyMTY=',
'number': 194,
'title': 'README.txt refers to pre-reorg PySAL',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1280158628,
'node_id': 'MDU6TGFiZWwxMjgwMTU4NjI4',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/maintenance',
'name': 'maintenance',
'color': 'c2e0c6',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2019-10-15T14:00:16Z',
'updated_at': '2019-10-16T14:45:17Z',
'closed_at': '2019-10-16T14:45:17Z',
'author_association': 'MEMBER',
'body': '[`README.txt`](https://github.com/pysal/libpysal/blob/master/README.txt):\r\n - refers to the structure of pre-reorganization of PySAL\r\n - `pysal.spatial_dynamics`, `pysal.network`, etc...\r\n - seems not to be used for any purpose \r\n\r\n\r\nrelated to #147 & #193'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/147',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/147/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/147/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/147/events',
'html_url': 'https://github.com/pysal/libpysal/issues/147',
'id': 422744045,
'node_id': 'MDU6SXNzdWU0MjI3NDQwNDU=',
'number': 147,
'title': 'remove `distribute_setup.py`?',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1280158628,
'node_id': 'MDU6TGFiZWwxMjgwMTU4NjI4',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/maintenance',
'name': 'maintenance',
'color': 'c2e0c6',
'default': False,
'description': ''},
{'id': 536186562,
'node_id': 'MDU6TGFiZWw1MzYxODY1NjI=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/question',
'name': 'question',
'color': 'cc317c',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 2,
'created_at': '2019-03-19T13:52:12Z',
'updated_at': '2019-10-16T14:44:56Z',
'closed_at': '2019-10-16T14:44:56Z',
'author_association': 'MEMBER',
'body': '[`distribute_setup.py`](https://github.com/pysal/libpysal/blob/master/distribute_setup.py) is no longer used in the `libpysal` [`setup.py`](https://github.com/pysal/libpysal/blob/master/setup.py). Should it be considered for removal?'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/128',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/128/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/128/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/128/events',
'html_url': 'https://github.com/pysal/libpysal/issues/128',
'id': 390924095,
'node_id': 'MDU6SXNzdWUzOTA5MjQwOTU=',
'number': 128,
'title': 'requires() decorator for libpysal.cg.alpha_shapes',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1000808619,
'node_id': 'MDU6TGFiZWwxMDAwODA4NjE5',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/rough%20edge',
'name': 'rough edge',
'color': '5621dd',
'default': False,
'description': 'Something that\'s not a bug, but that gets in the way of "it just works."'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2018-12-14T01:03:20Z',
'updated_at': '2019-10-16T14:42:06Z',
'closed_at': '2019-10-16T14:42:06Z',
'author_association': 'MEMBER',
'body': 'Is there a reason not to use the [`requires(\'geopandas\')` decorator](https://github.com/pysal/libpysal/blob/7b27a40b2283ea79087ed823513c57759c513a6d/libpysal/common.py#L100) for [`alpha_shape()`](https://github.com/pysal/libpysal/blob/7b27a40b2283ea79087ed823513c57759c513a6d/libpysal/cg/alpha_shapes.py#L413) and [`alpha_shape_auto()`](https://github.com/pysal/libpysal/blob/7b27a40b2283ea79087ed823513c57759c513a6d/libpysal/cg/alpha_shapes.py#L466), rather than the following in [`alpha_geoms()`](https://github.com/pysal/libpysal/blob/7b27a40b2283ea79087ed823513c57759c513a6d/libpysal/cg/alpha_shapes.py#L395)?\r\n\r\n```python\r\n try:\r\n from shapely.geometry import LineString\r\n from shapely.ops import polygonize\r\n except ImportError:\r\n raise ImportError("Shapely is a required package to use alpha_shapes")\r\n\r\n try:\r\n from geopandas import GeoSeries\r\n except ImportError:\r\n raise ImportError("Geopandas is a required package to use alpha_shapes")\r\n```\r\n\r\nIf `geopandas` is available, then a working install of `shapely` should also be available. However, to be on the safe side the function could be decorated with `requires(\'geopandas\', \'shapely\')`\r\n\r\n----------------------------\r\n\r\n- Platform information:\r\nposix darwin\r\nposix.uname_result(sysname=\'Darwin\', nodename=\'The-Gaboardi.local\', release=\'18.2.0\', version=\'Darwin Kernel Version 18.2.0: Mon Nov 12 20:24:46 PST 2018; root:xnu-4903.231.4~2/RELEASE_X86_64\', machine=\'x86_64\')\r\n\r\n- Python version: \r\n3.6.6 | packaged by conda-forge | (default, Jul 26 2018, 09:55:02) \r\n[GCC 4.2.1 Compatible Apple LLVM 6.1.0 (clang-602.0.53)]\r\n\r\n- SciPy version:\r\n1.1.0\r\n\r\n- NumPy version:\r\n1.14.3'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/129',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/129/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/129/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/129/events',
'html_url': 'https://github.com/pysal/libpysal/pull/129',
'id': 390927408,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MjM4NTgzNDM5',
'number': 129,
'title': 'decorating functions with requires()',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186559,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTk=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1000808619,
'node_id': 'MDU6TGFiZWwxMDAwODA4NjE5',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/rough%20edge',
'name': 'rough edge',
'color': '5621dd',
'default': False,
'description': 'Something that\'s not a bug, but that gets in the way of "it just works."'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 3,
'created_at': '2018-12-14T01:20:01Z',
'updated_at': '2019-10-16T14:47:59Z',
'closed_at': '2019-10-16T03:19:11Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/129',
'html_url': 'https://github.com/pysal/libpysal/pull/129',
'diff_url': 'https://github.com/pysal/libpysal/pull/129.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/129.patch'},
'body': 'The justification for this PR is: \r\n\r\nAddressing #128 (should this implementation be desired).'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/193',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/193/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/193/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/193/events',
'html_url': 'https://github.com/pysal/libpysal/pull/193',
'id': 507259057,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI4MjgyMTk3',
'number': 193,
'title': '[WIP] removing unused relics',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1032340595,
'node_id': 'MDU6TGFiZWwxMDMyMzQwNTk1',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/WIP',
'name': 'WIP',
'color': '9b8cf7',
'default': False,
'description': 'Work in progress, do not merge. Discussion only.'},
{'id': 1280158628,
'node_id': 'MDU6TGFiZWwxMjgwMTU4NjI4',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/maintenance',
'name': 'maintenance',
'color': 'c2e0c6',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 2,
'created_at': '2019-10-15T13:50:43Z',
'updated_at': '2019-10-16T14:46:00Z',
'closed_at': '2019-10-16T02:59:39Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/193',
'html_url': 'https://github.com/pysal/libpysal/pull/193',
'diff_url': 'https://github.com/pysal/libpysal/pull/193.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/193.patch'},
'body': 'The justification for this PR is:\r\n - This PR addresses #147 & #194 \r\n - [`distribute_setup.py`](https://github.com/pysal/libpysal/blob/master/distribute_setup.py) is no longer used in the `libpysal` [`setup.py`](https://github.com/pysal/libpysal/blob/master/setup.py) and seems to be a relic from the early days of the PySAL project.\r\n - [`README.txt`](https://github.com/pysal/libpysal/blob/master/README.txt) refers to the structure of pre-reorganization of PySAL (`pysal.spatial_dynamics`, `pysal.network`, etc...) and seems not to be used for any purpose.\r\n\r\nAlthough these are not large files, if they are no longer relevant we should remove them to cut down on the clutter.'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/191',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/191/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/191/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/191/events',
'html_url': 'https://github.com/pysal/libpysal/issues/191',
'id': 506339333,
'node_id': 'MDU6SXNzdWU1MDYzMzkzMzM=',
'number': 191,
'title': 'necessity of libpysal.common.iteritems()?',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1280158628,
'node_id': 'MDU6TGFiZWwxMjgwMTU4NjI4',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/maintenance',
'name': 'maintenance',
'color': 'c2e0c6',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2019-10-13T15:22:03Z',
'updated_at': '2019-10-16T02:45:37Z',
'closed_at': '2019-10-16T02:45:37Z',
'author_association': 'MEMBER',
'body': 'Since the reorganization of PySAL, only 3.5+ is supported. Therefore, it appears [`libpysal.common.iteritems()`](https://github.com/pysal/libpysal/blob/ed098ea900bde6a9913b96e162d64a72a9615e11/libpysal/common.py#L36-L50) is no longer needed in the code base.'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/192',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/192/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/192/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/192/events',
'html_url': 'https://github.com/pysal/libpysal/pull/192',
'id': 506340637,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI3NTY1ODE0',
'number': 192,
'title': 'removing iteritems decorator',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1280158628,
'node_id': 'MDU6TGFiZWwxMjgwMTU4NjI4',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/maintenance',
'name': 'maintenance',
'color': 'c2e0c6',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 0,
'created_at': '2019-10-13T15:32:20Z',
'updated_at': '2019-10-16T14:46:17Z',
'closed_at': '2019-10-16T02:45:21Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/192',
'html_url': 'https://github.com/pysal/libpysal/pull/192',
'diff_url': 'https://github.com/pysal/libpysal/pull/192.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/192.patch'},
'body': '- This PR addresses #191'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/189',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/189/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/189/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/189/events',
'html_url': 'https://github.com/pysal/libpysal/issues/189',
'id': 504766120,
'node_id': 'MDU6SXNzdWU1MDQ3NjYxMjA=',
'number': 189,
'title': 'Voronoi results in weights of different shape than input points',
'user': {'login': 'dillongardner',
'id': 13119614,
'node_id': 'MDQ6VXNlcjEzMTE5NjE0',
'avatar_url': 'https://avatars3.githubusercontent.com/u/13119614?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/dillongardner',
'html_url': 'https://github.com/dillongardner',
'followers_url': 'https://api.github.com/users/dillongardner/followers',
'following_url': 'https://api.github.com/users/dillongardner/following{/other_user}',
'gists_url': 'https://api.github.com/users/dillongardner/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/dillongardner/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/dillongardner/subscriptions',
'organizations_url': 'https://api.github.com/users/dillongardner/orgs',
'repos_url': 'https://api.github.com/users/dillongardner/repos',
'events_url': 'https://api.github.com/users/dillongardner/events{/privacy}',
'received_events_url': 'https://api.github.com/users/dillongardner/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 13,
'created_at': '2019-10-09T16:40:20Z',
'updated_at': '2019-10-15T13:33:08Z',
'closed_at': '2019-10-15T13:33:08Z',
'author_association': 'NONE',
'body': "```\r\nfrom libpysal.weights import Voronoi\r\nimport numpy as np\r\n\r\nnp.random.seed(12345)\r\npoints= np.random.random((500,2))*10 + 10\r\nw_test = Voronoi(points)\r\nassert len(w_test.neighbors) == len(points)\r\n```\r\n\r\nThis fails and I believe it should not. \r\n\r\n- Platform information:\r\n```\r\nposix darwin\r\nposix.uname_result(sysname='Darwin', nodename='xxxx', release='18.6.0', version='Darwin Kernel Version 18.6.0: Thu Apr 25 23:16:27 PDT 2019; root:xnu-4903.261.4~2/RELEASE_X86_64', machine='x86_64')\r\n```\r\n- Python version: \r\n```\r\n3.6.5 (default, Mar 30 2018, 06:41:53)\r\n[GCC 4.2.1 Compatible Apple LLVM 9.0.0 (clang-900.0.39.2)]\r\n```\r\n- SciPy version:\r\n```\r\n1.0.1\r\n```\r\n- NumPy version:\r\n```\r\n1.14.2\r\n```\r\n"},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/190',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/190/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/190/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/190/events',
'html_url': 'https://github.com/pysal/libpysal/pull/190',
'id': 504952864,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI2NDkyNzYx',
'number': 190,
'title': 'BUG: alpha_shape_auto can fail to contain all points in the set.',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 6,
'created_at': '2019-10-09T23:50:52Z',
'updated_at': '2019-10-15T03:21:47Z',
'closed_at': '2019-10-15T03:21:47Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/190',
'html_url': 'https://github.com/pysal/libpysal/pull/190',
'diff_url': 'https://github.com/pysal/libpysal/pull/190.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/190.patch'},
'body': 'In trying to debug #189 the following will reproduce the issue:\r\n<img width="1105" alt="Screen Shot 2019-10-09 at 7 44 33 PM" src="https://user-images.githubusercontent.com/118042/66528282-49de2000-eacd-11e9-95d6-997c52a87d60.png">\r\n\r\n\r\n\r\n\r\nThe issue appears to be that `alpha_shape_auto` can fail to contain all the points in a set as one of the conditions was that the bounding box for the alpha hull and the point set were equal. That was necessary, but not sufficient to ensure all points were contained in the hull.\r\n\r\nAs a result, when clipping the voronoi regions using the alpha hull, a point (and region) could be omitted and thus the number of points would not match the length of the resulting voronoi W. Notice the point in the north east of this diagram. The light green is the alpha shape clipper, blue are the voronoi regions, and in red are the points:\r\n\r\n\r\n<img width="281" alt="Screen Shot 2019-10-09 at 7 54 37 PM" src="https://user-images.githubusercontent.com/118042/66528700-e8b74c00-eace-11e9-803a-8eebd9b15b24.png">\r\n\r\n\r\nThe PR has fixes for this.\r\n\r\n\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/185',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/185/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/185/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/185/events',
'html_url': 'https://github.com/pysal/libpysal/issues/185',
'id': 491044734,
'node_id': 'MDU6SXNzdWU0OTEwNDQ3MzQ=',
'number': 185,
'title': 'WSP(sparse).to_W() has `array`s in weights,neighbors dictionaries, rather than lists. ',
'user': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1000808619,
'node_id': 'MDU6TGFiZWwxMDAwODA4NjE5',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/rough%20edge',
'name': 'rough edge',
'color': '5621dd',
'default': False,
'description': 'Something that\'s not a bug, but that gets in the way of "it just works."'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 2,
'created_at': '2019-09-09T11:41:57Z',
'updated_at': '2019-10-07T15:39:05Z',
'closed_at': '2019-10-07T15:39:05Z',
'author_association': 'MEMBER',
'body': 'All of the `scipy.sparse` stuff provides arrays when you call into `indices` or `indptr` or `data`. \r\n\r\nWhen we use these in [line 1454](https://github.com/pysal/libpysal/blob/e6998425b917d342ece7a569abe5df6a15af220a/libpysal/weights/weights.py#L1454), we never force them to be lists; we accept them as arrays. \r\n\r\nFor stability and consistency, these need to be cast to lists. '},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/186',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/186/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/186/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/186/events',
'html_url': 'https://github.com/pysal/libpysal/pull/186',
'id': 491068771,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzE1NTA5MDM2',
'number': 186,
'title': 'Cast arrays as lists (Issue 185) ',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1000808619,
'node_id': 'MDU6TGFiZWwxMDAwODA4NjE5',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/rough%20edge',
'name': 'rough edge',
'color': '5621dd',
'default': False,
'description': 'Something that\'s not a bug, but that gets in the way of "it just works."'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 0,
'created_at': '2019-09-09T12:34:43Z',
'updated_at': '2019-10-07T15:41:28Z',
'closed_at': '2019-10-07T15:37:28Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/186',
'html_url': 'https://github.com/pysal/libpysal/pull/186',
'diff_url': 'https://github.com/pysal/libpysal/pull/186.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/186.patch'},
'body': 'Addressing #185 \r\n\r\n* casting `self.sparse.indices`, `self.sparse.data`, and `self.sparse.indptr` as lists\r\n* adjusting [`docstring`](https://github.com/pysal/libpysal/blob/e6998425b917d342ece7a569abe5df6a15af220a/libpysal/weights/spintW.py#L46-L47) in `weights/spintW.py` to conform to `list` structure\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/188',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/188/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/188/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/188/events',
'html_url': 'https://github.com/pysal/libpysal/pull/188',
'id': 503163202,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI1MDc2Mzk3',
'number': 188,
'title': 'BUG: Update for geopandas use of GeometryArray',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2019-10-06T21:28:19Z',
'updated_at': '2019-10-07T15:36:12Z',
'closed_at': '2019-10-07T15:36:12Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/188',
'html_url': 'https://github.com/pysal/libpysal/pull/188',
'diff_url': 'https://github.com/pysal/libpysal/pull/188.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/188.patch'},
'body': 'A few tests in cg alphashapes were failing due to the gp update.'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/187',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/187/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/187/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/187/events',
'html_url': 'https://github.com/pysal/libpysal/pull/187',
'id': 493573964,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzE3NTI4Mzkx',
'number': 187,
'title': 'Updated documentation error (link incorrectly specified) in README.rst ',
'user': {'login': 'Siddharths8212376',
'id': 40669232,
'node_id': 'MDQ6VXNlcjQwNjY5MjMy',
'avatar_url': 'https://avatars2.githubusercontent.com/u/40669232?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/Siddharths8212376',
'html_url': 'https://github.com/Siddharths8212376',
'followers_url': 'https://api.github.com/users/Siddharths8212376/followers',
'following_url': 'https://api.github.com/users/Siddharths8212376/following{/other_user}',
'gists_url': 'https://api.github.com/users/Siddharths8212376/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/Siddharths8212376/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/Siddharths8212376/subscriptions',
'organizations_url': 'https://api.github.com/users/Siddharths8212376/orgs',
'repos_url': 'https://api.github.com/users/Siddharths8212376/repos',
'events_url': 'https://api.github.com/users/Siddharths8212376/events{/privacy}',
'received_events_url': 'https://api.github.com/users/Siddharths8212376/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1376367312,
'node_id': 'MDU6TGFiZWwxMzc2MzY3MzEy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/docs',
'name': 'docs',
'color': 'ffc8b2',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 0,
'created_at': '2019-09-14T02:39:42Z',
'updated_at': '2019-09-16T17:20:29Z',
'closed_at': '2019-09-16T17:20:29Z',
'author_association': 'CONTRIBUTOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/187',
'html_url': 'https://github.com/pysal/libpysal/pull/187',
'diff_url': 'https://github.com/pysal/libpysal/pull/187.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/187.patch'},
'body': 'Hello! Please make sure to check all these boxes before submitting a Pull Request\r\n(PR). Once you have checked the boxes, feel free to remove all text except the\r\njustification in point 5. \r\n\r\n1. [x] You have run tests on this submission, either by using [Travis Continuous Integration testing](https://github.com/pysal/pysal/wiki/GitHub-Standard-Operating-Procedures#automated-testing-w-travis-ci) testing or running `nosetests` on your changes?\r\n2. [x] This pull request is directed to the `pysal/master` branch.\r\n3. [x] This pull introduces new functionality covered by\r\n [docstrings](https://en.wikipedia.org/wiki/Docstring#Python) and\r\n [unittests](https://docs.python.org/2/library/unittest.html)? \r\n4. [x] You have [assigned a\r\n reviewer](https://help.github.com/articles/assigning-issues-and-pull-requests-to-other-github-users/) and added relevant [labels](https://help.github.com/articles/applying-labels-to-issues-and-pull-requests/)\r\n5. [x] The justification for this PR is: The contribution guidelines link was incorrectly specified in the README.rst file. Also added link to LICENSE.txt file.\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/182',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/182/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/182/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/182/events',
'html_url': 'https://github.com/pysal/libpysal/pull/182',
'id': 487931943,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEzMDY1NTgy',
'number': 182,
'title': 'Docs: badges for pypi',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-09-01T21:17:44Z',
'updated_at': '2019-09-01T21:19:52Z',
'closed_at': '2019-09-01T21:19:52Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/182',
'html_url': 'https://github.com/pysal/libpysal/pull/182',
'diff_url': 'https://github.com/pysal/libpysal/pull/182.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/182.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/178',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/178/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/178/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/178/events',
'html_url': 'https://github.com/pysal/libpysal/issues/178',
'id': 484969640,
'node_id': 'MDU6SXNzdWU0ODQ5Njk2NDA=',
'number': 178,
'title': 'development guidelines link failure',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."},
{'id': 1376367312,
'node_id': 'MDU6TGFiZWwxMzc2MzY3MzEy',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/docs',
'name': 'docs',
'color': 'ffc8b2',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-08-25T19:59:45Z',
'updated_at': '2019-09-01T20:52:43Z',
'closed_at': '2019-09-01T20:52:43Z',
'author_association': 'MEMBER',
'body': 'Since [switching from `readthedocs` to GH hosted docs](https://github.com/pysal/pysal/pull/1134), the [development guidelines](https://pysal.readthedocs.io/en/latest/developers/index.html) link is broken. \r\n\r\nShould the `development guidelines` link be:\r\n\r\n- pointing directly to the [wiki](https://github.com/pysal/pysal/wiki), as it on the [Community Guidelines](http://pysal.org/community) page, or \r\n- simply to the Community Guidelines page?'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/181',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/181/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/181/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/181/events',
'html_url': 'https://github.com/pysal/libpysal/pull/181',
'id': 487928809,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEzMDYzNDQy',
'number': 181,
'title': 'DOCS: moving off rtd',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-09-01T20:40:12Z',
'updated_at': '2019-09-01T20:51:55Z',
'closed_at': '2019-09-01T20:50:50Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/181',
'html_url': 'https://github.com/pysal/libpysal/pull/181',
'diff_url': 'https://github.com/pysal/libpysal/pull/181.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/181.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/180',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/180/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/180/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/180/events',
'html_url': 'https://github.com/pysal/libpysal/pull/180',
'id': 487924127,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEzMDYwMTI1',
'number': 180,
'title': 'REL 4.1.1 bf release',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-09-01T19:49:52Z',
'updated_at': '2019-09-01T19:54:46Z',
'closed_at': '2019-09-01T19:54:46Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/180',
'html_url': 'https://github.com/pysal/libpysal/pull/180',
'diff_url': 'https://github.com/pysal/libpysal/pull/180.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/180.patch'},
'body': 'Changelog'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/179',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/179/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/179/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/179/events',
'html_url': 'https://github.com/pysal/libpysal/pull/179',
'id': 487922620,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEzMDU5MDk0',
'number': 179,
'title': 'BUG: Updating manifest for additional requirements files',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-09-01T19:31:32Z',
'updated_at': '2019-09-01T19:44:28Z',
'closed_at': '2019-09-01T19:44:28Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/179',
'html_url': 'https://github.com/pysal/libpysal/pull/179',
'diff_url': 'https://github.com/pysal/libpysal/pull/179.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/179.patch'},
'body': 'We did not update MANIFEST.in when additional requirements files were added to the project.\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/169',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/169/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/169/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/169/events',
'html_url': 'https://github.com/pysal/libpysal/issues/169',
'id': 472421610,
'node_id': 'MDU6SXNzdWU0NzI0MjE2MTA=',
'number': 169,
'title': 'libpysal 4.1.0 is not released on pypi or conda-forge',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
{'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 4,
'created_at': '2019-07-24T18:05:43Z',
'updated_at': '2019-08-31T17:07:32Z',
'closed_at': '2019-08-31T17:07:32Z',
'author_association': 'MEMBER',
'body': 'The most recent version of libpysal on pypi and conda-forge is v4.0.1 from Oct 2018 https://pypi.org/project/libpysal/#history\r\n\r\nWe should probably make a release on pypi for consistency (conda-forge will automatically update once there is a new release on pypi).'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/131',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/131/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/131/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/131/events',
'html_url': 'https://github.com/pysal/libpysal/issues/131',
'id': 401509858,
'node_id': 'MDU6SXNzdWU0MDE1MDk4NTg=',
'number': 131,
'title': 'addressing DeprecationWarning: fromstring()',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1000808619,
'node_id': 'MDU6TGFiZWwxMDAwODA4NjE5',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/rough%20edge',
'name': 'rough edge',
'color': '5621dd',
'default': False,
'description': 'Something that\'s not a bug, but that gets in the way of "it just works."'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 2,
'created_at': '2019-01-21T21:04:26Z',
'updated_at': '2019-08-24T18:04:46Z',
'closed_at': '2019-08-24T18:04:46Z',
'author_association': 'MEMBER',
'body': 'Mimic `DeprecationWarning ` solution found in pysal/pysal#1073\r\n\r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/175',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/175/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/175/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/175/events',
'html_url': 'https://github.com/pysal/libpysal/pull/175',
'id': 484837679,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwNjI0MzQy',
'number': 175,
'title': 'ENH: fromstring has been deprecated',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 2,
'created_at': '2019-08-24T16:06:11Z',
'updated_at': '2019-08-24T16:17:24Z',
'closed_at': '2019-08-24T16:17:24Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/175',
'html_url': 'https://github.com/pysal/libpysal/pull/175',
'diff_url': 'https://github.com/pysal/libpysal/pull/175.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/175.patch'},
'body': 'This would silence verbose warnings in various test suites in downstream packages. [Example](https://travis-ci.org/sjsrey/geosnap/jobs/575622418#L5600).\r\n\r\nDocumentation on the [deprecation](https://docs.python.org/3/library/array.html#array.array.frombytes).'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/132',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/132/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/132/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/132/events',
'html_url': 'https://github.com/pysal/libpysal/pull/132',
'id': 401519082,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MjQ2NDE5MzQ4',
'number': 132,
'title': 'addressing DeprecationWarning: fromstring()',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186559,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTk=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1000808619,
'node_id': 'MDU6TGFiZWwxMDAwODA4NjE5',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/rough%20edge',
'name': 'rough edge',
'color': '5621dd',
'default': False,
'description': 'Something that\'s not a bug, but that gets in the way of "it just works."'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/libpysal/milestones/3',
'html_url': 'https://github.com/pysal/libpysal/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/milestones/3/labels',
'id': 4439549,
'node_id': 'MDk6TWlsZXN0b25lNDQzOTU0OQ==',
'number': 3,
'title': 'Next Release',
'description': 'This is the todo list for the Dec. 2019 release of libpysal, the core of the PySAL metapackage. ',
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 13,
'closed_issues': 33,
'state': 'open',
'created_at': '2019-06-25T13:18:11Z',
'updated_at': '2020-01-04T19:33:41Z',
'due_on': '2019-12-31T08:00:00Z',
'closed_at': None},
'comments': 3,
'created_at': '2019-01-21T21:40:49Z',
'updated_at': '2019-08-24T18:04:14Z',
'closed_at': '2019-08-24T16:16:50Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/132',
'html_url': 'https://github.com/pysal/libpysal/pull/132',
'diff_url': 'https://github.com/pysal/libpysal/pull/132.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/132.patch'},
'body': 'The justification for this PR is: \r\n - Addressing #131 \r\n'},
{'url': 'https://api.github.com/repos/pysal/libpysal/issues/172',
'repository_url': 'https://api.github.com/repos/pysal/libpysal',
'labels_url': 'https://api.github.com/repos/pysal/libpysal/issues/172/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/libpysal/issues/172/comments',
'events_url': 'https://api.github.com/repos/pysal/libpysal/issues/172/events',
'html_url': 'https://github.com/pysal/libpysal/pull/172',
'id': 474926207,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzAyNzc4NzYy',
'number': 172,
'title': 'Ci',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 536186557,
'node_id': 'MDU6TGFiZWw1MzYxODY1NTc=',
'url': 'https://api.github.com/repos/pysal/libpysal/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': "functionality that: returns invalid, erroneous, or meaningless results; or doesn't work at all."}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-07-31T04:13:15Z',
'updated_at': '2019-07-31T12:42:27Z',
'closed_at': '2019-07-31T12:42:27Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/libpysal/pulls/172',
'html_url': 'https://github.com/pysal/libpysal/pull/172',
'diff_url': 'https://github.com/pysal/libpysal/pull/172.diff',
'patch_url': 'https://github.com/pysal/libpysal/pull/172.patch'},
'body': '\r\n\r\n1. [X ] You have run tests on this submission, either by using [Travis Continuous Integration testing](https://github.com/pysal/pysal/wiki/GitHub-Standard-Operating-Procedures#automated-testing-w-travis-ci) testing or running `nosetests` on your changes?\r\n2. [ X] This pull request is directed to the `pysal/master` branch.\r\n4. [X ] You have [assigned a\r\n reviewer](https://help.github.com/articles/assigning-issues-and-pull-requests-to-other-github-users/) and added relevant [labels](https://help.github.com/articles/applying-labels-to-issues-and-pull-requests/)\r\n5. [X] The justification for this PR is: modernizing travis build\r\n'}],
'esda': [{'url': 'https://api.github.com/repos/pysal/esda/issues/98',
'repository_url': 'https://api.github.com/repos/pysal/esda',
'labels_url': 'https://api.github.com/repos/pysal/esda/issues/98/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/esda/issues/98/comments',
'events_url': 'https://api.github.com/repos/pysal/esda/issues/98/events',
'html_url': 'https://github.com/pysal/esda/pull/98',
'id': 545464689,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MzM0OTI2',
'number': 98,
'title': 'ENH: adjust tests for new libpysal.examples',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 538806477,
'node_id': 'MDU6TGFiZWw1Mzg4MDY0Nzc=',
'url': 'https://api.github.com/repos/pysal/esda/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-05T20:45:55Z',
'updated_at': '2020-01-11T16:03:53Z',
'closed_at': '2020-01-11T16:03:53Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/esda/pulls/98',
'html_url': 'https://github.com/pysal/esda/pull/98',
'diff_url': 'https://github.com/pysal/esda/pull/98.diff',
'patch_url': 'https://github.com/pysal/esda/pull/98.patch'},
'body': "- use the 'stl' example in tests."},
{'url': 'https://api.github.com/repos/pysal/esda/issues/97',
'repository_url': 'https://api.github.com/repos/pysal/esda',
'labels_url': 'https://api.github.com/repos/pysal/esda/issues/97/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/esda/issues/97/comments',
'events_url': 'https://api.github.com/repos/pysal/esda/issues/97/events',
'html_url': 'https://github.com/pysal/esda/pull/97',
'id': 539963713,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU0ODUxMjIy',
'number': 97,
'title': 'update docs',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-18T22:38:30Z',
'updated_at': '2019-12-19T00:05:44Z',
'closed_at': '2019-12-19T00:05:44Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/esda/pulls/97',
'html_url': 'https://github.com/pysal/esda/pull/97',
'diff_url': 'https://github.com/pysal/esda/pull/97.diff',
'patch_url': 'https://github.com/pysal/esda/pull/97.patch'},
'body': 'this pr:\r\n- adds documentation for the new geosilhouettes\r\n- moves the docs to the new dual directory model for publishing from gh-pages'},
{'url': 'https://api.github.com/repos/pysal/esda/issues/96',
'repository_url': 'https://api.github.com/repos/pysal/esda',
'labels_url': 'https://api.github.com/repos/pysal/esda/issues/96/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/esda/issues/96/comments',
'events_url': 'https://api.github.com/repos/pysal/esda/issues/96/events',
'html_url': 'https://github.com/pysal/esda/pull/96',
'id': 539867450,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU0NzY5MDk4',
'number': 96,
'title': 'DOC: changelog update',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-18T19:17:25Z',
'updated_at': '2019-12-18T19:17:34Z',
'closed_at': '2019-12-18T19:17:33Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/esda/pulls/96',
'html_url': 'https://github.com/pysal/esda/pull/96',
'diff_url': 'https://github.com/pysal/esda/pull/96.diff',
'patch_url': 'https://github.com/pysal/esda/pull/96.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/esda/issues/95',
'repository_url': 'https://api.github.com/repos/pysal/esda',
'labels_url': 'https://api.github.com/repos/pysal/esda/issues/95/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/esda/issues/95/comments',
'events_url': 'https://api.github.com/repos/pysal/esda/issues/95/events',
'html_url': 'https://github.com/pysal/esda/pull/95',
'id': 538085942,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUzMzAwMDYy',
'number': 95,
'title': 'ENH: bumping version and handle array creation error in join counts',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-15T18:13:23Z',
'updated_at': '2019-12-18T19:01:37Z',
'closed_at': '2019-12-18T19:01:37Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/esda/pulls/95',
'html_url': 'https://github.com/pysal/esda/pull/95',
'diff_url': 'https://github.com/pysal/esda/pull/95.diff',
'patch_url': 'https://github.com/pysal/esda/pull/95.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/esda/issues/87',
'repository_url': 'https://api.github.com/repos/pysal/esda',
'labels_url': 'https://api.github.com/repos/pysal/esda/issues/87/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/esda/issues/87/comments',
'events_url': 'https://api.github.com/repos/pysal/esda/issues/87/events',
'html_url': 'https://github.com/pysal/esda/issues/87',
'id': 488248392,
'node_id': 'MDU6SXNzdWU0ODgyNDgzOTI=',
'number': 87,
'title': 'development guidelines link in README.md and README.rst',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 538806475,
'node_id': 'MDU6TGFiZWw1Mzg4MDY0NzU=',
'url': 'https://api.github.com/repos/pysal/esda/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-09-02T15:47:13Z',
'updated_at': '2019-11-30T16:12:37Z',
'closed_at': '2019-11-30T16:12:37Z',
'author_association': 'MEMBER',
'body': 'As per pysal/libpysal#178 and pysal/libpysal#181, "development guidelines" link in `README.md` and `README.rst` is broken in the [Contribute](https://github.com/pysal/esda#contribute) section.\r\n\r\nfrom: ~~http://pysal.readthedocs.io/en/latest/developers/index.html~~\r\nto: https://github.com/pysal/pysal/wiki'},
{'url': 'https://api.github.com/repos/pysal/esda/issues/92',
'repository_url': 'https://api.github.com/repos/pysal/esda',
'labels_url': 'https://api.github.com/repos/pysal/esda/issues/92/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/esda/issues/92/comments',
'events_url': 'https://api.github.com/repos/pysal/esda/issues/92/events',
'html_url': 'https://github.com/pysal/esda/issues/92',
'id': 529536147,
'node_id': 'MDU6SXNzdWU1Mjk1MzYxNDc=',
'number': 92,
'title': '`PYSAL_PYPI` is not defined in `.travis.yml`',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1258134562,
'node_id': 'MDU6TGFiZWwxMjU4MTM0NTYy',
'url': 'https://api.github.com/repos/pysal/esda/labels/WIP',
'name': 'WIP',
'color': 'f7b391',
'default': False,
'description': 'work in progress (for discussion)'},
{'id': 538806480,
'node_id': 'MDU6TGFiZWw1Mzg4MDY0ODA=',
'url': 'https://api.github.com/repos/pysal/esda/labels/question',
'name': 'question',
'color': 'cc317c',
'default': True,
'description': None},
{'id': 1702005680,
'node_id': 'MDU6TGFiZWwxNzAyMDA1Njgw',
'url': 'https://api.github.com/repos/pysal/esda/labels/travis%20ci',
'name': 'travis ci',
'color': '1c1c7a',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/esda/milestones/1',
'html_url': 'https://github.com/pysal/esda/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/esda/milestones/1/labels',
'id': 4468607,
'node_id': 'MDk6TWlsZXN0b25lNDQ2ODYwNw==',
'number': 1,
'title': 'release',
'description': None,
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 3,
'state': 'open',
'created_at': '2019-07-05T16:40:24Z',
'updated_at': '2019-11-30T16:12:16Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2019-11-27T19:30:35Z',
'updated_at': '2019-11-30T16:12:16Z',
'closed_at': '2019-11-30T16:12:16Z',
'author_association': 'MEMBER',
'body': 'Currently `PYSAL_PYPI` is not defined in [`.travis.yml`](https://github.com/pysal/esda/blob/ae770cdcc5b557af8601a2b8d384fddb7a2e4128/.travis.yml#L37-L42) leading to every build being [tested off the current `libpysal` master](https://travis-ci.org/pysal/esda/jobs/617842703#L502) and none being tested off the current `libpysal` release. Is there a reason why `PYSAL_PYPI` is not in the testing matrix anymore? I will put together a PR for this.'},
{'url': 'https://api.github.com/repos/pysal/esda/issues/93',
'repository_url': 'https://api.github.com/repos/pysal/esda',
'labels_url': 'https://api.github.com/repos/pysal/esda/issues/93/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/esda/issues/93/comments',
'events_url': 'https://api.github.com/repos/pysal/esda/issues/93/events',
'html_url': 'https://github.com/pysal/esda/pull/93',
'id': 529546166,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ2NDAzNzEz',
'number': 93,
'title': 'addressing #92 -- .travis.yml issue',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1702005680,
'node_id': 'MDU6TGFiZWwxNzAyMDA1Njgw',
'url': 'https://api.github.com/repos/pysal/esda/labels/travis%20ci',
'name': 'travis ci',
'color': '1c1c7a',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/esda/milestones/1',
'html_url': 'https://github.com/pysal/esda/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/esda/milestones/1/labels',
'id': 4468607,
'node_id': 'MDk6TWlsZXN0b25lNDQ2ODYwNw==',
'number': 1,
'title': 'release',
'description': None,
'creator': {'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 3,
'state': 'open',
'created_at': '2019-07-05T16:40:24Z',
'updated_at': '2019-11-30T16:12:16Z',
'due_on': None,
'closed_at': None},
'comments': 0,
'created_at': '2019-11-27T19:55:04Z',
'updated_at': '2019-11-30T16:11:21Z',
'closed_at': '2019-11-30T16:11:16Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/esda/pulls/93',
'html_url': 'https://github.com/pysal/esda/pull/93',
'diff_url': 'https://github.com/pysal/esda/pull/93.diff',
'patch_url': 'https://github.com/pysal/esda/pull/93.patch'},
'body': 'Addressing #92, regarding\r\n * the exclusion of `pip` released `libpysal` versions from the testing matrix\r\n * streamlining of preamble (python versions, environment variables, etc.)\r\n * minor formatting issues'},
{'url': 'https://api.github.com/repos/pysal/esda/issues/88',
'repository_url': 'https://api.github.com/repos/pysal/esda',
'labels_url': 'https://api.github.com/repos/pysal/esda/issues/88/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/esda/issues/88/comments',
'events_url': 'https://api.github.com/repos/pysal/esda/issues/88/events',
'html_url': 'https://github.com/pysal/esda/pull/88',
'id': 488249750,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEzMzEzODkx',
'number': 88,
'title': 'resolving dev guidelines link',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 538806475,
'node_id': 'MDU6TGFiZWw1Mzg4MDY0NzU=',
'url': 'https://api.github.com/repos/pysal/esda/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-09-02T15:51:04Z',
'updated_at': '2019-09-03T15:51:19Z',
'closed_at': '2019-09-03T15:22:01Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/esda/pulls/88',
'html_url': 'https://github.com/pysal/esda/pull/88',
'diff_url': 'https://github.com/pysal/esda/pull/88.diff',
'patch_url': 'https://github.com/pysal/esda/pull/88.patch'},
'body': 'Addressing #87 '},
{'url': 'https://api.github.com/repos/pysal/esda/issues/80',
'repository_url': 'https://api.github.com/repos/pysal/esda',
'labels_url': 'https://api.github.com/repos/pysal/esda/issues/80/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/esda/issues/80/comments',
'events_url': 'https://api.github.com/repos/pysal/esda/issues/80/events',
'html_url': 'https://github.com/pysal/esda/issues/80',
'id': 474204365,
'node_id': 'MDU6SXNzdWU0NzQyMDQzNjU=',
'number': 80,
'title': 'Update officially supported Python versions',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1469701838,
'node_id': 'MDU6TGFiZWwxNDY5NzAxODM4',
'url': 'https://api.github.com/repos/pysal/esda/labels/docs',
'name': 'docs',
'color': 'e5933b',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-07-29T18:56:26Z',
'updated_at': '2019-08-05T22:30:13Z',
'closed_at': '2019-08-05T22:30:13Z',
'author_association': 'MEMBER',
'body': 'Currently, Python 3.6 and 3.7 are officially supported and tested in [TravisCI](https://github.com/pysal/esda/blob/1a76643b3fdb08546aad9c0be1099e649ff90236/.travis.yml#L7-L20). The documentation in [`setup.py`](https://github.com/pysal/esda/blob/1a76643b3fdb08546aad9c0be1099e649ff90236/setup.py#L73-L80) and [`doc/installation`](https://github.com/pysal/esda/blob/master/doc/installation.rst) should reflect this but still read as supporting Python 3.5 and 3.6.\r\n\r\n- [x] update `setup.py`\r\n- [x] update `doc/installation`'},
{'url': 'https://api.github.com/repos/pysal/esda/issues/74',
'repository_url': 'https://api.github.com/repos/pysal/esda',
'labels_url': 'https://api.github.com/repos/pysal/esda/issues/74/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/esda/issues/74/comments',
'events_url': 'https://api.github.com/repos/pysal/esda/issues/74/events',
'html_url': 'https://github.com/pysal/esda/pull/74',
'id': 467761493,
'node_id': 'MDExOlB1bGxSZXF1ZXN0Mjk3MzQ0MzY5',
'number': 74,
'title': 'ENH - Join count tails',
'user': {'login': 'rose-pearson',
'id': 38264857,
'node_id': 'MDQ6VXNlcjM4MjY0ODU3',
'avatar_url': 'https://avatars0.githubusercontent.com/u/38264857?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/rose-pearson',
'html_url': 'https://github.com/rose-pearson',
'followers_url': 'https://api.github.com/users/rose-pearson/followers',
'following_url': 'https://api.github.com/users/rose-pearson/following{/other_user}',
'gists_url': 'https://api.github.com/users/rose-pearson/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/rose-pearson/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/rose-pearson/subscriptions',
'organizations_url': 'https://api.github.com/users/rose-pearson/orgs',
'repos_url': 'https://api.github.com/users/rose-pearson/repos',
'events_url': 'https://api.github.com/users/rose-pearson/events{/privacy}',
'received_events_url': 'https://api.github.com/users/rose-pearson/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 10,
'created_at': '2019-07-13T20:06:53Z',
'updated_at': '2019-08-03T19:21:58Z',
'closed_at': '2019-08-03T19:21:58Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/esda/pulls/74',
'html_url': 'https://github.com/pysal/esda/pull/74',
'diff_url': 'https://github.com/pysal/esda/pull/74.diff',
'patch_url': 'https://github.com/pysal/esda/pull/74.patch'},
'body': 'In response to https://github.com/pysal/esda/issues/48.\r\n\r\n1. Added autocorr_neg and autocorr_pos as instance attributes to Join_Counts class\r\n2. Added relevant tests to the Join_Counts_Tester class\r\n\r\nWill create a separate branch on my fork / fork off my fork to update the docs associated with Join_Counts'},
{'url': 'https://api.github.com/repos/pysal/esda/issues/83',
'repository_url': 'https://api.github.com/repos/pysal/esda',
'labels_url': 'https://api.github.com/repos/pysal/esda/issues/83/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/esda/issues/83/comments',
'events_url': 'https://api.github.com/repos/pysal/esda/issues/83/events',
'html_url': 'https://github.com/pysal/esda/pull/83',
'id': 476473409,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA0MDE0ODQ1',
'number': 83,
'title': 'DOC: Have notebooks show output in the src',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-03T17:39:03Z',
'updated_at': '2019-08-03T17:46:01Z',
'closed_at': '2019-08-03T17:46:01Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/esda/pulls/83',
'html_url': 'https://github.com/pysal/esda/pull/83',
'diff_url': 'https://github.com/pysal/esda/pull/83.diff',
'patch_url': 'https://github.com/pysal/esda/pull/83.patch'},
'body': 'Fix for #82 '},
{'url': 'https://api.github.com/repos/pysal/esda/issues/82',
'repository_url': 'https://api.github.com/repos/pysal/esda',
'labels_url': 'https://api.github.com/repos/pysal/esda/issues/82/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/esda/issues/82/comments',
'events_url': 'https://api.github.com/repos/pysal/esda/issues/82/events',
'html_url': 'https://github.com/pysal/esda/issues/82',
'id': 476283406,
'node_id': 'MDU6SXNzdWU0NzYyODM0MDY=',
'number': 82,
'title': 'no output in documentation notebooks',
'user': {'login': 'apoorvalal',
'id': 12086926,
'node_id': 'MDQ6VXNlcjEyMDg2OTI2',
'avatar_url': 'https://avatars1.githubusercontent.com/u/12086926?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/apoorvalal',
'html_url': 'https://github.com/apoorvalal',
'followers_url': 'https://api.github.com/users/apoorvalal/followers',
'following_url': 'https://api.github.com/users/apoorvalal/following{/other_user}',
'gists_url': 'https://api.github.com/users/apoorvalal/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/apoorvalal/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/apoorvalal/subscriptions',
'organizations_url': 'https://api.github.com/users/apoorvalal/orgs',
'repos_url': 'https://api.github.com/users/apoorvalal/repos',
'events_url': 'https://api.github.com/users/apoorvalal/events{/privacy}',
'received_events_url': 'https://api.github.com/users/apoorvalal/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-08-02T17:29:48Z',
'updated_at': '2019-08-03T17:45:49Z',
'closed_at': '2019-08-03T17:45:49Z',
'author_association': 'NONE',
'body': 'Can someone who has access to the data run the [example notebook on the website](https://nbviewer.jupyter.org/github/pysal/esda/blob/master/notebooks/Spatial%20Autocorrelation%20for%20Areal%20Unit%20Data.ipynb) ? There is no output at the moment, which sort of defeats the purpose.\r\n\r\nThanks for the great package!'}],
'giddy': [{'url': 'https://api.github.com/repos/pysal/giddy/issues/131',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/131/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/131/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/131/events',
'html_url': 'https://github.com/pysal/giddy/pull/131',
'id': 553249671,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY1NjE1NjQz',
'number': 131,
'title': '(bug) sojourn_time for p with rows of 0s',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 605847208,
'node_id': 'MDU6TGFiZWw2MDU4NDcyMDg=',
'url': 'https://api.github.com/repos/pysal/giddy/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-22T01:17:05Z',
'updated_at': '2020-01-22T20:48:19Z',
'closed_at': '2020-01-22T20:48:19Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/giddy/pulls/131',
'html_url': 'https://github.com/pysal/giddy/pull/131',
'diff_url': 'https://github.com/pysal/giddy/pull/131.diff',
'patch_url': 'https://github.com/pysal/giddy/pull/131.patch'},
'body': 'This PR is \r\n* to fix the bug of [giddy.markov.sojourn_time](https://github.com/pysal/giddy/blob/master/giddy/markov.py#L2010) to deal with the case when the input transition probability matrix has rows full of 0s\r\n* to fix some inline docstrings '},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/129',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/129/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/129/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/129/events',
'html_url': 'https://github.com/pysal/giddy/issues/129',
'id': 545319008,
'node_id': 'MDU6SXNzdWU1NDUzMTkwMDg=',
'number': 129,
'title': 'Links broken with https://github.com/pysal/giddy/tutorial',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 605847208,
'node_id': 'MDU6TGFiZWw2MDU4NDcyMDg=',
'url': 'https://api.github.com/repos/pysal/giddy/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1412766579,
'node_id': 'MDU6TGFiZWwxNDEyNzY2NTc5',
'url': 'https://api.github.com/repos/pysal/giddy/labels/docs',
'name': 'docs',
'color': '0052cc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2020-01-04T18:59:26Z',
'updated_at': '2020-01-06T17:42:05Z',
'closed_at': '2020-01-06T17:42:05Z',
'author_association': 'MEMBER',
'body': 'Links broken to the source notebooks within https://github.com/pysal/giddy/tutorial.\r\n\r\ni.e. \r\n> This page was generated from [`doc/notebooks/MarkovBasedMethods.ipynb`](https://github.com/pysal/giddy/doc/notebooks/MarkovBasedMethods.ipynb)\r\n\r\nI will push up a fix for this based on what I did in [`spaghetti`](https://github.com/pysal/spaghetti/blob/056a170e0dfcc924ee85a00a2e5d4cc3253cd0cd/docsrc/conf.py#L273) shortly.\r\n\r\n'},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/130',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/130/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/130/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/130/events',
'html_url': 'https://github.com/pysal/giddy/pull/130',
'id': 545320857,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjM4OTQw',
'number': 130,
'title': 'fix for #129',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 605847208,
'node_id': 'MDU6TGFiZWw2MDU4NDcyMDg=',
'url': 'https://api.github.com/repos/pysal/giddy/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1412766579,
'node_id': 'MDU6TGFiZWwxNDEyNzY2NTc5',
'url': 'https://api.github.com/repos/pysal/giddy/labels/docs',
'name': 'docs',
'color': '0052cc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2020-01-04T19:09:21Z',
'updated_at': '2020-01-06T17:53:23Z',
'closed_at': '2020-01-06T17:41:45Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/giddy/pulls/130',
'html_url': 'https://github.com/pysal/giddy/pull/130',
'diff_url': 'https://github.com/pysal/giddy/pull/130.diff',
'patch_url': 'https://github.com/pysal/giddy/pull/130.patch'},
'body': 'This PR provides a fix for the broken link issue raised in #129 '},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/128',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/128/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/128/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/128/events',
'html_url': 'https://github.com/pysal/giddy/pull/128',
'id': 545094584,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MDcwNjM4',
'number': 128,
'title': 'github page redirected to readthedocs page',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2020-01-03T17:54:34Z',
'updated_at': '2020-01-06T18:21:56Z',
'closed_at': '2020-01-03T17:58:18Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/giddy/pulls/128',
'html_url': 'https://github.com/pysal/giddy/pull/128',
'diff_url': 'https://github.com/pysal/giddy/pull/128.diff',
'patch_url': 'https://github.com/pysal/giddy/pull/128.patch'},
'body': 'https://pysal.org/giddy/ will be redirected to https://giddy.readthedocs.io/'},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/107',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/107/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/107/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/107/events',
'html_url': 'https://github.com/pysal/giddy/issues/107',
'id': 465053694,
'node_id': 'MDU6SXNzdWU0NjUwNTM2OTQ=',
'number': 107,
'title': 'Extend functions for Markov classes to deal with non-ergodic Markov chains',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 605847210,
'node_id': 'MDU6TGFiZWw2MDU4NDcyMTA=',
'url': 'https://api.github.com/repos/pysal/giddy/labels/enhancement',
'name': 'enhancement',
'color': 'efd74f',
'default': True,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/giddy/milestones/1',
'html_url': 'https://github.com/pysal/giddy/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/giddy/milestones/1/labels',
'id': 4471584,
'node_id': 'MDk6TWlsZXN0b25lNDQ3MTU4NA==',
'number': 1,
'title': 'release 2.3.0',
'description': None,
'creator': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 3,
'state': 'open',
'created_at': '2019-07-08T04:47:29Z',
'updated_at': '2019-12-21T07:00:31Z',
'due_on': None,
'closed_at': None},
'comments': 0,
'created_at': '2019-07-08T04:54:12Z',
'updated_at': '2019-12-21T07:00:31Z',
'closed_at': '2019-12-21T07:00:31Z',
'author_association': 'MEMBER',
'body': 'It is possible that we run into non-ergodic Markov chains, e.g. it might be impossible to migrate from one Markov state to another. Right now, the [`steady_state`](https://github.com/pysal/giddy/blob/master/giddy/ergodic.py#L12) function for calculating the limiting distribution and the [`fmpt`](https://github.com/pysal/giddy/blob/master/giddy/ergodic.py#L62) function calculating first mean passage times can only deal with non-ergodic Markov chains. It will be useful to extend these functions to deal with non-ergodic Markov chains.\r\n'},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/127',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/127/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/127/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/127/events',
'html_url': 'https://github.com/pysal/giddy/pull/127',
'id': 541292381,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU1OTc2Mjkx',
'number': 127,
'title': 'prepare for releasing V2.3.0',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-21T06:46:42Z',
'updated_at': '2019-12-21T06:58:21Z',
'closed_at': '2019-12-21T06:58:20Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/giddy/pulls/127',
'html_url': 'https://github.com/pysal/giddy/pull/127',
'diff_url': 'https://github.com/pysal/giddy/pull/127.diff',
'patch_url': 'https://github.com/pysal/giddy/pull/127.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/109',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/109/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/109/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/109/events',
'html_url': 'https://github.com/pysal/giddy/pull/109',
'id': 466000570,
'node_id': 'MDExOlB1bGxSZXF1ZXN0Mjk1OTMyNTE3',
'number': 109,
'title': '(ENH) Extend functions for Markov classes to deal with non-ergodic Markov chains',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 605847210,
'node_id': 'MDU6TGFiZWw2MDU4NDcyMTA=',
'url': 'https://api.github.com/repos/pysal/giddy/labels/enhancement',
'name': 'enhancement',
'color': 'efd74f',
'default': True,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/giddy/milestones/1',
'html_url': 'https://github.com/pysal/giddy/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/giddy/milestones/1/labels',
'id': 4471584,
'node_id': 'MDk6TWlsZXN0b25lNDQ3MTU4NA==',
'number': 1,
'title': 'release 2.3.0',
'description': None,
'creator': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 3,
'state': 'open',
'created_at': '2019-07-08T04:47:29Z',
'updated_at': '2019-12-21T07:00:31Z',
'due_on': None,
'closed_at': None},
'comments': 5,
'created_at': '2019-07-09T21:50:12Z',
'updated_at': '2019-12-21T06:00:20Z',
'closed_at': '2019-12-21T06:00:20Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/giddy/pulls/109',
'html_url': 'https://github.com/pysal/giddy/pull/109',
'diff_url': 'https://github.com/pysal/giddy/pull/109.diff',
'patch_url': 'https://github.com/pysal/giddy/pull/109.patch'},
'body': 'This PR is to extend functions for Markov classes to deal with non-ergodic Markov chains. \r\n\r\nInstead of changing the behavior of existing functions like `giddy.ergodic.steady_state` and `giddy.ergodic.fmpt` which explicitly claim that they are only suitable for regular Markov chain, I added two functions: \r\n* [`giddy.ergodic.steady_state_general`](https://github.com/weikang9009/giddy/blob/limiting/giddy/ergodic.py#L64) \r\n* [`giddy.ergodic.fmpt_general`](https://github.com/weikang9009/giddy/blob/limiting/giddy/ergodic.py#L187)\r\n\r\nwhich could deal with reducible Markov chains. For instance, if the Markov chain has two communicating classes, `giddy.ergodic.steady_state_general` will return an array of two vectors (see [here](https://github.com/weikang9009/giddy/blob/limiting/giddy/ergodic.py#L105) for an example in the inline docstring.), and [`giddy.ergodic.fmpt_general`](https://github.com/weikang9009/giddy/blob/limiting/giddy/ergodic.py#L228) will return an array which contains `np.inf` since it is impossible to get a state in class 1 if the system starts at a state in class 2 (see [here] for an example in the inline docstring.).\r\n\r\nThese two functions replace`giddy.ergodic.steady_state` and `giddy.ergodic.fmpt` in classes `Markov`, `Spatial_Markov`, `FullRank_Markov` and `GeoRank_Markov` for estimating the steady state distributions and first mean passage times (spatially conditional or not).\r\n\r\nAnother enhancement is adding an optional parameter [`fill_diag `](https://github.com/weikang9009/giddy/blob/limiting/giddy/markov.py#L68) to each of these Markov related classes to allow users to determine whether a stochastic transition probability matrix (each row summing to 1) is desired.\r\n\r\n\r\n'},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/126',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/126/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/126/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/126/events',
'html_url': 'https://github.com/pysal/giddy/pull/126',
'id': 532131648,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ4NDQ1Nzgx',
'number': 126,
'title': 'travis CI: pip install from git clone',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 605847208,
'node_id': 'MDU6TGFiZWw2MDU4NDcyMDg=',
'url': 'https://api.github.com/repos/pysal/giddy/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1017693643,
'node_id': 'MDU6TGFiZWwxMDE3NjkzNjQz',
'url': 'https://api.github.com/repos/pysal/giddy/labels/package%20maintenance',
'name': 'package maintenance',
'color': '4de2bf',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/giddy/milestones/1',
'html_url': 'https://github.com/pysal/giddy/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/giddy/milestones/1/labels',
'id': 4471584,
'node_id': 'MDk6TWlsZXN0b25lNDQ3MTU4NA==',
'number': 1,
'title': 'release 2.3.0',
'description': None,
'creator': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 3,
'state': 'open',
'created_at': '2019-07-08T04:47:29Z',
'updated_at': '2019-12-21T07:00:31Z',
'due_on': None,
'closed_at': None},
'comments': 3,
'created_at': '2019-12-03T17:05:05Z',
'updated_at': '2019-12-03T17:54:14Z',
'closed_at': '2019-12-03T17:54:14Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/giddy/pulls/126',
'html_url': 'https://github.com/pysal/giddy/pull/126',
'diff_url': 'https://github.com/pysal/giddy/pull/126.diff',
'patch_url': 'https://github.com/pysal/giddy/pull/126.patch'},
'body': "this PR is to allow for testing against submodules' github master branch in .travis.yml https://github.com/pysal/pysal/issues/1145\r\n"},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/125',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/125/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/125/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/125/events',
'html_url': 'https://github.com/pysal/giddy/pull/125',
'id': 526743139,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ0MTUyNjAx',
'number': 125,
'title': 'rewrite utility function "get_lower" using numpy\'s more efficient functionality',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 605847210,
'node_id': 'MDU6TGFiZWw2MDU4NDcyMTA=',
'url': 'https://api.github.com/repos/pysal/giddy/labels/enhancement',
'name': 'enhancement',
'color': 'efd74f',
'default': True,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-11-21T17:38:31Z',
'updated_at': '2019-11-22T18:36:30Z',
'closed_at': '2019-11-22T18:36:30Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/giddy/pulls/125',
'html_url': 'https://github.com/pysal/giddy/pull/125',
'diff_url': 'https://github.com/pysal/giddy/pull/125.diff',
'patch_url': 'https://github.com/pysal/giddy/pull/125.patch'},
'body': "This PR is to rewrite the utility function `get_lower` to use numpy's more efficient functionalities."},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/121',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/121/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/121/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/121/events',
'html_url': 'https://github.com/pysal/giddy/issues/121',
'id': 488705080,
'node_id': 'MDU6SXNzdWU0ODg3MDUwODA=',
'number': 121,
'title': 'Release version 2.2.2 for bug fix ',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1017693643,
'node_id': 'MDU6TGFiZWwxMDE3NjkzNjQz',
'url': 'https://api.github.com/repos/pysal/giddy/labels/package%20maintenance',
'name': 'package maintenance',
'color': '4de2bf',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-09-03T16:27:03Z',
'updated_at': '2019-09-06T23:47:16Z',
'closed_at': '2019-09-06T23:47:16Z',
'author_association': 'MEMBER',
'body': 'giddy 2.2.2 should be released soon to incorporate the bug fix to the rank method https://github.com/pysal/giddy/pull/118'},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/123',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/123/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/123/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/123/events',
'html_url': 'https://github.com/pysal/giddy/pull/123',
'id': 490558765,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzE1MTQyNzM1',
'number': 123,
'title': 'prepare for new release: version 2.2.2 ',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1017693643,
'node_id': 'MDU6TGFiZWwxMDE3NjkzNjQz',
'url': 'https://api.github.com/repos/pysal/giddy/labels/package%20maintenance',
'name': 'package maintenance',
'color': '4de2bf',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-09-06T23:29:26Z',
'updated_at': '2019-09-06T23:30:09Z',
'closed_at': '2019-09-06T23:30:09Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/giddy/pulls/123',
'html_url': 'https://github.com/pysal/giddy/pull/123',
'diff_url': 'https://github.com/pysal/giddy/pull/123.diff',
'patch_url': 'https://github.com/pysal/giddy/pull/123.patch'},
'body': '* bump version \r\n* changelog\r\n\r\naddress https://github.com/pysal/giddy/issues/121'},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/122',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/122/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/122/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/122/events',
'html_url': 'https://github.com/pysal/giddy/pull/122',
'id': 490531614,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzE1MTIwOTQz',
'number': 122,
'title': 'remove the parameter in plt.show() due to the API change in Matplotlib (deprecation)',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1017693643,
'node_id': 'MDU6TGFiZWwxMDE3NjkzNjQz',
'url': 'https://api.github.com/repos/pysal/giddy/labels/package%20maintenance',
'name': 'package maintenance',
'color': '4de2bf',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-09-06T21:33:13Z',
'updated_at': '2019-09-06T23:14:31Z',
'closed_at': '2019-09-06T23:14:31Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/giddy/pulls/122',
'html_url': 'https://github.com/pysal/giddy/pull/122',
'diff_url': 'https://github.com/pysal/giddy/pull/122.diff',
'patch_url': 'https://github.com/pysal/giddy/pull/122.patch'},
'body': '[The interactive example in the docstring of `Rose`](https://github.com/pysal/giddy/blob/master/giddy/directional.py#L209) plots the rose diagram by calling function `plt.show(fig1)` from Matplotlib, resulting in the following warning:\r\n```python\r\n(base) weis-imac:giddy weikang$ nosetests --with-doctest \r\n./Users/weikang/Google Drive/python_repos/pysal-refactor/giddy/giddy/directional.py:1: MatplotlibDeprecationWarning: Passing the block parameter of show() positionally is deprecated since Matplotlib 3.1; the parameter will become keyword-only in 3.3.\r\n```\r\nThe PR removes the parameter in the function (it is not quite needed) to avoid the warning and potential error once Matplotlib 3.3 is released.'},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/119',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/119/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/119/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/119/events',
'html_url': 'https://github.com/pysal/giddy/issues/119',
'id': 488236402,
'node_id': 'MDU6SXNzdWU0ODgyMzY0MDI=',
'number': 119,
'title': 'development guidelines link in README.md',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 605847208,
'node_id': 'MDU6TGFiZWw2MDU4NDcyMDg=',
'url': 'https://api.github.com/repos/pysal/giddy/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-09-02T15:14:07Z',
'updated_at': '2019-09-03T16:20:41Z',
'closed_at': '2019-09-03T16:20:41Z',
'author_association': 'MEMBER',
'body': 'As per pysal/libpysal#178 and pysal/libpysal#181, "development guidelines" link in `README.md` is broken in the [Contribute](https://github.com/pysal/giddy#contribute) section.\r\n\r\nfrom: ~~http://pysal.readthedocs.io/en/latest/developers/index.html~~\r\nto: https://github.com/pysal/pysal/wiki'},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/118',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/118/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/118/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/118/events',
'html_url': 'https://github.com/pysal/giddy/pull/118',
'id': 487210720,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEyNTIyODky',
'number': 118,
'title': 'BUG: Fix for correct handling of ties in Tau',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 605847208,
'node_id': 'MDU6TGFiZWw2MDU4NDcyMDg=',
'url': 'https://api.github.com/repos/pysal/giddy/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-08-29T22:57:02Z',
'updated_at': '2019-09-03T16:19:46Z',
'closed_at': '2019-09-03T16:19:46Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/giddy/pulls/118',
'html_url': 'https://github.com/pysal/giddy/pull/118',
'diff_url': 'https://github.com/pysal/giddy/pull/118.diff',
'patch_url': 'https://github.com/pysal/giddy/pull/118.patch'},
'body': 'Fix for #117.'},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/120',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/120/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/120/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/120/events',
'html_url': 'https://github.com/pysal/giddy/pull/120',
'id': 488238158,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEzMzA0NDg5',
'number': 120,
'title': 'resolving broken dev link',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 605847208,
'node_id': 'MDU6TGFiZWw2MDU4NDcyMDg=',
'url': 'https://api.github.com/repos/pysal/giddy/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-09-02T15:18:45Z',
'updated_at': '2019-09-03T15:51:54Z',
'closed_at': '2019-09-03T15:18:17Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/giddy/pulls/120',
'html_url': 'https://github.com/pysal/giddy/pull/120',
'diff_url': 'https://github.com/pysal/giddy/pull/120.diff',
'patch_url': 'https://github.com/pysal/giddy/pull/120.patch'},
'body': 'Addressing #119 '},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/117',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/117/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/117/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/117/events',
'html_url': 'https://github.com/pysal/giddy/issues/117',
'id': 487000407,
'node_id': 'MDU6SXNzdWU0ODcwMDA0MDc=',
'number': 117,
'title': "initialization of ECount parameter in Kendall's Tau",
'user': {'login': 'dima-quant',
'id': 54665019,
'node_id': 'MDQ6VXNlcjU0NjY1MDE5',
'avatar_url': 'https://avatars3.githubusercontent.com/u/54665019?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/dima-quant',
'html_url': 'https://github.com/dima-quant',
'followers_url': 'https://api.github.com/users/dima-quant/followers',
'following_url': 'https://api.github.com/users/dima-quant/following{/other_user}',
'gists_url': 'https://api.github.com/users/dima-quant/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/dima-quant/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/dima-quant/subscriptions',
'organizations_url': 'https://api.github.com/users/dima-quant/orgs',
'repos_url': 'https://api.github.com/users/dima-quant/repos',
'events_url': 'https://api.github.com/users/dima-quant/events{/privacy}',
'received_events_url': 'https://api.github.com/users/dima-quant/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 605847208,
'node_id': 'MDU6TGFiZWw2MDU4NDcyMDg=',
'url': 'https://api.github.com/repos/pysal/giddy/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 3,
'created_at': '2019-08-29T09:42:38Z',
'updated_at': '2019-09-02T09:13:38Z',
'closed_at': '2019-09-02T09:13:38Z',
'author_association': 'NONE',
'body': 'A bug in pysal.spatial_dynamics.rank class Tau: def _calc(self, x, y). Ties are counted incorrectly. Cause: initialization is made with ECount = 0, but should be ECount = 1'},
{'url': 'https://api.github.com/repos/pysal/giddy/issues/116',
'repository_url': 'https://api.github.com/repos/pysal/giddy',
'labels_url': 'https://api.github.com/repos/pysal/giddy/issues/116/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/giddy/issues/116/comments',
'events_url': 'https://api.github.com/repos/pysal/giddy/issues/116/events',
'html_url': 'https://github.com/pysal/giddy/pull/116',
'id': 479769446,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA2NTk0OTg0',
'number': 116,
'title': '(bug) debug travis-ci ',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 605847208,
'node_id': 'MDU6TGFiZWw2MDU4NDcyMDg=',
'url': 'https://api.github.com/repos/pysal/giddy/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-12T17:46:10Z',
'updated_at': '2019-08-12T22:59:21Z',
'closed_at': '2019-08-12T22:59:21Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/giddy/pulls/116',
'html_url': 'https://github.com/pysal/giddy/pull/116',
'diff_url': 'https://github.com/pysal/giddy/pull/116.diff',
'patch_url': 'https://github.com/pysal/giddy/pull/116.patch'},
'body': 'this PR is to debug travis-ci testing https://travis-ci.org/pysal/giddy\r\n\r\nrely on conda-forge instead of pypi for dependency installation (this assumes that conda-forge and pypi are hosting the same release)'}],
'inequality': [{'url': 'https://api.github.com/repos/pysal/inequality/issues/12',
'repository_url': 'https://api.github.com/repos/pysal/inequality',
'labels_url': 'https://api.github.com/repos/pysal/inequality/issues/12/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/inequality/issues/12/comments',
'events_url': 'https://api.github.com/repos/pysal/inequality/issues/12/events',
'html_url': 'https://github.com/pysal/inequality/pull/12',
'id': 464463512,
'node_id': 'MDExOlB1bGxSZXF1ZXN0Mjk0NzM1ODYx',
'number': 12,
'title': 'updating supported python versions (3.6 and 3.7)',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-07-05T05:09:50Z',
'updated_at': '2019-11-28T03:46:16Z',
'closed_at': '2019-11-28T03:46:16Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/inequality/pulls/12',
'html_url': 'https://github.com/pysal/inequality/pull/12',
'diff_url': 'https://github.com/pysal/inequality/pull/12.diff',
'patch_url': 'https://github.com/pysal/inequality/pull/12.patch'},
'body': '* update setup.py\r\n* update travis.yml'}],
'pointpats': [{'url': 'https://api.github.com/repos/pysal/pointpats/issues/45',
'repository_url': 'https://api.github.com/repos/pysal/pointpats',
'labels_url': 'https://api.github.com/repos/pysal/pointpats/issues/45/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/pointpats/issues/45/comments',
'events_url': 'https://api.github.com/repos/pysal/pointpats/issues/45/events',
'html_url': 'https://github.com/pysal/pointpats/pull/45',
'id': 536755477,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUyMjMwOTEy',
'number': 45,
'title': 'BUG: fix for K function',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-12-12T04:50:00Z',
'updated_at': '2019-12-20T00:31:22Z',
'closed_at': '2019-12-20T00:31:22Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/pointpats/pulls/45',
'html_url': 'https://github.com/pysal/pointpats/pull/45',
'diff_url': 'https://github.com/pysal/pointpats/pull/45.diff',
'patch_url': 'https://github.com/pysal/pointpats/pull/45.patch'},
'body': 'This corrects the K function.'},
{'url': 'https://api.github.com/repos/pysal/pointpats/issues/43',
'repository_url': 'https://api.github.com/repos/pysal/pointpats',
'labels_url': 'https://api.github.com/repos/pysal/pointpats/issues/43/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/pointpats/issues/43/comments',
'events_url': 'https://api.github.com/repos/pysal/pointpats/issues/43/events',
'html_url': 'https://github.com/pysal/pointpats/pull/43',
'id': 536655826,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUyMTQ5NzY4',
'number': 43,
'title': '(docs) auto display inherited class members',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1410321276,
'node_id': 'MDU6TGFiZWwxNDEwMzIxMjc2',
'url': 'https://api.github.com/repos/pysal/pointpats/labels/docs',
'name': 'docs',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-11T22:45:03Z',
'updated_at': '2019-12-11T23:02:54Z',
'closed_at': '2019-12-11T23:02:53Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/pointpats/pulls/43',
'html_url': 'https://github.com/pysal/pointpats/pull/43',
'diff_url': 'https://github.com/pysal/pointpats/pull/43.diff',
'patch_url': 'https://github.com/pysal/pointpats/pull/43.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/pointpats/issues/42',
'repository_url': 'https://api.github.com/repos/pysal/pointpats',
'labels_url': 'https://api.github.com/repos/pysal/pointpats/issues/42/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/pointpats/issues/42/comments',
'events_url': 'https://api.github.com/repos/pysal/pointpats/issues/42/events',
'html_url': 'https://github.com/pysal/pointpats/pull/42',
'id': 534296583,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUwMjMxMTA4',
'number': 42,
'title': '(docs) remove generated rst files',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-06T22:56:21Z',
'updated_at': '2019-12-06T23:11:24Z',
'closed_at': '2019-12-06T23:11:24Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/pointpats/pulls/42',
'html_url': 'https://github.com/pysal/pointpats/pull/42',
'diff_url': 'https://github.com/pysal/pointpats/pull/42.diff',
'patch_url': 'https://github.com/pysal/pointpats/pull/42.patch'},
'body': '- remove generated rst files\r\n- configure sphinx to document Members without docstrings'},
{'url': 'https://api.github.com/repos/pysal/pointpats/issues/41',
'repository_url': 'https://api.github.com/repos/pysal/pointpats',
'labels_url': 'https://api.github.com/repos/pysal/pointpats/issues/41/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/pointpats/issues/41/comments',
'events_url': 'https://api.github.com/repos/pysal/pointpats/issues/41/events',
'html_url': 'https://github.com/pysal/pointpats/pull/41',
'id': 534289683,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUwMjI2MDQ1',
'number': 41,
'title': '(docs) update docs',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-06T22:35:07Z',
'updated_at': '2019-12-06T22:42:58Z',
'closed_at': '2019-12-06T22:42:58Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/pointpats/pulls/41',
'html_url': 'https://github.com/pysal/pointpats/pull/41',
'diff_url': 'https://github.com/pysal/pointpats/pull/41.diff',
'patch_url': 'https://github.com/pysal/pointpats/pull/41.patch'},
'body': 'update docs with adjusted style and with more intersphinx mapping packages'},
{'url': 'https://api.github.com/repos/pysal/pointpats/issues/38',
'repository_url': 'https://api.github.com/repos/pysal/pointpats',
'labels_url': 'https://api.github.com/repos/pysal/pointpats/issues/38/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/pointpats/issues/38/comments',
'events_url': 'https://api.github.com/repos/pysal/pointpats/issues/38/events',
'html_url': 'https://github.com/pysal/pointpats/pull/38',
'id': 526302338,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQzNzk0NjYw',
'number': 38,
'title': 'Add space time interaction tests',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-11-21T01:01:46Z',
'updated_at': '2019-12-06T21:56:02Z',
'closed_at': '2019-12-06T21:56:02Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/pointpats/pulls/38',
'html_url': 'https://github.com/pysal/pointpats/pull/38',
'diff_url': 'https://github.com/pysal/pointpats/pull/38.diff',
'patch_url': 'https://github.com/pysal/pointpats/pull/38.patch'},
'body': 'Move functionalities of space time interaction tests from legacy pysal.spatial_dynamics.interaction to `pointpats`:\r\n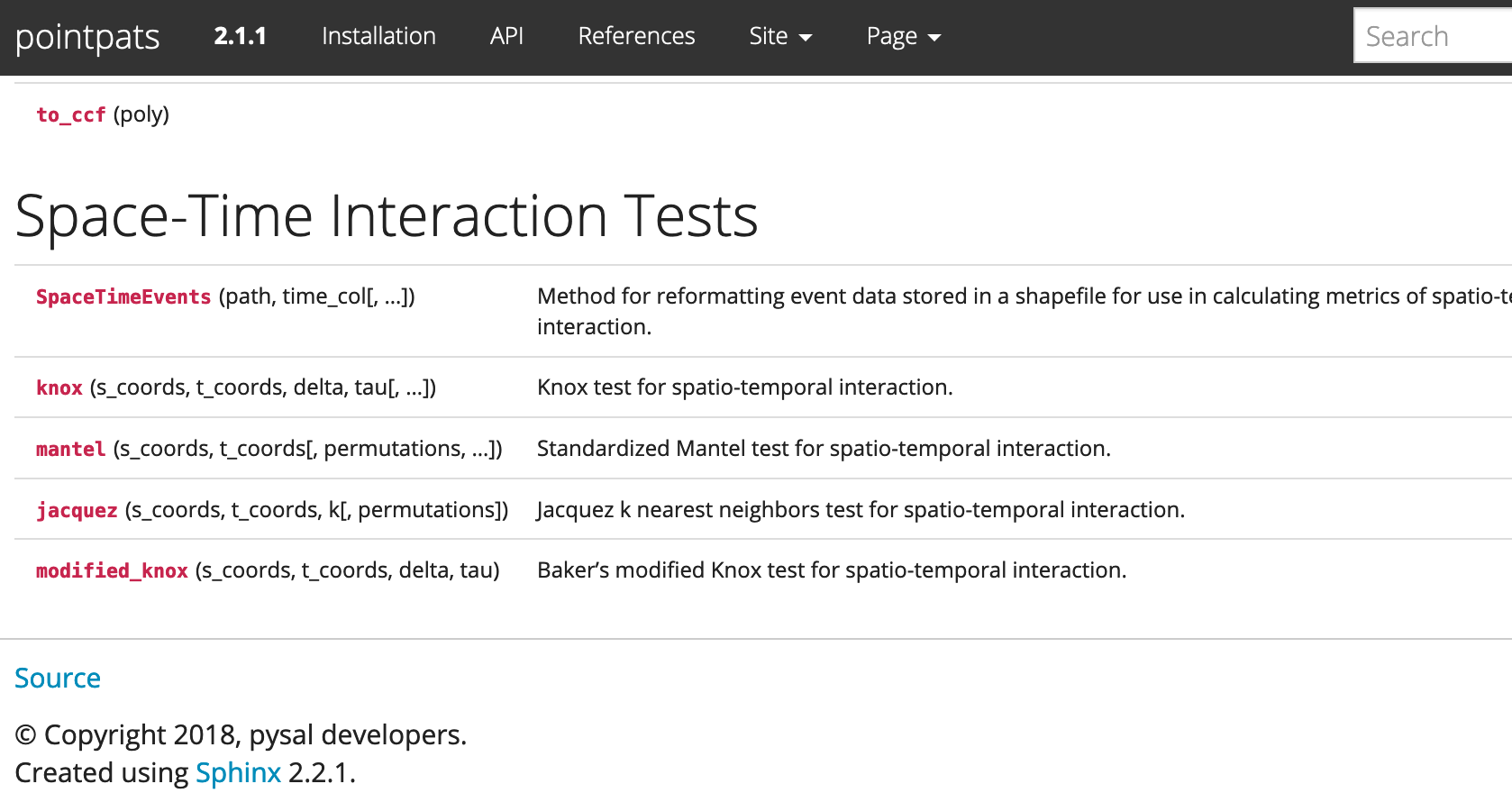\r\n \r\naddress https://github.com/pysal/giddy/issues/124'},
{'url': 'https://api.github.com/repos/pysal/pointpats/issues/39',
'repository_url': 'https://api.github.com/repos/pysal/pointpats',
'labels_url': 'https://api.github.com/repos/pysal/pointpats/issues/39/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/pointpats/issues/39/comments',
'events_url': 'https://api.github.com/repos/pysal/pointpats/issues/39/events',
'html_url': 'https://github.com/pysal/pointpats/pull/39',
'id': 532842986,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ5MDE4NTM1',
'number': 39,
'title': 'updating dual travis test procdeure',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 538797569,
'node_id': 'MDU6TGFiZWw1Mzg3OTc1Njk=',
'url': 'https://api.github.com/repos/pysal/pointpats/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-12-04T17:54:55Z',
'updated_at': '2019-12-04T18:10:10Z',
'closed_at': '2019-12-04T18:09:36Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/pointpats/pulls/39',
'html_url': 'https://github.com/pysal/pointpats/pull/39',
'diff_url': 'https://github.com/pysal/pointpats/pull/39.diff',
'patch_url': 'https://github.com/pysal/pointpats/pull/39.patch'},
'body': 'This PR addresses the faulty dual testing schema within `.travis.yml` raised in pysal/pysal#1145.'}],
'segregation': [{'url': 'https://api.github.com/repos/pysal/segregation/issues/144',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/144/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/144/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/144/events',
'html_url': 'https://github.com/pysal/segregation/pull/144',
'id': 555000773,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY3MDU0MDYy',
'number': 144,
'title': 'move import down',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-24T23:24:33Z',
'updated_at': '2020-01-25T01:04:12Z',
'closed_at': '2020-01-25T01:04:09Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/144',
'html_url': 'https://github.com/pysal/segregation/pull/144',
'diff_url': 'https://github.com/pysal/segregation/pull/144.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/144.patch'},
'body': 'this will raise an error if you try to use the function that requires the packages, as opposed to raising a general warning on import'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/141',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/141/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/141/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/141/events',
'html_url': 'https://github.com/pysal/segregation/pull/141',
'id': 536729795,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUyMjA5OTE4',
'number': 141,
'title': 'addressing pysal/pysal#1145',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-12-12T03:06:15Z',
'updated_at': '2019-12-13T22:16:55Z',
'closed_at': '2019-12-13T18:24:12Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/141',
'html_url': 'https://github.com/pysal/segregation/pull/141',
'diff_url': 'https://github.com/pysal/segregation/pull/141.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/141.patch'},
'body': 'addressing pysal/pysal#1145'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/137',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/137/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/137/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/137/events',
'html_url': 'https://github.com/pysal/segregation/issues/137',
'id': 487165446,
'node_id': 'MDU6SXNzdWU0ODcxNjU0NDY=',
'number': 137,
'title': 'tweaks readme',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-29T20:39:32Z',
'updated_at': '2019-11-10T00:19:07Z',
'closed_at': '2019-11-10T00:19:07Z',
'author_association': 'COLLABORATOR',
'body': 'The sentence `To see the estimated D in the first generic example above, the user would have just to run index.statistic to see the fitted value.` should change its location to after the "d_index" call and be updated to "d_index".\r\n\r\nAlso, we can pt a point after "total_population" and put uppercase "A" in:\r\n\r\n"a typical call would be something like this:"'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/140',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/140/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/140/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/140/events',
'html_url': 'https://github.com/pysal/segregation/pull/140',
'id': 506345907,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI3NTY5NTIy',
'number': 140,
'title': 'Fix ReadMe',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-10-13T16:17:50Z',
'updated_at': '2019-10-14T15:19:12Z',
'closed_at': '2019-10-14T15:19:12Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/140',
'html_url': 'https://github.com/pysal/segregation/pull/140',
'diff_url': 'https://github.com/pysal/segregation/pull/140.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/140.patch'},
'body': 'Address https://github.com/pysal/segregation/issues/137.'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/138',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/138/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/138/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/138/events',
'html_url': 'https://github.com/pysal/segregation/issues/138',
'id': 488214497,
'node_id': 'MDU6SXNzdWU0ODgyMTQ0OTc=',
'number': 138,
'title': 'development guidelines link broken',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1167292078,
'node_id': 'MDU6TGFiZWwxMTY3MjkyMDc4',
'url': 'https://api.github.com/repos/pysal/segregation/labels/bug',
'name': 'bug',
'color': 'd73a4a',
'default': True,
'description': "Something isn't working"}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-09-02T14:22:02Z',
'updated_at': '2019-09-03T06:15:16Z',
'closed_at': '2019-09-03T06:15:16Z',
'author_association': 'MEMBER',
'body': 'As per pysal/libpysal#178 and pysal/libpysal#181\r\n\r\nfrom: ~~http://pysal.readthedocs.io/en/latest/developers/index.html~~\r\nto: https://github.com/pysal/pysal/wiki'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/139',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/139/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/139/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/139/events',
'html_url': 'https://github.com/pysal/segregation/pull/139',
'id': 488215263,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEzMjg1NTc2',
'number': 139,
'title': 'resolving broken link',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1167292078,
'node_id': 'MDU6TGFiZWwxMTY3MjkyMDc4',
'url': 'https://api.github.com/repos/pysal/segregation/labels/bug',
'name': 'bug',
'color': 'd73a4a',
'default': True,
'description': "Something isn't working"}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-09-02T14:23:37Z',
'updated_at': '2019-09-03T15:51:34Z',
'closed_at': '2019-09-03T06:14:49Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/139',
'html_url': 'https://github.com/pysal/segregation/pull/139',
'diff_url': 'https://github.com/pysal/segregation/pull/139.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/139.patch'},
'body': 'Addressing #138 '},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/136',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/136/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/136/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/136/events',
'html_url': 'https://github.com/pysal/segregation/pull/136',
'id': 487153034,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEyNDc1MTY5',
'number': 136,
'title': 'improve inference wrappers docstring',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-29T20:09:01Z',
'updated_at': '2019-08-29T20:34:55Z',
'closed_at': '2019-08-29T20:34:55Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/136',
'html_url': 'https://github.com/pysal/segregation/pull/136',
'diff_url': 'https://github.com/pysal/segregation/pull/136.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/136.patch'},
'body': 'This improves the inference discussion in the docstrings.'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/135',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/135/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/135/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/135/events',
'html_url': 'https://github.com/pysal/segregation/issues/135',
'id': 484299743,
'node_id': 'MDU6SXNzdWU0ODQyOTk3NDM=',
'number': 135,
'title': 'Strange non-corresponding legend on output (Out)when running indexes',
'user': {'login': 'MyrnaSastre',
'id': 30789214,
'node_id': 'MDQ6VXNlcjMwNzg5MjE0',
'avatar_url': 'https://avatars2.githubusercontent.com/u/30789214?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/MyrnaSastre',
'html_url': 'https://github.com/MyrnaSastre',
'followers_url': 'https://api.github.com/users/MyrnaSastre/followers',
'following_url': 'https://api.github.com/users/MyrnaSastre/following{/other_user}',
'gists_url': 'https://api.github.com/users/MyrnaSastre/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/MyrnaSastre/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/MyrnaSastre/subscriptions',
'organizations_url': 'https://api.github.com/users/MyrnaSastre/orgs',
'repos_url': 'https://api.github.com/users/MyrnaSastre/repos',
'events_url': 'https://api.github.com/users/MyrnaSastre/events{/privacy}',
'received_events_url': 'https://api.github.com/users/MyrnaSastre/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 7,
'created_at': '2019-08-23T02:33:24Z',
'updated_at': '2019-08-23T05:13:02Z',
'closed_at': '2019-08-23T05:13:01Z',
'author_association': 'NONE',
'body': 'When running segregation indexes, I get a non-corresponding output legend (Out). It repeats the same output line: "segregation.spatial.spatial_indexes.SpatialDissim" regardless if the index has change... here an example of two different indexes, same Out legend:\r\n<img width="1123" alt="Screen Shot 2019-08-22 at 7 20 42 PM" src="https://user-images.githubusercontent.com/30789214/63562496-adf35780-c512-11e9-9bce-6f1e01986708.png">\r\n\r\n\r\nThis seems to be the default Output line in my local results... in every index...but this is not the case in the examples from the notebook. \r\n\r\nAny ideas as to what can be the problem in my local version? Thanks!!!\r\n\r\n'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/130',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/130/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/130/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/130/events',
'html_url': 'https://github.com/pysal/segregation/pull/130',
'id': 481322655,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA3ODQ0Njg3',
'number': 130,
'title': '[WIP] NA handling',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 3,
'created_at': '2019-08-15T20:23:54Z',
'updated_at': '2019-08-19T22:05:27Z',
'closed_at': '2019-08-19T22:05:27Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/130',
'html_url': 'https://github.com/pysal/segregation/pull/130',
'diff_url': 'https://github.com/pysal/segregation/pull/130.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/130.patch'},
'body': 'This handles https://github.com/pysal/segregation/issues/105 in a conservative way: it raises an informative error whenever the user has missing data in the input columns, but adds a `fillna` useful option if he/she wants to input 0 in these missings values to calculate the indexes.\r\n\r\nAll tests passed.'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/132',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/132/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/132/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/132/events',
'html_url': 'https://github.com/pysal/segregation/issues/132',
'id': 482443915,
'node_id': 'MDU6SXNzdWU0ODI0NDM5MTU=',
'number': 132,
'title': 'Update link for instruction of LTDB_Std_All_fullcount.zip',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-19T17:47:50Z',
'updated_at': '2019-08-19T22:05:12Z',
'closed_at': '2019-08-19T22:05:12Z',
'author_association': 'COLLABORATOR',
'body': 'Currently, we are point to https://github.com/spatialucr/geosnap/tree/master/geosnap/data, but we need to update this.'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/105',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/105/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/105/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/105/events',
'html_url': 'https://github.com/pysal/segregation/issues/105',
'id': 465402981,
'node_id': 'MDU6SXNzdWU0NjU0MDI5ODE=',
'number': 105,
'title': 'handling nans/missing values',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1487805301,
'node_id': 'MDU6TGFiZWwxNDg3ODA1MzAx',
'url': 'https://api.github.com/repos/pysal/segregation/labels/priority:%20high',
'name': 'priority: high',
'color': '006b75',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 12,
'created_at': '2019-07-08T18:38:26Z',
'updated_at': '2019-08-19T22:05:12Z',
'closed_at': '2019-08-19T22:05:12Z',
'author_association': 'MEMBER',
'body': "the following will result in a np.nan value for the `statistic` because `n_nonhsip_black_persons` has NaN values. \r\n\r\n```python\r\ndc = Community(source='ltdb', cbsafips='47900')\r\ndc = dc.tracts.merge(dc.census,left_on='geoid', right_index=True)\r\ndc_sd = SpatialDissim(dc, group_pop_var='n_nonhisp_black_persons', total_pop_var='n_total_pop')\r\n```\r\nWe should either move to more robust numpy operators that handle nans, or check whether there are any present in `group_pop_var` or `total_pop_var` and raise accordingly"},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/131',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/131/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/131/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/131/events',
'html_url': 'https://github.com/pysal/segregation/pull/131',
'id': 482439214,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA4NzEyMTI1',
'number': 131,
'title': 'Raise an informative error when input data have NAs (also update geosnap links)',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 5,
'created_at': '2019-08-19T17:37:14Z',
'updated_at': '2019-08-19T22:05:11Z',
'closed_at': '2019-08-19T22:05:11Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/131',
'html_url': 'https://github.com/pysal/segregation/pull/131',
'diff_url': 'https://github.com/pysal/segregation/pull/131.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/131.patch'},
'body': 'This implements https://github.com/pysal/segregation/pull/130#issuecomment-522137184. So, this is PR is open in favor of https://github.com/pysal/segregation/pull/130.'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/129',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/129/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/129/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/129/events',
'html_url': 'https://github.com/pysal/segregation/issues/129',
'id': 481064895,
'node_id': 'MDU6SXNzdWU0ODEwNjQ4OTU=',
'number': 129,
'title': " ModuleNotFoundError: No module named 'segregation.spatial'",
'user': {'login': 'MyrnaSastre',
'id': 30789214,
'node_id': 'MDQ6VXNlcjMwNzg5MjE0',
'avatar_url': 'https://avatars2.githubusercontent.com/u/30789214?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/MyrnaSastre',
'html_url': 'https://github.com/MyrnaSastre',
'followers_url': 'https://api.github.com/users/MyrnaSastre/followers',
'following_url': 'https://api.github.com/users/MyrnaSastre/following{/other_user}',
'gists_url': 'https://api.github.com/users/MyrnaSastre/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/MyrnaSastre/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/MyrnaSastre/subscriptions',
'organizations_url': 'https://api.github.com/users/MyrnaSastre/orgs',
'repos_url': 'https://api.github.com/users/MyrnaSastre/repos',
'events_url': 'https://api.github.com/users/MyrnaSastre/events{/privacy}',
'received_events_url': 'https://api.github.com/users/MyrnaSastre/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 3,
'created_at': '2019-08-15T09:14:33Z',
'updated_at': '2019-08-19T18:09:13Z',
'closed_at': '2019-08-19T18:09:13Z',
'author_association': 'NONE',
'body': "I'm getting this ModuleNotFoundError: \r\n\r\n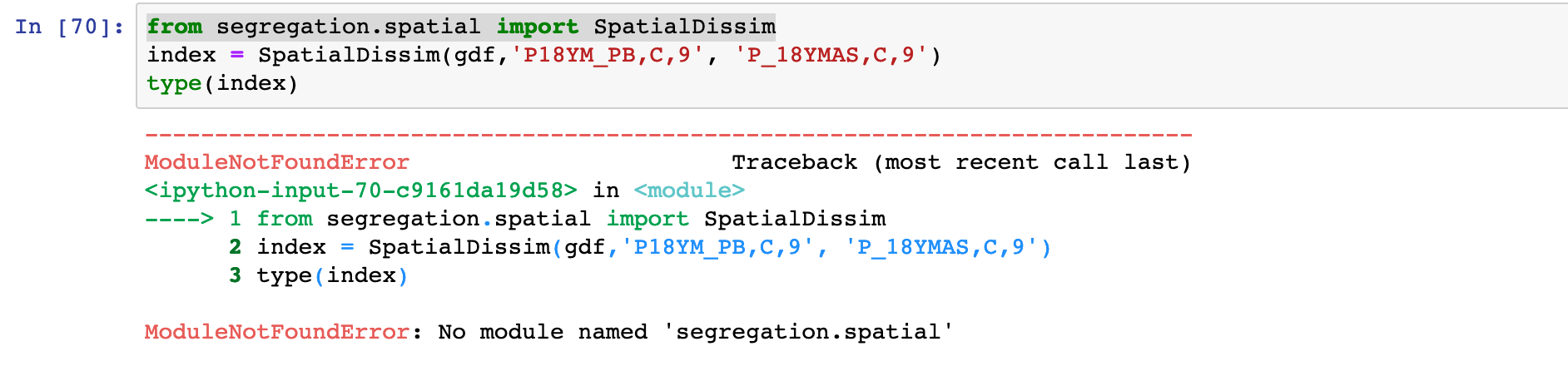\r\n\r\nI have segregation (1.1.1) and PySAL 2.1.0 in my environment. \r\n\r\nAny suggestions? Thanks!"},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/127',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/127/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/127/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/127/events',
'html_url': 'https://github.com/pysal/segregation/issues/127',
'id': 477646434,
'node_id': 'MDU6SXNzdWU0Nzc2NDY0MzQ=',
'number': 127,
'title': 'haversine_distance relies on newer version of scikit-learn',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1487805301,
'node_id': 'MDU6TGFiZWwxNDg3ODA1MzAx',
'url': 'https://api.github.com/repos/pysal/segregation/labels/priority:%20high',
'name': 'priority: high',
'color': '006b75',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-08-07T00:11:36Z',
'updated_at': '2019-08-15T16:08:01Z',
'closed_at': '2019-08-15T16:08:01Z',
'author_association': 'COLLABORATOR',
'body': 'Requirements of segregation should explicitly ask for a version of scikit-learn that has `haversine_distance` distance measures. Otherwise, it will raise an error while importing.'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/128',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/128/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/128/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/128/events',
'html_url': 'https://github.com/pysal/segregation/pull/128',
'id': 479881331,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA2Njg0ODc1',
'number': 128,
'title': 'update scikit-learn requirements',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-12T22:38:26Z',
'updated_at': '2019-08-13T21:00:50Z',
'closed_at': '2019-08-13T21:00:50Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/128',
'html_url': 'https://github.com/pysal/segregation/pull/128',
'diff_url': 'https://github.com/pysal/segregation/pull/128.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/128.patch'},
'body': 'This addresses https://github.com/pysal/segregation/issues/127.'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/126',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/126/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/126/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/126/events',
'html_url': 'https://github.com/pysal/segregation/pull/126',
'id': 476940989,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA0MzY3MDcy',
'number': 126,
'title': 'fix bug of compute_all tests',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-05T16:03:55Z',
'updated_at': '2019-08-05T16:05:54Z',
'closed_at': '2019-08-05T16:04:01Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/126',
'html_url': 'https://github.com/pysal/segregation/pull/126',
'diff_url': 'https://github.com/pysal/segregation/pull/126.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/126.patch'},
'body': "A small bug was spotted in the tests compute_all class of functions in https://github.com/pysal/segregation/commit/93cfb33fba409781e49c00c71c226b82d7645c30. This PR fixes that for the Travis build. I'll just merge this right away since this is just a quick fix."},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/123',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/123/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/123/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/123/events',
'html_url': 'https://github.com/pysal/segregation/issues/123',
'id': 475849040,
'node_id': 'MDU6SXNzdWU0NzU4NDkwNDA=',
'number': 123,
'title': 'Swap to more robust tests that rely on numpy seeds',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 2,
'created_at': '2019-08-01T19:24:44Z',
'updated_at': '2019-08-05T15:37:03Z',
'closed_at': '2019-08-05T15:37:03Z',
'author_association': 'COLLABORATOR',
'body': "Segregation on PySAL started to fail on travis https://travis-ci.org/pysal/pysal/jobs/566597373#L2405\r\n\r\nThis is due to indexes that rely on simulations and, therefore, numpy seeds. Travis now is building using numpy 1.17.0 (released 6 days ago). Some features were changed in the generating process of the seeds as you can read in https://github.com/numpy/numpy/releases.\r\n\r\n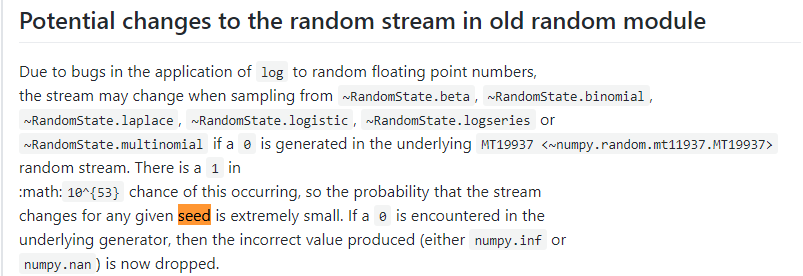\r\n\r\nI upgraded locally my numpy to 1.17.0 (previously it was 1.16.0) and, now, my seed generates the values that match the new tests (7 digit precision).\r\n\r\nSince I don't think it is a good thing the rely on the numpy version for the simulation-based tests... I think I'll increase the degree of tolerance of all tests and rewrite the tests (inference based also will change since they also rely on numpy seeds)."},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/121',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/121/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/121/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/121/events',
'html_url': 'https://github.com/pysal/segregation/pull/121',
'id': 472965524,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzAxMjI1MjMy',
'number': 121,
'title': 'Adds MinMax and SpatialMinMax to documentations/notebooks and compute_all and tweak notebooks',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-07-25T16:41:00Z',
'updated_at': '2019-08-05T15:32:22Z',
'closed_at': '2019-08-05T15:32:22Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/121',
'html_url': 'https://github.com/pysal/segregation/pull/121',
'diff_url': 'https://github.com/pysal/segregation/pull/121.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/121.patch'},
'body': 'This basically adds the MinMax and SpatialMinMax indexes in the documentation (readme, notebooks, compute_all functions, sphinx).\r\n\r\nps.: this PR also makes a small tweak in the spatial notebook running the RelativeConcentration index of the inference wrappers with its fixed value.'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/125',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/125/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/125/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/125/events',
'html_url': 'https://github.com/pysal/segregation/pull/125',
'id': 475919256,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzAzNTc5NDY3',
'number': 125,
'title': 'Change precision of simulated-based tests.',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-08-01T22:40:41Z',
'updated_at': '2019-08-05T15:15:06Z',
'closed_at': '2019-08-05T15:15:06Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/125',
'html_url': 'https://github.com/pysal/segregation/pull/125',
'diff_url': 'https://github.com/pysal/segregation/pull/125.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/125.patch'},
'body': 'It addresses #123.\r\n\r\nIt covers inference, some segregation indexes and inference wrappers.'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/124',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/124/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/124/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/124/events',
'html_url': 'https://github.com/pysal/segregation/pull/124',
'id': 475903284,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzAzNTY2MzUw',
'number': 124,
'title': 'Increase the precision of simulated-based tests.',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-08-01T21:45:58Z',
'updated_at': '2019-08-01T23:19:16Z',
'closed_at': '2019-08-01T21:48:47Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/124',
'html_url': 'https://github.com/pysal/segregation/pull/124',
'diff_url': 'https://github.com/pysal/segregation/pull/124.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/124.patch'},
'body': 'It addresses https://github.com/pysal/segregation/issues/123.\r\n\r\nIt covers inference, some segregation indexes and inference wrappers.'},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/86',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/86/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/86/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/86/events',
'html_url': 'https://github.com/pysal/segregation/issues/86',
'id': 462820129,
'node_id': 'MDU6SXNzdWU0NjI4MjAxMjk=',
'number': 86,
'title': '[ENH] extend inference framework to multigroup indices',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1167292080,
'node_id': 'MDU6TGFiZWwxMTY3MjkyMDgw',
'url': 'https://api.github.com/repos/pysal/segregation/labels/enhancement',
'name': 'enhancement',
'color': 'a2eeef',
'default': True,
'description': 'New feature or request'}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 4,
'created_at': '2019-07-01T17:07:13Z',
'updated_at': '2019-07-31T21:04:00Z',
'closed_at': '2019-07-31T21:04:00Z',
'author_association': 'MEMBER',
'body': ''},
{'url': 'https://api.github.com/repos/pysal/segregation/issues/122',
'repository_url': 'https://api.github.com/repos/pysal/segregation',
'labels_url': 'https://api.github.com/repos/pysal/segregation/issues/122/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/segregation/issues/122/comments',
'events_url': 'https://api.github.com/repos/pysal/segregation/issues/122/events',
'html_url': 'https://github.com/pysal/segregation/pull/122',
'id': 474863944,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzAyNzMwODAy',
'number': 122,
'title': 'Adds inference wrappers for multigroup indexes (Single and Two)',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-07-30T23:18:28Z',
'updated_at': '2019-07-31T21:03:32Z',
'closed_at': '2019-07-31T21:03:32Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/segregation/pulls/122',
'html_url': 'https://github.com/pysal/segregation/pull/122',
'diff_url': 'https://github.com/pysal/segregation/pull/122.diff',
'patch_url': 'https://github.com/pysal/segregation/pull/122.patch'},
'body': 'This PR adds the "evenness" and "bootstrap" approach for MultiGroup indexes as raised in https://github.com/pysal/segregation/issues/86. Some of the formattings were changed as I thought It was easier to spot which lines to change in the code and how to properly construct the null hypothesis.\r\n\r\nI assumed that the evenness approach is simply the generalization of the single group approach, as every unit had a fixed vector of probability of allocating for each subgroup. However, the difference is that now the simulation is a multinomial distribution in the tract level. So, we need to simulate many multinomial distributions and I achieved that using a `map` function.\r\n\r\nThis is still a WIP since I\'d like to add the `random_label` approach for comparing two Multigroup Measures.\r\n\r\nEdit: I added the MultiGroup case for two indexes, so removed the WIP of the name of this PR.'}],
'spaghetti': [{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/394',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/394/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/394/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/394/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/394',
'id': 555002224,
'node_id': 'MDU6SXNzdWU1NTUwMDIyMjQ=',
'number': 394,
'title': '[DOC] paths docstring missing',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 0,
'created_at': '2020-01-24T23:30:28Z',
'updated_at': '2020-01-25T01:28:11Z',
'closed_at': '2020-01-25T01:28:11Z',
'author_association': 'MEMBER',
'body': 'Description of [`paths`](https://github.com/pysal/spaghetti/blob/d87011a5722a332604bb8cb5ef1e233216312ced/spaghetti/network.py#L2805) missing from docstring in [`spaghetti.Network.element_as_gdf()`](https://github.com/pysal/spaghetti/blob/d87011a5722a332604bb8cb5ef1e233216312ced/spaghetti/network.py#L2734).'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/395',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/395/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/395/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/395/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/395',
'id': 555019140,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY3MDY5Mzcy',
'number': 395,
'title': 'correcting routes doc',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-25T00:53:48Z',
'updated_at': '2020-01-25T01:25:05Z',
'closed_at': '2020-01-25T01:25:01Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/395',
'html_url': 'https://github.com/pysal/spaghetti/pull/395',
'diff_url': 'https://github.com/pysal/spaghetti/pull/395.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/395.patch'},
'body': 'This PR:\r\n* addresses #394 \r\n* adds minor formatting improvement to shortest path notebook'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/393',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/393/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/393/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/393/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/393',
'id': 554546623,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY2NjgxMDUw',
'number': 393,
'title': '[ENH] add back `build` badge',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-24T05:05:17Z',
'updated_at': '2020-01-24T05:22:12Z',
'closed_at': '2020-01-24T05:22:08Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/393',
'html_url': 'https://github.com/pysal/spaghetti/pull/393',
'diff_url': 'https://github.com/pysal/spaghetti/pull/393.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/393.patch'},
'body': 'This PR adds the Travis CI\xa0`build` badge back into `README.md`.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/387',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/387/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/387/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/387/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/387',
'id': 553953689,
'node_id': 'MDU6SXNzdWU1NTM5NTM2ODk=',
'number': 387,
'title': '[BUG] Stale links on pysal.org/spaghetti',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118413,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTM=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-23T05:56:28Z',
'updated_at': '2020-01-23T15:59:42Z',
'closed_at': '2020-01-23T15:59:42Z',
'author_association': 'MEMBER',
'body': '* all three links to notebooks on the homepage\r\n* connected components notebook link on tutorial page'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/391',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/391/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/391/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/391/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/391',
'id': 553979014,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY2MjE0OTkx',
'number': 391,
'title': 'Rebuild docs',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-23T07:17:31Z',
'updated_at': '2020-01-23T15:58:16Z',
'closed_at': '2020-01-23T15:58:13Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/391',
'html_url': 'https://github.com/pysal/spaghetti/pull/391',
'diff_url': 'https://github.com/pysal/spaghetti/pull/391.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/391.patch'},
'body': 'Rebuilding docs for resolution to #387 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/390',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/390/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/390/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/390/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/390',
'id': 553969848,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY2MjA3MzQ1',
'number': 390,
'title': 'force read-add conn-comp notebook',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2020-01-23T06:49:46Z',
'updated_at': '2020-01-23T07:01:21Z',
'closed_at': '2020-01-23T07:00:12Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/390',
'html_url': 'https://github.com/pysal/spaghetti/pull/390',
'diff_url': 'https://github.com/pysal/spaghetti/pull/390.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/390.patch'},
'body': '(Hopefully) finally resolving #387 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/389',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/389/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/389/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/389/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/389',
'id': 553964962,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY2MjAzMjU3',
'number': 389,
'title': 'repushing missing notebooks',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118413,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTM=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2020-01-23T06:33:50Z',
'updated_at': '2020-01-23T06:43:02Z',
'closed_at': '2020-01-23T06:42:59Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/389',
'html_url': 'https://github.com/pysal/spaghetti/pull/389',
'diff_url': 'https://github.com/pysal/spaghetti/pull/389.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/389.patch'},
'body': 'This PR addresses the issue of missing notebooks, an underlying part of #387 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/388',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/388/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/388/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/388/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/388',
'id': 553958832,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY2MTk4MDUy',
'number': 388,
'title': 'Stale links',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118413,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTM=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2020-01-23T06:14:03Z',
'updated_at': '2020-01-23T06:24:19Z',
'closed_at': '2020-01-23T06:24:16Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/388',
'html_url': 'https://github.com/pysal/spaghetti/pull/388',
'diff_url': 'https://github.com/pysal/spaghetti/pull/388.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/388.patch'},
'body': 'This PR address the stale links issue raise din #387 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/380',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/380/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/380/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/380/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/380',
'id': 550878765,
'node_id': 'MDU6SXNzdWU1NTA4Nzg3NjU=',
'number': 380,
'title': '[ENH] shortest-path extraction',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 0,
'created_at': '2020-01-16T15:24:48Z',
'updated_at': '2020-01-20T21:36:42Z',
'closed_at': '2020-01-20T21:36:42Z',
'author_association': 'MEMBER',
'body': 'Need to add functionality for\r\n* shortest-path extraction as `libpysal.cg.Chain` objects\r\n* option for shortest-path extraction as `shapely.geoemtry.LineString` objects in a `geopandas.GeoDataFrame`\r\n\r\nRelated to: #217, #377\r\nPrerequisite for: #292, #374 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/385',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/385/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/385/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/385/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/385',
'id': 552065324,
'node_id': 'MDU6SXNzdWU1NTIwNjUzMjQ=',
'number': 385,
'title': '[ENH] regular lattice generator ',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-20T04:53:03Z',
'updated_at': '2020-01-20T21:36:33Z',
'closed_at': '2020-01-20T21:36:33Z',
'author_association': 'MEMBER',
'body': 'Add functionality for a regular lattice generator that can be used for testing/demonstration purposed.\r\n\r\n* [x] function\r\n* [x] docstring\r\n* [x] example\r\n* [x] tests\r\n* [x] rebuild docs'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/382',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/382/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/382/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/382/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/382',
'id': 551205645,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzYzOTY5MzAw',
'number': 382,
'title': '[WIP][ENH] addressing shortest path extract',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1674941943,
'node_id': 'MDU6TGFiZWwxNjc0OTQxOTQz',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/WIP',
'name': 'WIP',
'color': '3913ad',
'default': False,
'description': ''},
{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 4,
'created_at': '2020-01-17T05:22:03Z',
'updated_at': '2020-01-20T21:34:40Z',
'closed_at': '2020-01-20T21:34:36Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/382',
'html_url': 'https://github.com/pysal/spaghetti/pull/382',
'diff_url': 'https://github.com/pysal/spaghetti/pull/382.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/382.patch'},
'body': 'This PR addresses #380 \r\n\r\n* [x] `spaghetti.Network.shortests_paths()`\r\n * [x] method\r\n * [x] docstring\r\n * [x] tests\r\n * [x] example/notebook\r\n* [x] `spaghetti.element_as_gdf(new, routes=paths)`\r\n * [x] function\r\n * [x] docstring\r\n * [x] tests\r\n * [x] example/notebook\r\n* [x] rebuild docs'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/384',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/384/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/384/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/384/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/384',
'id': 551961056,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY0NTYyMTkx',
'number': 384,
'title': 'mention the pysal/notebook project',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2020-01-19T18:19:11Z',
'updated_at': '2020-01-19T18:28:15Z',
'closed_at': '2020-01-19T18:28:10Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/384',
'html_url': 'https://github.com/pysal/spaghetti/pull/384',
'diff_url': 'https://github.com/pysal/spaghetti/pull/384.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/384.patch'},
'body': 'This PR adds a link to the pysal/notebook project, based that in [`splot`](https://github.com/pysal/splot/pull/97/commits/c0ae8cd4fa665c24132c87afe7ef7ffd739a987e).'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/383',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/383/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/383/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/383/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/383',
'id': 551791516,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY0NDQyMDMx',
'number': 383,
'title': 'point pattern --> network error message',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1748719359,
'node_id': 'MDU6TGFiZWwxNzQ4NzE5MzU5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/rough%20edge',
'name': 'rough edge',
'color': 'ef6040',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-18T16:32:31Z',
'updated_at': '2020-01-18T16:40:17Z',
'closed_at': '2020-01-18T16:40:13Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/383',
'html_url': 'https://github.com/pysal/spaghetti/pull/383',
'diff_url': 'https://github.com/pysal/spaghetti/pull/383.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/383.patch'},
'body': 'This PR fixes an [incorrect error message](https://github.com/pysal/spaghetti/blob/6f216e5b4d338819eb61e6c133e16de3c55c009f/spaghetti/network.py#L416) in `network.py`.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/381',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/381/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/381/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/381/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/381',
'id': 551198816,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzYzOTYzNTg2',
'number': 381,
'title': '[ENH] connected components demo notebook',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1763805303,
'node_id': 'MDU6TGFiZWwxNzYzODA1MzAz',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/binders',
'name': 'binders',
'color': '3897b7',
'default': False,
'description': ''},
{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2020-01-17T04:55:44Z',
'updated_at': '2020-01-17T05:09:59Z',
'closed_at': '2020-01-17T05:09:55Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/381',
'html_url': 'https://github.com/pysal/spaghetti/pull/381',
'diff_url': 'https://github.com/pysal/spaghetti/pull/381.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/381.patch'},
'body': 'This PR adds a notebook highlighting the usage of connected components in the network.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/379',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/379/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/379/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/379/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/379',
'id': 549599708,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzYyNjYxMDkx',
'number': 379,
'title': 'updating README.md',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-14T14:21:21Z',
'updated_at': '2020-01-14T14:34:51Z',
'closed_at': '2020-01-14T14:34:47Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/379',
'html_url': 'https://github.com/pysal/spaghetti/pull/379',
'diff_url': 'https://github.com/pysal/spaghetti/pull/379.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/379.patch'},
'body': 'Adding to BibTeX section info in `README.md`'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/378',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/378/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/378/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/378/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/378',
'id': 548620150,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzYxODY2MTc1',
'number': 378,
'title': 'data type testing',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1783401156,
'node_id': 'MDU6TGFiZWwxNzgzNDAxMTU2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/testing',
'name': 'testing',
'color': 'e04a5b',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-12T20:09:10Z',
'updated_at': '2020-01-12T20:18:05Z',
'closed_at': '2020-01-12T20:18:00Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/378',
'html_url': 'https://github.com/pysal/spaghetti/pull/378',
'diff_url': 'https://github.com/pysal/spaghetti/pull/378.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/378.patch'},
'body': "This PR adds testing for `tuple` and `numpy.array` containers when instantiating a `spaghetti.Network` object, and testing for `tuple` containers when instantiating a `spaghetti.PointPattern` object.\r\n\r\nSupport for instantiating a `spaghetti.PointPattern` object of `libpysal.cg.Point`s stored in a `numpy.array` can't be supported at this time due to `numpy` overwriting the `libpysal.cg.Point` datatype into simple arrays of floats."},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/217',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/217/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/217/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/217/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/217',
'id': 391395790,
'node_id': 'MDU6SXNzdWUzOTEzOTU3OTA=',
'number': 217,
'title': 'spaghetti should handle native cg.Point/Chain objects',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2018-12-15T17:18:52Z',
'updated_at': '2020-01-16T15:25:40Z',
'closed_at': '2020-01-12T04:06:59Z',
'author_association': 'MEMBER',
'body': 'Currently, input for instantiating a [`network.Network`](https://github.com/pysal/spaghetti/blob/c89bd3ee7d87e55337fbbd3dfbff6f649073de77/spaghetti/network.py#L19) and [`network.PointPattern`](https://github.com/pysal/spaghetti/blob/c89bd3ee7d87e55337fbbd3dfbff6f649073de77/spaghetti/network.py#L2213) can be accomplished with either a [`.shp` file](https://github.com/pysal/spaghetti/blob/c89bd3ee7d87e55337fbbd3dfbff6f649073de77/spaghetti/network.py#L344) or a [`geopandas.GeoDataFrame`](https://github.com/pysal/spaghetti/blob/c89bd3ee7d87e55337fbbd3dfbff6f649073de77/spaghetti/network.py#L346). However, passing in native [`pysal.cg`](https://github.com/pysal/libpysal/tree/master/libpysal/cg) objects is not an option. As `spaghetti` is a member of the [`pysal` federation](http://pysal.org/docs.html), this functionality should be available.\r\n\r\n - [x] `network.Network` accepts `cg.Chain` objects for instantiation\r\n - [x] `network.PointPattern` accepts `cg.Point` objects for instantiation\r\n\r\n-------------------\r\n\r\n***Tests***\r\n- [x] tests/test_network.py -- test_network_from_cg(self)\r\n - [x] cg test against known\r\n - [x] shp against cg\r\n- [x] tests/test_network_api.py -- test_network_from_cg(self)\r\n - [x] cg test against known\r\n - [x] shp against cg\r\n\r\n------------------\r\n\r\n***Docs***\r\n - [x] re-run docs'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/377',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/377/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/377/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/377/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/377',
'id': 548517619,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzYxNzkxNzYx',
'number': 377,
'title': 'Native geometries',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2020-01-12T03:57:44Z',
'updated_at': '2020-01-16T15:25:28Z',
'closed_at': '2020-01-12T04:06:34Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/377',
'html_url': 'https://github.com/pysal/spaghetti/pull/377',
'diff_url': 'https://github.com/pysal/spaghetti/pull/377.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/377.patch'},
'body': 'This PR addresses #217 and includes:\r\n * incorporates the handling of `libpysal.cg` geometries for\r\n * network creation\r\n * point pattern snapping\r\n * add a reference for [`snkit`](https://github.com/tomalrussell/snkit/tree/v1.6.0)'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/376',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/376/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/376/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/376/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/376',
'id': 548101969,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzYxNDY4NTc5',
'number': 376,
'title': 'adding CoC link to README.md',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-10T14:13:32Z',
'updated_at': '2020-01-10T14:25:17Z',
'closed_at': '2020-01-10T14:25:13Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/376',
'html_url': 'https://github.com/pysal/spaghetti/pull/376',
'diff_url': 'https://github.com/pysal/spaghetti/pull/376.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/376.patch'},
'body': 'This PR adds Code of Conduct information from PySAL to `README.md`.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/375',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/375/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/375/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/375/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/375',
'id': 546333060,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzYwMDMwMzU3',
'number': 375,
'title': 'update CHANGELOG.md format',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-07T15:03:37Z',
'updated_at': '2020-01-07T15:47:06Z',
'closed_at': '2020-01-07T15:47:02Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/375',
'html_url': 'https://github.com/pysal/spaghetti/pull/375',
'diff_url': 'https://github.com/pysal/spaghetti/pull/375.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/375.patch'},
'body': 'This PR improves the formatting and consistency of [`CHANGELOG.md`](https://github.com/pysal/spaghetti/blob/master/CHANGELOG.md).'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/346',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/346/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/346/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/346/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/346',
'id': 542199074,
'node_id': 'MDU6SXNzdWU1NDIxOTkwNzQ=',
'number': 346,
'title': 'all reqs found in requirements*.txt necessary?',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''},
{'id': 1748719359,
'node_id': 'MDU6TGFiZWwxNzQ4NzE5MzU5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/rough%20edge',
'name': 'rough edge',
'color': 'ef6040',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/3',
'html_url': 'https://github.com/pysal/spaghetti/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/3/labels',
'id': 4469157,
'node_id': 'MDk6TWlsZXN0b25lNDQ2OTE1Nw==',
'number': 3,
'title': 'future release',
'description': None,
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 14,
'closed_issues': 6,
'state': 'open',
'created_at': '2019-07-05T22:51:58Z',
'updated_at': '2020-01-06T18:59:07Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2019-12-24T19:34:09Z',
'updated_at': '2020-01-06T18:17:32Z',
'closed_at': '2020-01-06T18:17:32Z',
'author_association': 'MEMBER',
'body': 'Review and prune all unused/redundant requirements found in the `requirements*.txt` files.\r\n\r\n - [x] [`requirements.txt`](https://github.com/pysal/spaghetti/blob/master/requirements.txt)\r\n - [x] [`requirements_dev.txt`](https://github.com/pysal/spaghetti/blob/master/requirements_dev.txt)\r\n - [x] [`requirements_docs.txt`](https://github.com/pysal/spaghetti/blob/master/requirements_docs.txt)\r\n - [x] [`requirements_plus.txt`](https://github.com/pysal/spaghetti/blob/master/requirements_plus.txt)\r\n - [x] [`requirements_tests.txt`](https://github.com/pysal/spaghetti/blob/master/requirements_tests.txt)'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/320',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/320/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/320/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/320/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/320',
'id': 507394603,
'node_id': 'MDU6SXNzdWU1MDczOTQ2MDM=',
'number': 320,
'title': '[ENH] explore overlapping "nearest point" calculation — DRY',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1045274469,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NDY5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-mid',
'name': 'priority-mid',
'color': 'e2be51',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/3',
'html_url': 'https://github.com/pysal/spaghetti/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/3/labels',
'id': 4469157,
'node_id': 'MDk6TWlsZXN0b25lNDQ2OTE1Nw==',
'number': 3,
'title': 'future release',
'description': None,
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 14,
'closed_issues': 6,
'state': 'open',
'created_at': '2019-07-05T22:51:58Z',
'updated_at': '2020-01-06T18:59:07Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2019-10-15T17:55:48Z',
'updated_at': '2020-01-06T18:15:29Z',
'closed_at': '2020-01-06T18:15:29Z',
'author_association': 'MEMBER',
'body': 'explore overlapping "nearest point along a line" calculation comparing the two following:\r\n- [`spaghetti.util.squared_distance_point_link()`](https://github.com/pysal/spaghetti/blob/cf7908bae2d6686ec7d72b9e8ae0e92d035bd2a3/spaghetti/util.py#L317-L383) \r\n- [`libpysal.cg.standalone.get_segment_point_dist()`](https://github.com/pysal/libpysal/blob/574192fca6fc2640f05d9617a80cb2637e00f93b/libpysal/cg/standalone.py#L491-L553) '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/372',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/372/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/372/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/372/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/372',
'id': 545339679,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjUyMzMw',
'number': 372,
'title': 'altering reference format in docs',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-04T22:23:16Z',
'updated_at': '2020-01-05T22:18:37Z',
'closed_at': '2020-01-04T22:57:55Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/372',
'html_url': 'https://github.com/pysal/spaghetti/pull/372',
'diff_url': 'https://github.com/pysal/spaghetti/pull/372.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/372.patch'},
'body': 'This PR alters the format of the reference keys in https://pysal.org/spaghetti/references.html'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/369',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/369/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/369/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/369/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/369',
'id': 545233296,
'node_id': 'MDU6SXNzdWU1NDUyMzMyOTY=',
'number': 369,
'title': 'Clear instructions for black/pre-commit for contributing',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''},
{'id': 1748719359,
'node_id': 'MDU6TGFiZWwxNzQ4NzE5MzU5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/rough%20edge',
'name': 'rough edge',
'color': 'ef6040',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 0,
'created_at': '2020-01-04T03:07:09Z',
'updated_at': '2020-01-04T16:19:03Z',
'closed_at': '2020-01-04T16:19:03Z',
'author_association': 'MEMBER',
'body': '* [x] Add `pre-commit` to [`requirements_dev.txt`](https://github.com/pysal/spaghetti/blob/master/requirements_dev.txt)\r\n* [x] give explicit instructions regarding the install/execution of pre-commit\r\n * great resource [here](https://ljvmiranda921.github.io/notebook/2018/06/21/precommits-using-black-and-flake8/)\r\n* [x] add instructions in [`.github/CONTRIBUTING.md`](https://github.com/pysal/spaghetti/blob/master/.github/CONTRIBUTING.md)\r\n* [x] link [`README.md`](https://github.com/pysal/spaghetti/blob/master/.github/CONTRIBUTING.md) to [`.github/CONTRIBUTING.md`](https://github.com/pysal/spaghetti/blob/master/README.md)\r\n\r\n\r\nSee also #333 \r\n'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/371',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/371/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/371/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/371/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/371',
'id': 545290970,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MjE3OTg3',
'number': 371,
'title': 'Contrib precommit',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None},
{'id': 1768208407,
'node_id': 'MDU6TGFiZWwxNzY4MjA4NDA3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/requirements',
'name': 'requirements',
'color': 'd6c724',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-04T14:26:50Z',
'updated_at': '2020-01-04T16:16:04Z',
'closed_at': '2020-01-04T16:15:58Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/371',
'html_url': 'https://github.com/pysal/spaghetti/pull/371',
'diff_url': 'https://github.com/pysal/spaghetti/pull/371.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/371.patch'},
'body': 'This PR aims to solve:\r\n * #346 \r\n * #369 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/351',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/351/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/351/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/351/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/351',
'id': 544288371,
'node_id': 'MDU6SXNzdWU1NDQyODgzNzE=',
'number': 351,
'title': 'alldistances vs. distancematrix?',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 650035276,
'node_id': 'MDU6TGFiZWw2NTAwMzUyNzY=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/idea/suggestion/future%20work',
'name': 'idea/suggestion/future work',
'color': '006b75',
'default': False,
'description': None},
{'id': 1045274469,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NDY5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-mid',
'name': 'priority-mid',
'color': 'e2be51',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 3,
'created_at': '2019-12-31T23:36:49Z',
'updated_at': '2020-01-04T04:02:13Z',
'closed_at': '2020-01-04T04:02:13Z',
'author_association': 'MEMBER',
'body': 'Refactor the storage of the `spaghetti.Network.alldistances` and `spaghetti.Network.distancematrix` attributes. These currently are redundant and can be combined into one attribute.\r\n\r\nsee: [here](https://github.com/pysal/spaghetti/blob/3421d64d9bfd8c21b57d28f7342d5dc097c08619/spaghetti/network.py#L1428-L1429) and [here](https://github.com/pysal/spaghetti/blob/3421d64d9bfd8c21b57d28f7342d5dc097c08619/spaghetti/network.py#L1466-L1467)\r\n'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/370',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/370/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/370/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/370/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/370',
'id': 545235845,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MTc5MTY4',
'number': 370,
'title': 'distance matrix and tree storage',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-04T03:39:09Z',
'updated_at': '2020-01-04T04:00:17Z',
'closed_at': '2020-01-04T04:00:12Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/370',
'html_url': 'https://github.com/pysal/spaghetti/pull/370',
'diff_url': 'https://github.com/pysal/spaghetti/pull/370.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/370.patch'},
'body': 'This PR addresses #351 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/367',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/367/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/367/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/367/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/367',
'id': 545152575,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MTE1MDIy',
'number': 367,
'title': 'update readme',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2020-01-03T20:35:33Z',
'updated_at': '2020-01-03T20:56:19Z',
'closed_at': '2020-01-03T20:56:15Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/367',
'html_url': 'https://github.com/pysal/spaghetti/pull/367',
'diff_url': 'https://github.com/pysal/spaghetti/pull/367.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/367.patch'},
'body': 'updating format of readme.md'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/366',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/366/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/366/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/366/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/366',
'id': 544785777,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4ODI0NDQw',
'number': 366,
'title': 'Adding citations for facility location notebook',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-02T23:08:51Z',
'updated_at': '2020-01-02T23:17:35Z',
'closed_at': '2020-01-02T23:17:31Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/366',
'html_url': 'https://github.com/pysal/spaghetti/pull/366',
'diff_url': 'https://github.com/pysal/spaghetti/pull/366.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/366.patch'},
'body': 'This PR add a complement of citations for the [facility location notebook](https://pysal.org/spaghetti/tutorial.html)'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/340',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/340/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/340/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/340/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/340',
'id': 539842532,
'node_id': 'MDU6SXNzdWU1Mzk4NDI1MzI=',
'number': 340,
'title': 'v1.4 release checklist',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1674941943,
'node_id': 'MDU6TGFiZWwxNjc0OTQxOTQz',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/WIP',
'name': 'WIP',
'color': '3913ad',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''},
{'id': 1371649050,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MDUw',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/version',
'name': 'version',
'color': 'c5def5',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 0,
'created_at': '2019-12-18T18:21:58Z',
'updated_at': '2020-01-02T19:43:28Z',
'closed_at': '2020-01-02T19:43:28Z',
'author_association': 'MEMBER',
'body': '#### wrap up work\r\n* [x] close all issues/PRs related to `v1.4` or move to +1/`v2.0` release\r\n * [x] TSP notebook #330 (#342)/ #110 / #100 \r\n * [x] Use_case-tsp.ipynb\r\n * punt to `v2.0`\r\n * [x] figure out sphinx issue or punt to next release #339, #342 \r\n* [x] review/rename/rerun notebooks\r\n * [x] remove/assimilate [Snapping_Demonstration.ipynb](https://github.com/pysal/spaghetti/blob/master/notebooks/Snapping_Demonstration.ipynb)\r\n * [x] rename ~~[Spaghetti_Pointpatterns_Empirical.ipynb](https://github.com/pysal/spaghetti/blob/master/notebooks/Spaghetti_Pointpatterns_Empirical.ipynb)~~ --> Basic_spaghetti_tutorial.ipynb\r\n * [x] rename ~~[Network_Usage.ipynb](https://github.com/pysal/spaghetti/blob/master/notebooks/Network_Usage.ipynb)~~ --> Advanced_spaghetti_tutorial.ipynb\r\n * [x] rename ~~[Facility_Location.ipynb](https://github.com/pysal/spaghetti/blob/master/notebooks/Facility_Location.ipynb)~~ --> Use_case-facility_location.ipynb\r\n * [x] uniform notebook layout\r\n * [x] standardize font\r\n * [x] disclaimer/citation\r\n * [x] Title/subtitle/author\r\n * [x] explanation/structure\r\n * [x] load watermark / system watermark\r\n * [x] notebook requirements\r\n * [x] (uniform) import structure\r\n * [x] watermark -iv\r\n * [x] nb_black formatting for cells\r\n * [x] update notebook links in `README.md`\r\n * [x] binderize notebooks in docs (see [`giddy`](https://giddy.readthedocs.io/en/latest/tutorial.html) for example)(also #78)\r\n* [x] review `README.md` for references to version\r\n * [x] ~~python~~ --> Python\r\n\r\n---------------------------------------------------------------\r\n#### release `v1.4.0`\r\n* [x] bump version in `__init__`\r\n* [x] rerun `docsrc`\r\n* [x] update CHANGELOG (automatic) **if needed**\r\n* [x] release on `github`\r\n* [x] release on `pypi`\r\n* [x] release on `conda-forge`\r\n * [x] set [`sha256`](https://github.com/conda-forge/spaghetti-feedstock/blob/9839400d2564d17c404e6aff626388c881aa6a13/recipe/meta.yaml#L10) as a variable in the feedstock recipe (as is done in [`watermark-feedstock`](https://github.com/conda-forge/watermark-feedstock/blob/047b3788fb3076b63700cead651525ca226d5511/recipe/meta.yaml#L3)).\r\n * [x] update [`doc_url`](https://github.com/conda-forge/spaghetti-feedstock/blob/9839400d2564d17c404e6aff626388c881aa6a13/recipe/meta.yaml#L46) in the feedstock recipe\r\n * [x] update [`home`](https://github.com/conda-forge/spaghetti-feedstock/blob/9839400d2564d17c404e6aff626388c881aa6a13/recipe/meta.yaml#L34) in the feedstock recipe\r\n * [x] update [`description`](https://github.com/conda-forge/spaghetti-feedstock/blob/9839400d2564d17c404e6aff626388c881aa6a13/recipe/meta.yaml#L39) in the feedstock recipe\r\n* [ ] ~~update CHANGELOG (manual)~~\r\n---------------------------------------------------------------\r\n#### ~~announce `v1.4.0`~~ save announcement for `v1.4.1` #365 \r\n* [ ] ~~announce on `pysal/pysal.github.io`~~\r\n* [ ] ~~announce on Twitter/LinkedIn/etc.~~'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/364',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/364/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/364/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/364/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/364',
'id': 544623119,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4Njk1MTMw',
'number': 364,
'title': 'updating README.md',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2020-01-02T15:25:22Z',
'updated_at': '2020-01-02T15:42:40Z',
'closed_at': '2020-01-02T15:42:36Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/364',
'html_url': 'https://github.com/pysal/spaghetti/pull/364',
'diff_url': 'https://github.com/pysal/spaghetti/pull/364.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/364.patch'},
'body': 'This PR updates some information on README.md and makes some cosmetic changes.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/333',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/333/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/333/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/333/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/333',
'id': 526616427,
'node_id': 'MDU6SXNzdWU1MjY2MTY0Mjc=',
'number': 333,
'title': 'add pre-commit black for PRs?',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1487221050,
'node_id': 'MDU6TGFiZWwxNDg3MjIxMDUw',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/discussion',
'name': 'discussion',
'color': '1092ba',
'default': False,
'description': ''},
{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1045274469,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NDY5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-mid',
'name': 'priority-mid',
'color': 'e2be51',
'default': False,
'description': ''},
{'id': 1432773648,
'node_id': 'MDU6TGFiZWwxNDMyNzczNjQ4',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/travis%20ci',
'name': 'travis ci',
'color': 'c2f23e',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/3',
'html_url': 'https://github.com/pysal/spaghetti/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/3/labels',
'id': 4469157,
'node_id': 'MDk6TWlsZXN0b25lNDQ2OTE1Nw==',
'number': 3,
'title': 'future release',
'description': None,
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 14,
'closed_issues': 6,
'state': 'open',
'created_at': '2019-07-05T22:51:58Z',
'updated_at': '2020-01-06T18:59:07Z',
'due_on': None,
'closed_at': None},
'comments': 0,
'created_at': '2019-11-21T14:06:20Z',
'updated_at': '2020-01-02T02:29:06Z',
'closed_at': '2020-01-02T02:29:06Z',
'author_association': 'MEMBER',
'body': 'Implement updated workflow for code formatting with pre-commits using black?\r\n\r\nSee here — [precommits-using-black-and-flake8](https://ljvmiranda921.github.io/notebook/2018/06/21/precommits-using-black-and-flake8/)'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/363',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/363/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/363/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/363/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/363',
'id': 544425837,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4NTM3MDAz',
'number': 363,
'title': 'trying out the black pre-commit hook',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-02T02:17:29Z',
'updated_at': '2020-01-02T02:27:56Z',
'closed_at': '2020-01-02T02:27:53Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/363',
'html_url': 'https://github.com/pysal/spaghetti/pull/363',
'diff_url': 'https://github.com/pysal/spaghetti/pull/363.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/363.patch'},
'body': 'Addressing #333 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/362',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/362/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/362/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/362/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/362',
'id': 544424434,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4NTM1OTc1',
'number': 362,
'title': 'adding descartes to environment.yml',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1763805303,
'node_id': 'MDU6TGFiZWwxNzYzODA1MzAz',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/binders',
'name': 'binders',
'color': '3897b7',
'default': False,
'description': ''},
{'id': 584118413,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTM=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-02T02:05:39Z',
'updated_at': '2020-01-02T02:09:51Z',
'closed_at': '2020-01-02T02:06:40Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/362',
'html_url': 'https://github.com/pysal/spaghetti/pull/362',
'diff_url': 'https://github.com/pysal/spaghetti/pull/362.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/362.patch'},
'body': 'adding descartes to `environment.yml`'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/361',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/361/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/361/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/361/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/361',
'id': 544420028,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4NTMyNzMz',
'number': 361,
'title': 'Attempt binder3',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-02T01:21:23Z',
'updated_at': '2020-01-02T01:43:27Z',
'closed_at': '2020-01-02T01:43:22Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/361',
'html_url': 'https://github.com/pysal/spaghetti/pull/361',
'diff_url': 'https://github.com/pysal/spaghetti/pull/361.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/361.patch'},
'body': "This PR attempts to solve the binder launch failures (yet again #357 #358).\r\n\r\nCurrent failure when launching binder.\r\n\r\n```\r\nWaiting for build to start...\r\nPicked Git content provider.\r\nCloning into '/tmp/repo2dockerc9c2cwd3'...\r\nHEAD is now at 8e29a4f Merge pull request #358 from jGaboardi/attempt_binder2\r\nError during build: list index out of range\r\n```"},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/360',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/360/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/360/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/360/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/360',
'id': 544410012,
'node_id': 'MDU6SXNzdWU1NDQ0MTAwMTI=',
'number': 360,
'title': 'need readthedocs.yml?',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-01T23:23:29Z',
'updated_at': '2020-01-01T23:39:28Z',
'closed_at': '2020-01-01T23:39:28Z',
'author_association': 'MEMBER',
'body': 'Since moving off ReadTheDocs, there is no need to keep [`readthedocs.yml`](https://github.com/pysal/spaghetti/blob/master/readthedocs.yml).'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/359',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/359/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/359/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/359/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/359',
'id': 544409634,
'node_id': 'MDU6SXNzdWU1NDQ0MDk2MzQ=',
'number': 359,
'title': 'need all tarball tests?',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1432773648,
'node_id': 'MDU6TGFiZWwxNDMyNzczNjQ4',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/travis%20ci',
'name': 'travis ci',
'color': 'c2f23e',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-01T23:19:04Z',
'updated_at': '2020-01-01T23:39:20Z',
'closed_at': '2020-01-01T23:39:20Z',
'author_association': 'MEMBER',
'body': 'Currently we are performing [four `tarball` tests](https://github.com/pysal/spaghetti/blob/master/.travis.yml). This can probably be pruned down to one.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/358',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/358/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/358/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/358/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/358',
'id': 544409065,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4NTI1MTE1',
'number': 358,
'title': 'Attempt binder2',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2020-01-01T23:12:35Z',
'updated_at': '2020-01-01T23:38:27Z',
'closed_at': '2020-01-01T23:38:24Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/358',
'html_url': 'https://github.com/pysal/spaghetti/pull/358',
'diff_url': 'https://github.com/pysal/spaghetti/pull/358.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/358.patch'},
'body': 'This PR attempts to address the lack of `spaghetti` be installed in `environment.yml`.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/357',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/357/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/357/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/357/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/357',
'id': 544394895,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4NTE0OTg2',
'number': 357,
'title': 'using environment.yml for binder',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 3,
'created_at': '2020-01-01T20:21:09Z',
'updated_at': '2020-01-01T20:34:22Z',
'closed_at': '2020-01-01T20:33:21Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/357',
'html_url': 'https://github.com/pysal/spaghetti/pull/357',
'diff_url': 'https://github.com/pysal/spaghetti/pull/357.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/357.patch'},
'body': 'This PR attempts to solve the issue of notebook binders not being created with the proper requirements.\r\n\r\nSee related #78 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/354',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/354/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/354/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/354/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/354',
'id': 544370503,
'node_id': 'MDU6SXNzdWU1NDQzNzA1MDM=',
'number': 354,
'title': 'Add blob/master/ to notebooks link',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118413,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTM=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2020-01-01T15:37:18Z',
'updated_at': '2020-01-01T16:56:58Z',
'closed_at': '2020-01-01T16:56:58Z',
'author_association': 'MEMBER',
'body': 'Add `blob/master/` to the `This page was generated from notebooks/Basic_spaghetti_tutorial.ipynb` message on the [rendered notebook tutorials](https://pysal.org/spaghetti/notebooks/Basic_spaghetti_tutorial.html).\r\n\r\nFor example:\r\n`notebooks/Basic_spaghetti_tutorial.ipynb`\r\nto \r\n`blob/master/notebooks/Basic_spaghetti_tutorial.ipynb`\r\n\r\n\r\n'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/356',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/356/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/356/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/356/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/356',
'id': 544376072,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4NTAxNzIy',
'number': 356,
'title': 'addressing #354',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118413,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTM=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 3,
'created_at': '2020-01-01T16:40:34Z',
'updated_at': '2020-01-01T16:53:17Z',
'closed_at': '2020-01-01T16:53:12Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/356',
'html_url': 'https://github.com/pysal/spaghetti/pull/356',
'diff_url': 'https://github.com/pysal/spaghetti/pull/356.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/356.patch'},
'body': 'This PR addresses #354 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/355',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/355/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/355/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/355/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/355',
'id': 544371460,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4NDk4Mzg1',
'number': 355,
'title': 'Citation review',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1748719359,
'node_id': 'MDU6TGFiZWwxNzQ4NzE5MzU5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/rough%20edge',
'name': 'rough edge',
'color': 'ef6040',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6',
'html_url': 'https://github.com/pysal/spaghetti/milestone/6',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/6/labels',
'id': 4966383,
'node_id': 'MDk6TWlsZXN0b25lNDk2NjM4Mw==',
'number': 6,
'title': 'Release v1.4.1',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 4,
'closed_issues': 38,
'state': 'open',
'created_at': '2020-01-01T15:38:01Z',
'updated_at': '2020-01-25T01:28:11Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2020-01-01T15:49:04Z',
'updated_at': '2020-01-01T16:23:19Z',
'closed_at': '2020-01-01T16:23:16Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/355',
'html_url': 'https://github.com/pysal/spaghetti/pull/355',
'diff_url': 'https://github.com/pysal/spaghetti/pull/355.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/355.patch'},
'body': 'This PR rebuilds the docs for a corrections to a minor typo in citations in `network.py`'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/78',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/78/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/78/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/78/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/78',
'id': 351924584,
'node_id': 'MDU6SXNzdWUzNTE5MjQ1ODQ=',
'number': 78,
'title': 'launch binder',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 650035276,
'node_id': 'MDU6TGFiZWw2NTAwMzUyNzY=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/idea/suggestion/future%20work',
'name': 'idea/suggestion/future work',
'color': '006b75',
'default': False,
'description': None},
{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None},
{'id': 1045274760,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NzYw',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-low',
'name': 'priority-low',
'color': '77ea9d',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2018-08-19T18:41:55Z',
'updated_at': '2020-01-01T03:24:49Z',
'closed_at': '2020-01-01T03:24:49Z',
'author_association': 'MEMBER',
'body': 'Set up a `launch binder` badge for interactive notebooks.\r\n\r\nNeed `environment.yml` for non-`spaghetti` dependencies:\r\n\r\n- [ ] `matplotlib`\r\n\r\n- [ ] `geopandas`\r\n\r\n- [ ] `shapely`\r\n\r\n- [ ] `ortools`'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/353',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/353/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/353/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/353/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/353',
'id': 544303523,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4NDQ1Njky',
'number': 353,
'title': 'version 1.3.1 --> 1.4 bump',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649050,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MDUw',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/version',
'name': 'version',
'color': 'c5def5',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2020-01-01T03:06:34Z',
'updated_at': '2020-01-01T03:13:38Z',
'closed_at': '2020-01-01T03:10:53Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/353',
'html_url': 'https://github.com/pysal/spaghetti/pull/353',
'diff_url': 'https://github.com/pysal/spaghetti/pull/353.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/353.patch'},
'body': 'This PR is a version 1.3.1 --> 1.4 bump'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/352',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/352/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/352/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/352/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/352',
'id': 544302908,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4NDQ1MjQ4',
'number': 352,
'title': 'updating version and docs',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''},
{'id': 1371649050,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MDUw',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/version',
'name': 'version',
'color': 'c5def5',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2020-01-01T02:53:07Z',
'updated_at': '2020-01-01T03:00:51Z',
'closed_at': '2020-01-01T02:57:25Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/352',
'html_url': 'https://github.com/pysal/spaghetti/pull/352',
'diff_url': 'https://github.com/pysal/spaghetti/pull/352.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/352.patch'},
'body': 'updating version and docs'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/332',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/332/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/332/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/332/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/332',
'id': 524073448,
'node_id': 'MDU6SXNzdWU1MjQwNzM0NDg=',
'number': 332,
'title': 'improve spaghetti homepage and notebooks?',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 650035276,
'node_id': 'MDU6TGFiZWw2NTAwMzUyNzY=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/idea/suggestion/future%20work',
'name': 'idea/suggestion/future work',
'color': '006b75',
'default': False,
'description': None},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 5,
'created_at': '2019-11-18T00:19:41Z',
'updated_at': '2020-01-01T02:42:31Z',
'closed_at': '2020-01-01T02:42:31Z',
'author_association': 'MEMBER',
'body': 'Referencing [this critique](https://gitter.im/pysal/pysal?at=5dd1dfd7e75b2d5a19efb7cc) from the `pysal` gitter channel.\r\n\r\nPotential for improvement:\r\n * "quick-start" guide\r\n * thoroughly explain a basic workflow, while linking to other pysal submodules\r\n * better demonstration / tutorial'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/338',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/338/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/338/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/338/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/338',
'id': 534414474,
'node_id': 'MDU6SXNzdWU1MzQ0MTQ0NzQ=',
'number': 338,
'title': 'Review docs for links, etc.',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
{'login': 'rahul799',
'id': 41531498,
'node_id': 'MDQ6VXNlcjQxNTMxNDk4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/41531498?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/rahul799',
'html_url': 'https://github.com/rahul799',
'followers_url': 'https://api.github.com/users/rahul799/followers',
'following_url': 'https://api.github.com/users/rahul799/following{/other_user}',
'gists_url': 'https://api.github.com/users/rahul799/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/rahul799/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/rahul799/subscriptions',
'organizations_url': 'https://api.github.com/users/rahul799/orgs',
'repos_url': 'https://api.github.com/users/rahul799/repos',
'events_url': 'https://api.github.com/users/rahul799/events{/privacy}',
'received_events_url': 'https://api.github.com/users/rahul799/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 12,
'created_at': '2019-12-07T15:22:44Z',
'updated_at': '2020-01-01T02:42:04Z',
'closed_at': '2020-01-01T02:42:04Z',
'author_association': 'MEMBER',
'body': 'Following #336 & #337, a review of the docs are needed for stale/broken links. Examples include:\r\n * [`libpysal.weights.weights.W`](https://libpysal.readthedocs.io/en/latest/generated/libpysal.weights.W.html#libpysal.weights.W) in [`spaghetti.Network.extract_components`](https://pysal.org/spaghetti/generated/spaghetti.Network.extract_components.html#spaghetti.Network.extract_components)\r\n\r\nSpecifically check for links to other packages link `geopandas`. Be sure to check check the generated locally for format and link functionality.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/341',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/341/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/341/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/341/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/341',
'id': 541510630,
'node_id': 'MDU6SXNzdWU1NDE1MTA2MzA=',
'number': 341,
'title': 'Spelling and default value of `n_processes`',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1045274469,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NDY5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-mid',
'name': 'priority-mid',
'color': 'e2be51',
'default': False,
'description': ''},
{'id': 1748719359,
'node_id': 'MDU6TGFiZWwxNzQ4NzE5MzU5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/rough%20edge',
'name': 'rough edge',
'color': 'ef6040',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-12-22T22:49:20Z',
'updated_at': '2020-01-01T02:41:52Z',
'closed_at': '2020-01-01T02:41:52Z',
'author_association': 'MEMBER',
'body': 'While working on #339, I came across a parameter, which is present in several methods, that needs review.\r\n 1. `n_proccess` is spelled incorrectly in `Network.distancbandweights()` --> `n_processes`.\r\n 2. The default value of `n_processes` is `None`, which then defaults to `1` if the user does not specify either `"all"` for all cores of the CPU or request a specific number of cores, `2` for example. This should probably by explicitly set as a default of `n_processes =1` because there could never actually be `None` processes...\r\n\r\n\r\n * [x] [`Network.distancbandweights()`](https://github.com/pysal/spaghetti/blob/d4930d62c9073791b9f08dac6f4ee698808050e2/spaghetti/network.py#L827)\r\n* [x] [`Network.full_distance_matrix()`](https://github.com/pysal/spaghetti/blob/d4930d62c9073791b9f08dac6f4ee698808050e2/spaghetti/network.py#L1381)\r\n* [x] [`Network.allneighbordistances()`](https://github.com/pysal/spaghetti/blob/d4930d62c9073791b9f08dac6f4ee698808050e2/spaghetti/network.py#L1469)\r\n* [x] [`Network.nearestneighbordistances()`](https://github.com/pysal/spaghetti/blob/d4930d62c9073791b9f08dac6f4ee698808050e2/spaghetti/network.py#L1746)'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/330',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/330/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/330/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/330/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/330',
'id': 522375168,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQwNTg3NTcz',
'number': 330,
'title': '[WIP] TSP notebook',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1674941943,
'node_id': 'MDU6TGFiZWwxNjc0OTQxOTQz',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/WIP',
'name': 'WIP',
'color': '3913ad',
'default': False,
'description': ''},
{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/4',
'html_url': 'https://github.com/pysal/spaghetti/milestone/4',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/4/labels',
'id': 4469159,
'node_id': 'MDk6TWlsZXN0b25lNDQ2OTE1OQ==',
'number': 4,
'title': 'release +1',
'description': 'after `v1.4.2`',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 1,
'state': 'open',
'created_at': '2019-07-05T22:52:38Z',
'updated_at': '2020-01-10T01:13:35Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2019-11-13T17:44:01Z',
'updated_at': '2020-01-01T02:30:47Z',
'closed_at': '2020-01-01T02:30:47Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/330',
'html_url': 'https://github.com/pysal/spaghetti/pull/330',
'diff_url': 'https://github.com/pysal/spaghetti/pull/330.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/330.patch'},
'body': 'Addressing #110 (Traveling Salesman Problem notebook/demo)'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/342',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/342/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/342/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/342/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/342',
'id': 541534850,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU2MTQ3MDMy',
'number': 342,
'title': '[ENH][WIP]extension of #339: Rahul799 docs/bugfix',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1674941943,
'node_id': 'MDU6TGFiZWwxNjc0OTQxOTQz',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/WIP',
'name': 'WIP',
'color': '3913ad',
'default': False,
'description': ''},
{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 4,
'created_at': '2019-12-23T01:43:35Z',
'updated_at': '2020-01-01T02:31:44Z',
'closed_at': '2020-01-01T02:30:45Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/342',
'html_url': 'https://github.com/pysal/spaghetti/pull/342',
'diff_url': 'https://github.com/pysal/spaghetti/pull/342.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/342.patch'},
'body': 'This PR was initialized as #339 by @Rahul799, which addresses #336 and #338. Also, this PR partially addresses #340 and #341.\r\n\r\n'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/350',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/350/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/350/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/350/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/350',
'id': 543298230,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU3NTk1ODUz',
'number': 350,
'title': 'Revert "order analysis.py classes/functions alphabetically"',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-12-29T00:34:34Z',
'updated_at': '2019-12-29T00:58:05Z',
'closed_at': '2019-12-29T00:57:56Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/350',
'html_url': 'https://github.com/pysal/spaghetti/pull/350',
'diff_url': 'https://github.com/pysal/spaghetti/pull/350.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/350.patch'},
'body': 'Reverts pysal/spaghetti#349'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/349',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/349/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/349/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/349/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/349',
'id': 543296737,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU3NTk0ODMz',
'number': 349,
'title': 'order analysis.py classes/functions alphabetically',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1748719359,
'node_id': 'MDU6TGFiZWwxNzQ4NzE5MzU5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/rough%20edge',
'name': 'rough edge',
'color': 'ef6040',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-12-29T00:13:47Z',
'updated_at': '2019-12-29T00:25:55Z',
'closed_at': '2019-12-29T00:25:51Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/349',
'html_url': 'https://github.com/pysal/spaghetti/pull/349',
'diff_url': 'https://github.com/pysal/spaghetti/pull/349.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/349.patch'},
'body': 'This PR reorders the classes/functions in `analysis.py` alphabetically and cleans up documentation typos.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/347',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/347/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/347/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/347/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/347',
'id': 542393243,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU2ODQyNjYz',
'number': 347,
'title': 'updating requirement_dev.txt',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-12-25T23:08:48Z',
'updated_at': '2019-12-27T17:40:59Z',
'closed_at': '2019-12-27T17:40:55Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/347',
'html_url': 'https://github.com/pysal/spaghetti/pull/347',
'diff_url': 'https://github.com/pysal/spaghetti/pull/347.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/347.patch'},
'body': 'This PR adds [`watermark`](https://github.com/rasbt/watermark) to `requirement_dev.txt`.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/348',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/348/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/348/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/348/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/348',
'id': 542939486,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU3Mjg2NDA0',
'number': 348,
'title': 'update README.md',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118413,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTM=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 2,
'created_at': '2019-12-27T17:23:15Z',
'updated_at': '2019-12-27T17:40:21Z',
'closed_at': '2019-12-27T17:40:17Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/348',
'html_url': 'https://github.com/pysal/spaghetti/pull/348',
'diff_url': 'https://github.com/pysal/spaghetti/pull/348.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/348.patch'},
'body': 'correction of wrong `url` in the `recipe` badge.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/343',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/343/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/343/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/343/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/343',
'id': 541537913,
'node_id': 'MDU6SXNzdWU1NDE1Mzc5MTM=',
'number': 343,
'title': 'tarball jobs failing on Travis',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1045274760,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NzYw',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-low',
'name': 'priority-low',
'color': '77ea9d',
'default': False,
'description': ''},
{'id': 1432773648,
'node_id': 'MDU6TGFiZWwxNDMyNzczNjQ4',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/travis%20ci',
'name': 'travis ci',
'color': 'c2f23e',
'default': False,
'description': ''},
{'id': 1432772591,
'node_id': 'MDU6TGFiZWwxNDMyNzcyNTkx',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/upstream',
'name': 'upstream',
'color': 'e13aea',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 3,
'created_at': '2019-12-23T02:03:18Z',
'updated_at': '2020-01-22T01:47:28Z',
'closed_at': '2019-12-24T14:57:16Z',
'author_association': 'MEMBER',
'body': '\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\nCurrently the [`tarball ` jobs](https://github.com/pysal/spaghetti/blob/d4930d62c9073791b9f08dac6f4ee698808050e2/.travis.yml#L79-L82) are [failing on travis](https://travis-ci.org/pysal/spaghetti/jobs/628546231#L782-L795), seemingly due to an issue with version `twine`/[`importlib`](https://docs.python.org/3/library/importlib.metadata.html):\r\n\r\n```\r\nTraceback (most recent call last):\r\n File "/home/travis/miniconda/envs/TEST/lib/python3.7/site-packages/twine/__init__.py", line 22, in <module>\r\n import importlib.metadata as importlib_metadata\r\nModuleNotFoundError: No module named \'importlib.metadata\'\r\n\r\nDuring handling of the above exception, another exception occurred:\r\n\r\nTraceback (most recent call last):\r\n File "/home/travis/miniconda/envs/TEST/bin/twine", line 6, in <module>\r\n from twine.__main__ import main\r\n File "/home/travis/miniconda/envs/TEST/lib/python3.7/site-packages/twine/__init__.py", line 24, in <module>\r\n import importlib_metadata\r\nModuleNotFoundError: No module named \'importlib_metadata\'\r\nThe command "if [[ $TRAVIS_JOB_NAME == tarball* ]]; then pip wheel . -w dist --no-deps; twine check dist/*; fi" exited with 1.\r\n```'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/344',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/344/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/344/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/344/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/344',
'id': 541781794,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU2MzUxNjE1',
'number': 344,
'title': 'updating README.md',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1748719359,
'node_id': 'MDU6TGFiZWwxNzQ4NzE5MzU5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/rough%20edge',
'name': 'rough edge',
'color': 'ef6040',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-12-23T14:39:23Z',
'updated_at': '2019-12-23T14:57:29Z',
'closed_at': '2019-12-23T14:57:25Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/344',
'html_url': 'https://github.com/pysal/spaghetti/pull/344',
'diff_url': 'https://github.com/pysal/spaghetti/pull/344.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/344.patch'},
'body': 'Evening out indentation in the [Citation](https://github.com/pysal/spaghetti#bibtex-citation) section.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/339',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/339/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/339/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/339/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/339',
'id': 536972521,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUyNDA5NjIx',
'number': 339,
'title': 'links in the docs,reviewed ',
'user': {'login': 'rahul799',
'id': 41531498,
'node_id': 'MDQ6VXNlcjQxNTMxNDk4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/41531498?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/rahul799',
'html_url': 'https://github.com/rahul799',
'followers_url': 'https://api.github.com/users/rahul799/followers',
'following_url': 'https://api.github.com/users/rahul799/following{/other_user}',
'gists_url': 'https://api.github.com/users/rahul799/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/rahul799/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/rahul799/subscriptions',
'organizations_url': 'https://api.github.com/users/rahul799/orgs',
'repos_url': 'https://api.github.com/users/rahul799/repos',
'events_url': 'https://api.github.com/users/rahul799/events{/privacy}',
'received_events_url': 'https://api.github.com/users/rahul799/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'rahul799',
'id': 41531498,
'node_id': 'MDQ6VXNlcjQxNTMxNDk4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/41531498?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/rahul799',
'html_url': 'https://github.com/rahul799',
'followers_url': 'https://api.github.com/users/rahul799/followers',
'following_url': 'https://api.github.com/users/rahul799/following{/other_user}',
'gists_url': 'https://api.github.com/users/rahul799/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/rahul799/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/rahul799/subscriptions',
'organizations_url': 'https://api.github.com/users/rahul799/orgs',
'repos_url': 'https://api.github.com/users/rahul799/repos',
'events_url': 'https://api.github.com/users/rahul799/events{/privacy}',
'received_events_url': 'https://api.github.com/users/rahul799/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'rahul799',
'id': 41531498,
'node_id': 'MDQ6VXNlcjQxNTMxNDk4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/41531498?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/rahul799',
'html_url': 'https://github.com/rahul799',
'followers_url': 'https://api.github.com/users/rahul799/followers',
'following_url': 'https://api.github.com/users/rahul799/following{/other_user}',
'gists_url': 'https://api.github.com/users/rahul799/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/rahul799/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/rahul799/subscriptions',
'organizations_url': 'https://api.github.com/users/rahul799/orgs',
'repos_url': 'https://api.github.com/users/rahul799/repos',
'events_url': 'https://api.github.com/users/rahul799/events{/privacy}',
'received_events_url': 'https://api.github.com/users/rahul799/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 21,
'created_at': '2019-12-12T13:17:45Z',
'updated_at': '2019-12-27T16:32:42Z',
'closed_at': '2019-12-23T01:55:35Z',
'author_association': 'CONTRIBUTOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/339',
'html_url': 'https://github.com/pysal/spaghetti/pull/339',
'diff_url': 'https://github.com/pysal/spaghetti/pull/339.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/339.patch'},
'body': 'Hello! Please make sure to check all these boxes before submitting a Pull Request\r\n(PR). Once you have checked the boxes, feel free to remove all text except the\r\njustification in point 4. \r\n\r\n1. [x] You have run tests on this submission, either by using [Travis Continuous Integration testing](https://github.com/pysal/pysal/wiki/GitHub-Standard-Operating-Procedures#automated-testing-w-travis-ci) testing or running `nosetests` on your changes?\r\n2. [x] This pull introduces new functionality covered by [docstrings](https://en.wikipedia.org/wiki/Docstring#Python) and [unittests](https://docs.python.org/3.6/library/unittest.html)?\r\n3. [x] You have [assigned a reviewer](https://help.github.com/articles/assigning-issues-and-pull-requests-to-other-github-users/) and added relevant [labels](https://help.github.com/articles/applying-labels-to-issues-and-pull-requests/).\r\n4. [x] The justification for this PR is:\r\n * It may fix the issue #338 \r\n'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/336',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/336/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/336/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/336/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/336',
'id': 534191712,
'node_id': 'MDU6SXNzdWU1MzQxOTE3MTI=',
'number': 336,
'title': 'automatically generate docstrings for class members',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1045274469,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NDY5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-mid',
'name': 'priority-mid',
'color': 'e2be51',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 0,
'created_at': '2019-12-06T18:23:36Z',
'updated_at': '2019-12-07T01:27:51Z',
'closed_at': '2019-12-07T01:27:51Z',
'author_association': 'MEMBER',
'body': 'pysal/submodule_template#23'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/337',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/337/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/337/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/337/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/337',
'id': 534210499,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUwMTYwNDk1',
'number': 337,
'title': 'Update docs',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1045274469,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NDY5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-mid',
'name': 'priority-mid',
'color': 'e2be51',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 20,
'created_at': '2019-12-06T19:08:39Z',
'updated_at': '2019-12-07T01:26:40Z',
'closed_at': '2019-12-07T01:26:35Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/337',
'html_url': 'https://github.com/pysal/spaghetti/pull/337',
'diff_url': 'https://github.com/pysal/spaghetti/pull/337.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/337.patch'},
'body': 'This PR addresses #336 (from pysal/submodule_template#23)'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/335',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/335/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/335/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/335/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/335',
'id': 529590747,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ2NDQwMTMw',
'number': 335,
'title': 'Updating .travis.yml',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1432773648,
'node_id': 'MDU6TGFiZWwxNDMyNzczNjQ4',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/travis%20ci',
'name': 'travis ci',
'color': 'c2f23e',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-11-27T21:59:57Z',
'updated_at': '2019-11-27T22:15:01Z',
'closed_at': '2019-11-27T22:14:57Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/335',
'html_url': 'https://github.com/pysal/spaghetti/pull/335',
'diff_url': 'https://github.com/pysal/spaghetti/pull/335.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/335.patch'},
'body': 'This PR is a temporary fix for #322 (though we should still keep it open), and also standardized `bash` line breaks in `.travis.yml`.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/334',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/334/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/334/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/334/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/334',
'id': 528754167,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ1NzU2NzM4',
'number': 334,
'title': 'fix indentation in `.travis.yml`',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1432773648,
'node_id': 'MDU6TGFiZWwxNDMyNzczNjQ4',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/travis%20ci',
'name': 'travis ci',
'color': 'c2f23e',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 2,
'created_at': '2019-11-26T14:17:00Z',
'updated_at': '2019-11-26T19:01:18Z',
'closed_at': '2019-11-26T19:01:14Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/334',
'html_url': 'https://github.com/pysal/spaghetti/pull/334',
'diff_url': 'https://github.com/pysal/spaghetti/pull/334.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/334.patch'},
'body': 'This PR fixes a minor indentation inconsistency in `.travis.yml`.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/331',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/331/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/331/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/331/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/331',
'id': 522523449,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQwNzA5MzE4',
'number': 331,
'title': 'update README.md badges',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-11-13T23:03:28Z',
'updated_at': '2019-11-14T00:10:14Z',
'closed_at': '2019-11-14T00:10:11Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/331',
'html_url': 'https://github.com/pysal/spaghetti/pull/331',
'diff_url': 'https://github.com/pysal/spaghetti/pull/331.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/331.patch'},
'body': 'updating badges on README.md'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/327',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/327/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/327/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/327/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/327',
'id': 521020321,
'node_id': 'MDU6SXNzdWU1MjEwMjAzMjE=',
'number': 327,
'title': 'Citation stipulation for notebooks',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 2,
'created_at': '2019-11-11T15:14:24Z',
'updated_at': '2019-11-12T18:46:30Z',
'closed_at': '2019-11-12T18:46:30Z',
'author_association': 'MEMBER',
'body': 'A citation request should be included at the top of all notebooks. Something like:\r\n* "If any part of this notebook is used in your research, please cite with the reference found in [`README.md`](https://github.com/pysal/spaghetti#bibtex-citation)."'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/329',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/329/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/329/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/329/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/329',
'id': 521697761,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQwMDI4NTE5',
'number': 329,
'title': 'Notebooks update part 2',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118413,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTM=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-11-12T17:38:30Z',
'updated_at': '2019-11-12T18:44:35Z',
'closed_at': '2019-11-12T18:44:31Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/329',
'html_url': 'https://github.com/pysal/spaghetti/pull/329',
'diff_url': 'https://github.com/pysal/spaghetti/pull/329.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/329.patch'},
'body': 'Addressing [this problem](https://github.com/pysal/spaghetti/issues/327#issuecomment-552979566) from #327 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/328',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/328/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/328/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/328/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/328',
'id': 521645127,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzM5OTg2NjMz',
'number': 328,
'title': 'Notebooks update',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-11-12T16:07:50Z',
'updated_at': '2019-11-12T16:41:20Z',
'closed_at': '2019-11-12T16:41:16Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/328',
'html_url': 'https://github.com/pysal/spaghetti/pull/328',
'diff_url': 'https://github.com/pysal/spaghetti/pull/328.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/328.patch'},
'body': 'Addressing #327 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/324',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/324/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/324/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/324/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/324',
'id': 519565115,
'node_id': 'MDU6SXNzdWU1MTk1NjUxMTU=',
'number': 324,
'title': 'update email address in notebooks',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''},
{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 0,
'created_at': '2019-11-07T23:09:10Z',
'updated_at': '2019-11-11T00:17:19Z',
'closed_at': '2019-11-11T00:17:19Z',
'author_association': 'MEMBER',
'body': 'Along with #323 update email address in:\r\n* [Facility_Location.ipynb](https://github.com/pysal/spaghetti/blob/master/notebooks/Facility_Location.ipynb)\r\n* [Spaghetti_Pointpatterns_Empirical.ipynb](https://github.com/pysal/spaghetti/blob/master/notebooks/Spaghetti_Pointpatterns_Empirical.ipynb)'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/325',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/325/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/325/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/325/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/325',
'id': 520619826,
'node_id': 'MDU6SXNzdWU1MjA2MTk4MjY=',
'number': 325,
'title': 'Plotting error in Facility_Location.ipynb',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118413,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTM=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 0,
'created_at': '2019-11-10T15:58:16Z',
'updated_at': '2019-11-11T00:17:07Z',
'closed_at': '2019-11-11T00:17:07Z',
'author_association': 'MEMBER',
'body': '**Describe the bug**\r\n`descartes` now required for plotting polygons:\r\n\r\n`ImportError: The descartes package is required for plotting polygons in geopandas.`\r\n\r\n--> [Facility_Location.ipynb](https://github.com/pysal/spaghetti/blob/master/notebooks/Facility_Location.ipynb)\r\n\r\n\r\n\r\n```\r\n---------------------------------------------------------------------------\r\nModuleNotFoundError Traceback (most recent call last)\r\n~/miniconda3/envs/py3_spgh_dev/lib/python3.6/site-packages/geopandas/plotting.py in plot_polygon_collection(ax, geoms, values, color, cmap, vmin, vmax, **kwargs)\r\n 84 try:\r\n---> 85 from descartes.patch import PolygonPatch\r\n 86 except ImportError:\r\n\r\nModuleNotFoundError: No module named \'descartes\'\r\n\r\nDuring handling of the above exception, another exception occurred:\r\n\r\nImportError Traceback (most recent call last)\r\n<ipython-input-25-3ccffdf37b5a> in <module>\r\n 1 add_to_plot = {\'streets\':streets,\r\n 2 \'buffer\':streets_buffer}\r\n----> 3 plotter(plot_aux=add_to_plot, buffered=buff, title=title)\r\n\r\n<ipython-input-8-a2109470473d> in plotter(fig, base, plot_aux, buffered, model, pt1_size, pt2_size, plot_res, save_fig, title, figsize)\r\n 55 df.plot(ax=base, lw=2, color=\'k\', zorder=1)\r\n 56 if k == \'buffer\':\r\n---> 57 df.plot(ax=base, color=\'y\', lw=.25, alpha=.25, zorder=1)\r\n 58 if k == \'cli_tru\':\r\n 59 if plot_res:\r\n\r\n~/miniconda3/envs/py3_spgh_dev/lib/python3.6/site-packages/geopandas/geodataframe.py in plot(self, *args, **kwargs)\r\n 548 from there.\r\n 549 """\r\n--> 550 return plot_dataframe(self, *args, **kwargs)\r\n 551 \r\n 552 plot.__doc__ = plot_dataframe.__doc__\r\n\r\n~/miniconda3/envs/py3_spgh_dev/lib/python3.6/site-packages/geopandas/plotting.py in plot_dataframe(df, column, cmap, color, ax, cax, categorical, legend, scheme, k, vmin, vmax, markersize, figsize, legend_kwds, classification_kwds, **style_kwds)\r\n 425 return plot_series(df.geometry, cmap=cmap, color=color, ax=ax,\r\n 426 figsize=figsize, markersize=markersize,\r\n--> 427 **style_kwds)\r\n 428 \r\n 429 # To accept pd.Series and np.arrays as column\r\n\r\n~/miniconda3/envs/py3_spgh_dev/lib/python3.6/site-packages/geopandas/plotting.py in plot_series(s, cmap, color, ax, figsize, **style_kwds)\r\n 295 values_ = values[poly_idx] if cmap else None\r\n 296 plot_polygon_collection(ax, polys, values_, facecolor=facecolor,\r\n--> 297 cmap=cmap, **style_kwds)\r\n 298 \r\n 299 # plot all LineStrings and MultiLineString components in same collection\r\n\r\n~/miniconda3/envs/py3_spgh_dev/lib/python3.6/site-packages/geopandas/plotting.py in plot_polygon_collection(ax, geoms, values, color, cmap, vmin, vmax, **kwargs)\r\n 85 from descartes.patch import PolygonPatch\r\n 86 except ImportError:\r\n---> 87 raise ImportError("The descartes package is required"\r\n 88 " for plotting polygons in geopandas.")\r\n 89 from matplotlib.collections import PatchCollection\r\n\r\nImportError: The descartes package is required for plotting polygons in geopandas.\r\n```'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/326',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/326/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/326/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/326/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/326',
'id': 520676472,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzM5MjA1MDI4',
'number': 326,
'title': 'Updating Notebooks',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''},
{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 2,
'created_at': '2019-11-10T23:39:14Z',
'updated_at': '2019-11-11T00:17:32Z',
'closed_at': '2019-11-11T00:16:55Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/326',
'html_url': 'https://github.com/pysal/spaghetti/pull/326',
'diff_url': 'https://github.com/pysal/spaghetti/pull/326.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/326.patch'},
'body': '* `Spaghetti_Pointpatterns_Empirical.ipynb`\r\n * update email address (#324)\r\n * adopt `black` formatting\r\n\r\n* `Facility_Location.ipynb`\r\n * update email address\r\n * adopt `black` formatting\r\n * add `descartes` as required for notebook (#325)\r\n\r\n* `Network_Usage.ipynb`\r\n * adopt `black` formatting'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/323',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/323/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/323/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/323/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/323',
'id': 519534556,
'node_id': 'MDU6SXNzdWU1MTk1MzQ1NTY=',
'number': 323,
'title': "module 'pysal.explore.spaghetti' has no attribute 'element_as_gdf'",
'user': {'login': 'TransUMD',
'id': 29628412,
'node_id': 'MDQ6VXNlcjI5NjI4NDEy',
'avatar_url': 'https://avatars0.githubusercontent.com/u/29628412?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/TransUMD',
'html_url': 'https://github.com/TransUMD',
'followers_url': 'https://api.github.com/users/TransUMD/followers',
'following_url': 'https://api.github.com/users/TransUMD/following{/other_user}',
'gists_url': 'https://api.github.com/users/TransUMD/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/TransUMD/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/TransUMD/subscriptions',
'organizations_url': 'https://api.github.com/users/TransUMD/orgs',
'repos_url': 'https://api.github.com/users/TransUMD/repos',
'events_url': 'https://api.github.com/users/TransUMD/events{/privacy}',
'received_events_url': 'https://api.github.com/users/TransUMD/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1664254047,
'node_id': 'MDU6TGFiZWwxNjY0MjU0MDQ3',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/notebooks',
'name': 'notebooks',
'color': '1bc8fc',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 12,
'created_at': '2019-11-07T21:52:53Z',
'updated_at': '2019-11-10T03:35:40Z',
'closed_at': '2019-11-10T03:35:40Z',
'author_association': 'NONE',
'body': "**Describe the bug**\r\nmodule 'pysal.explore.spaghetti' has no attribute 'element_as_gdf'\r\n\r\n**To Reproduce**\r\nRunning the example Spaghetti_Pointpatterns_Empirical.ipynb\r\n"},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/318',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/318/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/318/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/318/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/318',
'id': 506862640,
'node_id': 'MDU6SXNzdWU1MDY4NjI2NDA=',
'number': 318,
'title': '[ENH] util.compute_length() — DRY',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1045274469,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NDY5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-mid',
'name': 'priority-mid',
'color': 'e2be51',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/3',
'html_url': 'https://github.com/pysal/spaghetti/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/3/labels',
'id': 4469157,
'node_id': 'MDk6TWlsZXN0b25lNDQ2OTE1Nw==',
'number': 3,
'title': 'future release',
'description': None,
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 14,
'closed_issues': 6,
'state': 'open',
'created_at': '2019-07-05T22:51:58Z',
'updated_at': '2020-01-06T18:59:07Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2019-10-14T20:38:43Z',
'updated_at': '2019-10-15T17:23:04Z',
'closed_at': '2019-10-15T17:23:04Z',
'author_association': 'MEMBER',
'body': 'The current implementation on [`spaghetti.util.compute_length()` performs a raw euclidean distance calculation using `numpy`](https://github.com/pysal/spaghetti/blob/e9d31e0c2fa937583fab225c8093955c757cd27c/spaghetti/util.py#L47). However, this same result can be obtained through [`libpysal.cg.standalone.get_points_dist()`](https://github.com/pysal/libpysal/blob/ed098ea900bde6a9913b96e162d64a72a9615e11/libpysal/cg/standalone.py#L467-L488). Switching from the current implementation to using the `libpysal.cg` implementation will be beneficial in two ways:\r\n1. improves DRY programming\r\n2. improves performance by approx. 5x, as shown below\r\n\r\n```python\r\n>>> import math\r\n>>> import numpy\r\n>>> pt1 = (0,0)\r\n>>> pt2 = (3,4)\r\n\r\n# spaghetti.util.compute_length\r\n>>> %timeit numpy.sqrt((pt1[0] - pt2[0]) ** 2 + (pt1[1] - pt2[1]) ** 2)\r\n# 1.6 µs ± 29.1 ns per loop (mean ± std. dev. of 7 runs, 1000000 loops each)\r\n\r\n# libpysal.cg.standalone.get_points_dist\r\n>>> %timeit math.hypot(pt1[0] - pt2[0], pt1[1] - pt2[1])\r\n# 277 ns ± 4.21 ns per loop (mean ± std. dev. of 7 runs, 1000000 loops each)\r\n```\r\n\r\n**This is a part of [Refactor: `spaghetti v2.0`](https://github.com/pysal/spaghetti/wiki/Refactor:-spaghetti-v2.0#keys)**'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/319',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/319/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/319/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/319/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/319',
'id': 506872133,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI3OTcxMDAz',
'number': 319,
'title': 'swapping out distance calculation function',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1045274469,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NDY5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-mid',
'name': 'priority-mid',
'color': 'e2be51',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/3',
'html_url': 'https://github.com/pysal/spaghetti/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/3/labels',
'id': 4469157,
'node_id': 'MDk6TWlsZXN0b25lNDQ2OTE1Nw==',
'number': 3,
'title': 'future release',
'description': None,
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 14,
'closed_issues': 6,
'state': 'open',
'created_at': '2019-07-05T22:51:58Z',
'updated_at': '2020-01-06T18:59:07Z',
'due_on': None,
'closed_at': None},
'comments': 2,
'created_at': '2019-10-14T21:01:12Z',
'updated_at': '2019-10-16T01:50:44Z',
'closed_at': '2019-10-15T17:21:24Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/319',
'html_url': 'https://github.com/pysal/spaghetti/pull/319',
'diff_url': 'https://github.com/pysal/spaghetti/pull/319.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/319.patch'},
'body': 'The justification for this PR is: \r\n - addressing #318 \r\n - improves performance — euclidean distance calculation\r\n - improves DRY programming'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/317',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/317/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/317/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/317/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/317',
'id': 506805098,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI3OTE4Nzg1',
'number': 317,
'title': 'adjusting snapping image in README.md',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-10-14T18:21:19Z',
'updated_at': '2019-10-14T18:55:31Z',
'closed_at': '2019-10-14T18:55:27Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/317',
'html_url': 'https://github.com/pysal/spaghetti/pull/317',
'diff_url': 'https://github.com/pysal/spaghetti/pull/317.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/317.patch'},
'body': 'improve snapping image aesthetics in README.md\r\n - center image\r\n - decrease image size'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/315',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/315/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/315/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/315/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/315',
'id': 503130238,
'node_id': 'MDU6SXNzdWU1MDMxMzAyMzg=',
'number': 315,
'title': 'inaccurate documentation — Network.split_arcs()',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-10-06T17:10:08Z',
'updated_at': '2019-10-07T23:55:23Z',
'closed_at': '2019-10-07T23:55:23Z',
'author_association': 'MEMBER',
'body': 'According to the `docstring` for [`Network.split_arcs()`](http://pysal.org/spaghetti/generated/spaghetti.Network.split_arcs.html#spaghetti.Network.split_arcs), we currently offer the functionality of either splitting network arcs by either a specific number of breaks or by a distance interval. This is incorrect as we only offer the splitting of arcs by a specified distance.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/316',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/316/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/316/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/316/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/316',
'id': 503133719,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI1MDU2MDg0',
'number': 316,
'title': 'Split arcs update',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 3,
'created_at': '2019-10-06T17:36:57Z',
'updated_at': '2019-10-07T23:55:07Z',
'closed_at': '2019-10-07T23:55:01Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/316',
'html_url': 'https://github.com/pysal/spaghetti/pull/316',
'diff_url': 'https://github.com/pysal/spaghetti/pull/316.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/316.patch'},
'body': 'Addressing #315 \r\n\r\n\r\nThis was initiated from an email:\r\n\r\n>I have what should be a quick question: in spaghetti - and referring to the Network Usage notebook on github with respect to network segmentation - what does the command n200 = ntw.split_arcs(200.0) actually do? What does the 200.0 tell spaghetti to do? Divide the network in to 200 segments, or segment the network at 200 meter intervals? Or something else entirely?'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/313',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/313/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/313/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/313/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/313',
'id': 503114879,
'node_id': 'MDU6SXNzdWU1MDMxMTQ4Nzk=',
'number': 313,
'title': 'streamline badges in README.md',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-10-06T15:19:53Z',
'updated_at': '2019-10-06T15:50:17Z',
'closed_at': '2019-10-06T15:50:17Z',
'author_association': 'MEMBER',
'body': 'remove extra badges and put into table format.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/314',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/314/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/314/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/314/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/314',
'id': 503115429,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI1MDQzOTA2',
'number': 314,
'title': 'streaming badges on README.md',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 0,
'created_at': '2019-10-06T15:22:16Z',
'updated_at': '2019-10-06T15:50:02Z',
'closed_at': '2019-10-06T15:49:57Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/314',
'html_url': 'https://github.com/pysal/spaghetti/pull/314',
'diff_url': 'https://github.com/pysal/spaghetti/pull/314.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/314.patch'},
'body': 'Addressing #313\r\n'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/312',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/312/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/312/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/312/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/312',
'id': 490446174,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzE1MDUxMjAz',
'number': 312,
'title': 'adding +travis to notification recipients',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1432773648,
'node_id': 'MDU6TGFiZWwxNDMyNzczNjQ4',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/travis%20ci',
'name': 'travis ci',
'color': 'c2f23e',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 2,
'created_at': '2019-09-06T17:45:25Z',
'updated_at': '2019-09-08T21:16:41Z',
'closed_at': '2019-09-08T21:15:47Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/312',
'html_url': 'https://github.com/pysal/spaghetti/pull/312',
'diff_url': 'https://github.com/pysal/spaghetti/pull/312.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/312.patch'},
'body': 'adding `+travis` to notification recipients in `.travis.yml`.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/309',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/309/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/309/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/309/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/309',
'id': 485432147,
'node_id': 'MDU6SXNzdWU0ODU0MzIxNDc=',
'number': 309,
'title': 'broken "development guidelines" link',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118413,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTM=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1045274206,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0MjA2',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-high',
'name': 'priority-high',
'color': 'd4c5f9',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 2,
'created_at': '2019-08-26T20:22:15Z',
'updated_at': '2019-09-01T21:15:37Z',
'closed_at': '2019-09-01T21:15:37Z',
'author_association': 'MEMBER',
'body': 'Due to switching from RTD to GH hosted docs the "development guidelines" link found in the [Contribute](https://github.com/pysal/spaghetti#contribute) section of [`README.md`](https://github.com/pysal/spaghetti/blob/master/README.md) fails.\r\n\r\nRelated: pysal/libpysal#178'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/311',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/311/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/311/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/311/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/311',
'id': 487930627,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEzMDY0NzAy',
'number': 311,
'title': 'updating broken dev link',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118413,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTM=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-09-01T21:02:49Z',
'updated_at': '2019-09-01T21:13:25Z',
'closed_at': '2019-09-01T21:10:51Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/311',
'html_url': 'https://github.com/pysal/spaghetti/pull/311',
'diff_url': 'https://github.com/pysal/spaghetti/pull/311.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/311.patch'},
'body': 'Addressing #309 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/299',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/299/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/299/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/299/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/299',
'id': 475371345,
'node_id': 'MDU6SXNzdWU0NzUzNzEzNDU=',
'number': 299,
'title': 'Update Travis CI schema',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1045274760,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NzYw',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-low',
'name': 'priority-low',
'color': '77ea9d',
'default': False,
'description': ''},
{'id': 1432773648,
'node_id': 'MDU6TGFiZWwxNDMyNzczNjQ4',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/travis%20ci',
'name': 'travis ci',
'color': 'c2f23e',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-07-31T21:44:22Z',
'updated_at': '2019-09-01T20:32:43Z',
'closed_at': '2019-09-01T20:32:43Z',
'author_association': 'MEMBER',
'body': 'Mimic the `.travis.yml` laid out in [this PR to `pysal/pysal`](https://github.com/pysal/pysal/pull/1130) for updated and streamlined procedure.'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/308',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/308/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/308/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/308/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/308',
'id': 484950166,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwNjk3Nzc3',
'number': 308,
'title': 'Update travis CI for new testing schema',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118416,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTY=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/help%20wanted',
'name': 'help wanted',
'color': '128A0C',
'default': True,
'description': None},
{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None},
{'id': 1432773648,
'node_id': 'MDU6TGFiZWwxNDMyNzczNjQ4',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/travis%20ci',
'name': 'travis ci',
'color': 'c2f23e',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 7,
'created_at': '2019-08-25T16:33:51Z',
'updated_at': '2019-09-02T16:03:07Z',
'closed_at': '2019-09-01T20:24:09Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/308',
'html_url': 'https://github.com/pysal/spaghetti/pull/308',
'diff_url': 'https://github.com/pysal/spaghetti/pull/308.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/308.patch'},
'body': 'Attempting to address #299 \r\n'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/310',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/310/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/310/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/310/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/310',
'id': 486060011,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzExNTg5MjQ3',
'number': 310,
'title': 'adding static docs badge',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-08-27T22:13:18Z',
'updated_at': '2019-08-27T22:31:38Z',
'closed_at': '2019-08-27T22:31:35Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/310',
'html_url': 'https://github.com/pysal/spaghetti/pull/310',
'diff_url': 'https://github.com/pysal/spaghetti/pull/310.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/310.patch'},
'body': 'adding static docs badge following #303'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/288',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/288/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/288/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/288/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/288',
'id': 458125165,
'node_id': 'MDU6SXNzdWU0NTgxMjUxNjU=',
'number': 288,
'title': 'mock c modules for doc dependencies',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 650035276,
'node_id': 'MDU6TGFiZWw2NTAwMzUyNzY=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/idea/suggestion/future%20work',
'name': 'idea/suggestion/future work',
'color': '006b75',
'default': False,
'description': None},
{'id': 1045274760,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NzYw',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-low',
'name': 'priority-low',
'color': '77ea9d',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 4,
'created_at': '2019-06-19T17:04:19Z',
'updated_at': '2019-08-25T18:38:10Z',
'closed_at': '2019-08-25T18:38:09Z',
'author_association': 'MEMBER',
'body': "Consider @renanxcortes's [method](https://github.com/pysal/segregation/issues/58#issuecomment-503368564) as an alternative to #268 for failing doc builds."},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/306',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/306/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/306/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/306/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/306',
'id': 484819561,
'node_id': 'MDU6SXNzdWU0ODQ4MTk1NjE=',
'number': 306,
'title': 'doi missing from citation',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 2,
'created_at': '2019-08-24T13:54:04Z',
'updated_at': '2019-08-24T18:43:13Z',
'closed_at': '2019-08-24T18:43:13Z',
'author_association': 'MEMBER',
'body': '- [x] README.md\r\n- [x] http://pysal.org/spaghetti/'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/307',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/307/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/307/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/307/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/307',
'id': 484849320,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwNjMyMTEw',
'number': 307,
'title': 'adding Zenodo DOI to README.md and website',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-08-24T18:22:59Z',
'updated_at': '2019-08-24T18:41:48Z',
'closed_at': '2019-08-24T18:37:20Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/307',
'html_url': 'https://github.com/pysal/spaghetti/pull/307',
'diff_url': 'https://github.com/pysal/spaghetti/pull/307.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/307.patch'},
'body': 'Addressing #306 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/305',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/305/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/305/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/305/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/305',
'id': 484686699,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwNTEzOTMw',
'number': 305,
'title': 'blackify setup.py',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-08-23T19:52:56Z',
'updated_at': '2019-08-23T20:04:53Z',
'closed_at': '2019-08-23T20:04:50Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/305',
'html_url': 'https://github.com/pysal/spaghetti/pull/305',
'diff_url': 'https://github.com/pysal/spaghetti/pull/305.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/305.patch'},
'body': 'minor PR\r\n\r\nimplementing code black formatting in `setup.py`'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/301',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/301/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/301/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/301/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/301',
'id': 477450975,
'node_id': 'MDU6SXNzdWU0Nzc0NTA5NzU=',
'number': 301,
'title': 'Change Read the Docs to GitHub?',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1487221050,
'node_id': 'MDU6TGFiZWwxNDg3MjIxMDUw',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/discussion',
'name': 'discussion',
'color': '1092ba',
'default': False,
'description': ''},
{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''},
{'id': 1045274469,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NDY5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-mid',
'name': 'priority-mid',
'color': 'e2be51',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 8,
'created_at': '2019-08-06T15:32:10Z',
'updated_at': '2019-08-23T19:41:33Z',
'closed_at': '2019-08-23T19:41:33Z',
'author_association': 'MEMBER',
'body': "I received the email attached below yesterday from the Read the Docs team. Basically, it says that all RTD hosted sites will have advertisements soon. While this is in no way a knock on their model, maybe it is time to switch over to the GitHub hosted docs, as mentioned in the [August 2019 dev meeting](https://hackmd.io/Kp6Mr5HlTieLikgkzXlgyg?both) and implemented in [PySAL-meta](https://github.com/pysal/pysal/pull/1134). \r\n\r\nThoughts, @weikang9009 @sjsrey?\r\n\r\n\r\n>As you might already know, Read the Docs has been using advertising to help build a sustainable business around open source documentation hosting. We call it Ethical Advertising, because we don't allow any third-party scripts for tracking, and all the ads are served from our servers. Since we started, these ads have only been displayed on docs with the Read the Docs or Alabaster themes. We are now changing this, and starting to display ads on all documentation themes. \r\n>\r\n>You're getting this email because you maintain a project that uses a custom theme, and will soon start getting ads on it. We wanted to be proactive about this change, and alert you. Our blog post has more details on these changes, including how you might opt out: https://urldefense.com/v3/__https://blog.readthedocs.com/fixed-footer-ad-all-themes/__;!5Xm4_O-4tfk!n-8e9c86LvcMedZ_BypZxnjpFDEX_OY_6S4rcJVbS0eEXPjheUB2iFu3Ak76DWjB$ \r\n>\r\n>Read the Docs is provided for free to all open source projects. Running this service requires multiple people to wear pagers, respond to GitHub issues, and other work that is mostly invisible and thankless. We are using the money generated to help support our community and make the service sustainable.\r\n>\r\n>You can read more about our advertising here: https://urldefense.com/v3/__https://docs.readthedocs.io/page/ethical-advertising.html__;!5Xm4_O-4tfk!n-8e9c86LvcMedZ_BypZxnjpFDEX_OY_6S4rcJVbS0eEXPjheUB2iFu3AqIOCOed$ . We are doing our best to respect our users, while making our service sustainable, and we care that you believe and support the work that we do. Please let us know if you have any questions or concerns, and we will do our best to address them.\r\n>\r\n>If you would like to completely remove advertising from your open source project, but Read the Docs for Business (https://urldefense.com/v3/__https://readthedocs.com__;!5Xm4_O-4tfk!n-8e9c86LvcMedZ_BypZxnjpFDEX_OY_6S4rcJVbS0eEXPjheUB2iFu3Aqi-XAH5$ ) doesn't seem like the right fit, please respond to this email to discuss alternatives to advertising such as our Gold Membership program: https://urldefense.com/v3/__https://readthedocs.org/accounts/gold/subscription/__;!5Xm4_O-4tfk!n-8e9c86LvcMedZ_BypZxnjpFDEX_OY_6S4rcJVbS0eEXPjheUB2iFu3AisyAWni$ .\r\n>\r\n>Cheers, \r\n>The Read the Docs Team"},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/304',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/304/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/304/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/304/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/304',
'id': 484677845,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwNTA2NjMw',
'number': 304,
'title': 'updating README to reflect GitHub docs',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-08-23T19:26:16Z',
'updated_at': '2019-08-23T19:39:19Z',
'closed_at': '2019-08-23T19:39:14Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/304',
'html_url': 'https://github.com/pysal/spaghetti/pull/304',
'diff_url': 'https://github.com/pysal/spaghetti/pull/304.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/304.patch'},
'body': 'updating README to reflect GitHub docs'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/303',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/303/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/303/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/303/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/303',
'id': 484641121,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwNDc2OTQx',
'number': 303,
'title': 'switching to github docs as per #301',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1371649182,
'node_id': 'MDU6TGFiZWwxMzcxNjQ5MTgy',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/docs',
'name': 'docs',
'color': 'd12c29',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 5,
'created_at': '2019-08-23T17:44:39Z',
'updated_at': '2019-08-23T19:23:27Z',
'closed_at': '2019-08-23T19:21:14Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/303',
'html_url': 'https://github.com/pysal/spaghetti/pull/303',
'diff_url': 'https://github.com/pysal/spaghetti/pull/303.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/303.patch'},
'body': 'Addressing #301 '},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/302',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/302/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/302/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/302/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/302',
'id': 482023377,
'node_id': 'MDU6SXNzdWU0ODIwMjMzNzc=',
'number': 302,
'title': 'get_versions() function',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/3',
'html_url': 'https://github.com/pysal/spaghetti/milestone/3',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/3/labels',
'id': 4469157,
'node_id': 'MDk6TWlsZXN0b25lNDQ2OTE1Nw==',
'number': 3,
'title': 'future release',
'description': None,
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 14,
'closed_issues': 6,
'state': 'open',
'created_at': '2019-07-05T22:51:58Z',
'updated_at': '2020-01-06T18:59:07Z',
'due_on': None,
'closed_at': None},
'comments': 5,
'created_at': '2019-08-18T18:31:56Z',
'updated_at': '2019-08-19T16:52:44Z',
'closed_at': '2019-08-19T16:52:44Z',
'author_association': 'MEMBER',
'body': 'Add a `get_versions()` function similar to that in `geopandas`.\r\n\r\n\r\n[`geopandas._version.py`](https://github.com/geopandas/geopandas/blob/master/geopandas/_version.py)'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/293',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/293/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/293/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/293/events',
'html_url': 'https://github.com/pysal/spaghetti/issues/293',
'id': 468173724,
'node_id': 'MDU6SXNzdWU0NjgxNzM3MjQ=',
'number': 293,
'title': 'code formatting with black',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None},
{'id': 1045274760,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NzYw',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-low',
'name': 'priority-low',
'color': '77ea9d',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 0,
'created_at': '2019-07-15T14:52:15Z',
'updated_at': '2019-08-05T00:20:42Z',
'closed_at': '2019-08-05T00:20:42Z',
'author_association': 'MEMBER',
'body': 'Update code formatting with [`black`](https://black.readthedocs.io/en/stable/), as with [`esda`](https://github.com/pysal/esda/pull/62#issuecomment-505910439)'},
{'url': 'https://api.github.com/repos/pysal/spaghetti/issues/300',
'repository_url': 'https://api.github.com/repos/pysal/spaghetti',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/issues/300/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spaghetti/issues/300/comments',
'events_url': 'https://api.github.com/repos/pysal/spaghetti/issues/300/events',
'html_url': 'https://github.com/pysal/spaghetti/pull/300',
'id': 476246782,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzAzODQxNTgw',
'number': 300,
'title': 'blackifying code (#293)',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 584118415,
'node_id': 'MDU6TGFiZWw1ODQxMTg0MTU=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 650034258,
'node_id': 'MDU6TGFiZWw2NTAwMzQyNTg=',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/package%20maintenance',
'name': 'package maintenance',
'color': 'b60205',
'default': False,
'description': None},
{'id': 1045274469,
'node_id': 'MDU6TGFiZWwxMDQ1Mjc0NDY5',
'url': 'https://api.github.com/repos/pysal/spaghetti/labels/priority-mid',
'name': 'priority-mid',
'color': 'e2be51',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2',
'html_url': 'https://github.com/pysal/spaghetti/milestone/2',
'labels_url': 'https://api.github.com/repos/pysal/spaghetti/milestones/2/labels',
'id': 4341901,
'node_id': 'MDk6TWlsZXN0b25lNDM0MTkwMQ==',
'number': 2,
'title': 'v1.4 Stable',
'description': '',
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 0,
'closed_issues': 44,
'state': 'closed',
'created_at': '2019-05-23T12:50:56Z',
'updated_at': '2020-01-02T23:09:35Z',
'due_on': '2019-12-30T08:00:00Z',
'closed_at': '2020-01-02T23:09:35Z'},
'comments': 1,
'created_at': '2019-08-02T15:50:57Z',
'updated_at': '2019-08-04T22:43:39Z',
'closed_at': '2019-08-04T22:43:35Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spaghetti/pulls/300',
'html_url': 'https://github.com/pysal/spaghetti/pull/300',
'diff_url': 'https://github.com/pysal/spaghetti/pull/300.diff',
'patch_url': 'https://github.com/pysal/spaghetti/pull/300.patch'},
'body': 'The justification for this PR is:\r\n\r\nThis PR:\r\n\r\n- adopts [`black`](https://black.readthedocs.io/en/stable/) code formatting to adopt uniform readability across projects.\r\n- adds the `black` badge to `README.md`.\r\n- adresses #293\r\n'}],
'mgwr': [{'url': 'https://api.github.com/repos/pysal/mgwr/issues/74',
'repository_url': 'https://api.github.com/repos/pysal/mgwr',
'labels_url': 'https://api.github.com/repos/pysal/mgwr/issues/74/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mgwr/issues/74/comments',
'events_url': 'https://api.github.com/repos/pysal/mgwr/issues/74/events',
'html_url': 'https://github.com/pysal/mgwr/pull/74',
'id': 534958256,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUwNzM2OTc2',
'number': 74,
'title': 'Update .travis for proper dual tests',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1035836899,
'node_id': 'MDU6TGFiZWwxMDM1ODM2ODk5',
'url': 'https://api.github.com/repos/pysal/mgwr/labels/maintenance',
'name': 'maintenance',
'color': 'f9d0c4',
'default': False,
'description': 'Package maintenance for pysal reorg, etc.'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-12-09T14:13:43Z',
'updated_at': '2019-12-29T00:20:39Z',
'closed_at': '2019-12-29T00:20:35Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/mgwr/pulls/74',
'html_url': 'https://github.com/pysal/mgwr/pull/74',
'diff_url': 'https://github.com/pysal/mgwr/pull/74.diff',
'patch_url': 'https://github.com/pysal/mgwr/pull/74.patch'},
'body': '* Addressing pysal/pysal#1145\r\n* adding updated options/syntax to `.travis.yml`'}],
'spglm': [{'url': 'https://api.github.com/repos/pysal/spglm/issues/26',
'repository_url': 'https://api.github.com/repos/pysal/spglm',
'labels_url': 'https://api.github.com/repos/pysal/spglm/issues/26/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spglm/issues/26/comments',
'events_url': 'https://api.github.com/repos/pysal/spglm/issues/26/events',
'html_url': 'https://github.com/pysal/spglm/pull/26',
'id': 537933786,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUzMTg5OTAw',
'number': 26,
'title': 'addressing pysal/pysal#1145',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 519739862,
'node_id': 'MDU6TGFiZWw1MTk3Mzk4NjI=',
'url': 'https://api.github.com/repos/pysal/spglm/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-12-14T16:22:11Z',
'updated_at': '2019-12-29T00:23:08Z',
'closed_at': '2019-12-29T00:23:05Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spglm/pulls/26',
'html_url': 'https://github.com/pysal/spglm/pull/26',
'diff_url': 'https://github.com/pysal/spglm/pull/26.diff',
'patch_url': 'https://github.com/pysal/spglm/pull/26.patch'},
'body': 'addressing the travis dual testing problem raised in pysal/pysal#1145'}],
'spint': [{'url': 'https://api.github.com/repos/pysal/spint/issues/24',
'repository_url': 'https://api.github.com/repos/pysal/spint',
'labels_url': 'https://api.github.com/repos/pysal/spint/issues/24/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spint/issues/24/comments',
'events_url': 'https://api.github.com/repos/pysal/spint/issues/24/events',
'html_url': 'https://github.com/pysal/spint/pull/24',
'id': 538120534,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUzMzI1MTU5',
'number': 24,
'title': 'addressing pysal/pysal#1145',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 519739384,
'node_id': 'MDU6TGFiZWw1MTk3MzkzODQ=',
'url': 'https://api.github.com/repos/pysal/spint/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-12-15T23:07:11Z',
'updated_at': '2019-12-30T19:27:48Z',
'closed_at': '2019-12-30T19:27:45Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spint/pulls/24',
'html_url': 'https://github.com/pysal/spint/pull/24',
'diff_url': 'https://github.com/pysal/spint/pull/24.diff',
'patch_url': 'https://github.com/pysal/spint/pull/24.patch'},
'body': 'addressing pysal/pysal#1145'}],
'spreg': [{'url': 'https://api.github.com/repos/pysal/spreg/issues/30',
'repository_url': 'https://api.github.com/repos/pysal/spreg',
'labels_url': 'https://api.github.com/repos/pysal/spreg/issues/30/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spreg/issues/30/comments',
'events_url': 'https://api.github.com/repos/pysal/spreg/issues/30/events',
'html_url': 'https://github.com/pysal/spreg/pull/30',
'id': 538620729,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUzNzM1MDQ4',
'number': 30,
'title': '(Bug) deal with libpysal example data sets change and fix inline doctests',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 926682635,
'node_id': 'MDU6TGFiZWw5MjY2ODI2MzU=',
'url': 'https://api.github.com/repos/pysal/spreg/labels/bug',
'name': 'bug',
'color': 'd73a4a',
'default': True,
'description': "Something isn't working"},
{'id': 1694056123,
'node_id': 'MDU6TGFiZWwxNjk0MDU2MTIz',
'url': 'https://api.github.com/repos/pysal/spreg/labels/docs',
'name': 'docs',
'color': 'a9f9b5',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/spreg/milestones/1',
'html_url': 'https://github.com/pysal/spreg/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/spreg/milestones/1/labels',
'id': 4895099,
'node_id': 'MDk6TWlsZXN0b25lNDg5NTA5OQ==',
'number': 1,
'title': 'next release',
'description': None,
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 1,
'closed_issues': 3,
'state': 'open',
'created_at': '2019-12-02T14:47:10Z',
'updated_at': '2020-01-20T19:34:32Z',
'due_on': None,
'closed_at': None},
'comments': 0,
'created_at': '2019-12-16T19:57:25Z',
'updated_at': '2020-01-20T19:34:32Z',
'closed_at': '2020-01-20T19:34:32Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spreg/pulls/30',
'html_url': 'https://github.com/pysal/spreg/pull/30',
'diff_url': 'https://github.com/pysal/spreg/pull/30.diff',
'patch_url': 'https://github.com/pysal/spreg/pull/30.patch'},
'body': 'This PR is to fulfill three purposes:\r\n* deal with libpysal example data sets change. As some example data sets have been removed out of the source distribution, we need to remote fetch these data sets using the newly defined APIs (detailed in https://github.com/pysal/libpysal/pull/176):\r\n * three data sets used for spreg testing are impacted: South, Baltimore, and Natregimes\r\n* fix all inline doctests\r\n * Now all doctests are passing locally\r\n* update sphinx configurations'},
{'url': 'https://api.github.com/repos/pysal/spreg/issues/28',
'repository_url': 'https://api.github.com/repos/pysal/spreg',
'labels_url': 'https://api.github.com/repos/pysal/spreg/issues/28/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spreg/issues/28/comments',
'events_url': 'https://api.github.com/repos/pysal/spreg/issues/28/events',
'html_url': 'https://github.com/pysal/spreg/pull/28',
'id': 532220461,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ4NTE1NjE1',
'number': 28,
'title': 'add online docs badge to readme',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1694056123,
'node_id': 'MDU6TGFiZWwxNjk0MDU2MTIz',
'url': 'https://api.github.com/repos/pysal/spreg/labels/docs',
'name': 'docs',
'color': 'a9f9b5',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/spreg/milestones/1',
'html_url': 'https://github.com/pysal/spreg/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/spreg/milestones/1/labels',
'id': 4895099,
'node_id': 'MDk6TWlsZXN0b25lNDg5NTA5OQ==',
'number': 1,
'title': 'next release',
'description': None,
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 1,
'closed_issues': 3,
'state': 'open',
'created_at': '2019-12-02T14:47:10Z',
'updated_at': '2020-01-20T19:34:32Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2019-12-03T19:43:56Z',
'updated_at': '2019-12-04T17:57:54Z',
'closed_at': '2019-12-04T17:57:54Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spreg/pulls/28',
'html_url': 'https://github.com/pysal/spreg/pull/28',
'diff_url': 'https://github.com/pysal/spreg/pull/28.diff',
'patch_url': 'https://github.com/pysal/spreg/pull/28.patch'},
'body': 'https://spreg.readthedocs.io/en/latest/'},
{'url': 'https://api.github.com/repos/pysal/spreg/issues/1',
'repository_url': 'https://api.github.com/repos/pysal/spreg',
'labels_url': 'https://api.github.com/repos/pysal/spreg/issues/1/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spreg/issues/1/comments',
'events_url': 'https://api.github.com/repos/pysal/spreg/issues/1/events',
'html_url': 'https://github.com/pysal/spreg/issues/1',
'id': 321480086,
'node_id': 'MDU6SXNzdWUzMjE0ODAwODY=',
'number': 1,
'title': 'Docstrings use references to older pysal bibliography',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 986408748,
'node_id': 'MDU6TGFiZWw5ODY0MDg3NDg=',
'url': 'https://api.github.com/repos/pysal/spreg/labels/scipy2018',
'name': 'scipy2018',
'color': '0e8a16',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 2,
'created_at': '2018-05-09T08:37:12Z',
'updated_at': '2019-12-03T19:21:16Z',
'closed_at': '2019-12-03T19:21:16Z',
'author_association': 'MEMBER',
'body': 'For example:\r\n\r\n```\r\n \r\n GMM method for a spatial error model with heteroskedasticity (note: no\r\n consistency checks, diagnostics or constant added); based on Arraiz\r\n et al [Arraiz2010]_, following Anselin [Anselin2011]_.\r\n\r\n```\r\n\r\nWe may want to develop a centralized scheme were all pysal projects can reference the same master bib source.'},
{'url': 'https://api.github.com/repos/pysal/spreg/issues/27',
'repository_url': 'https://api.github.com/repos/pysal/spreg',
'labels_url': 'https://api.github.com/repos/pysal/spreg/issues/27/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spreg/issues/27/comments',
'events_url': 'https://api.github.com/repos/pysal/spreg/issues/27/events',
'html_url': 'https://github.com/pysal/spreg/pull/27',
'id': 527528050,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ0Nzg4Njg3',
'number': 27,
'title': 'spreg Documentation website',
'user': {'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1694056123,
'node_id': 'MDU6TGFiZWwxNjk0MDU2MTIz',
'url': 'https://api.github.com/repos/pysal/spreg/labels/docs',
'name': 'docs',
'color': 'a9f9b5',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'pedrovma',
'id': 4238922,
'node_id': 'MDQ6VXNlcjQyMzg5MjI=',
'avatar_url': 'https://avatars0.githubusercontent.com/u/4238922?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/pedrovma',
'html_url': 'https://github.com/pedrovma',
'followers_url': 'https://api.github.com/users/pedrovma/followers',
'following_url': 'https://api.github.com/users/pedrovma/following{/other_user}',
'gists_url': 'https://api.github.com/users/pedrovma/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/pedrovma/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/pedrovma/subscriptions',
'organizations_url': 'https://api.github.com/users/pedrovma/orgs',
'repos_url': 'https://api.github.com/users/pedrovma/repos',
'events_url': 'https://api.github.com/users/pedrovma/events{/privacy}',
'received_events_url': 'https://api.github.com/users/pedrovma/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'pedrovma',
'id': 4238922,
'node_id': 'MDQ6VXNlcjQyMzg5MjI=',
'avatar_url': 'https://avatars0.githubusercontent.com/u/4238922?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/pedrovma',
'html_url': 'https://github.com/pedrovma',
'followers_url': 'https://api.github.com/users/pedrovma/followers',
'following_url': 'https://api.github.com/users/pedrovma/following{/other_user}',
'gists_url': 'https://api.github.com/users/pedrovma/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/pedrovma/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/pedrovma/subscriptions',
'organizations_url': 'https://api.github.com/users/pedrovma/orgs',
'repos_url': 'https://api.github.com/users/pedrovma/repos',
'events_url': 'https://api.github.com/users/pedrovma/events{/privacy}',
'received_events_url': 'https://api.github.com/users/pedrovma/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/spreg/milestones/1',
'html_url': 'https://github.com/pysal/spreg/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/spreg/milestones/1/labels',
'id': 4895099,
'node_id': 'MDk6TWlsZXN0b25lNDg5NTA5OQ==',
'number': 1,
'title': 'next release',
'description': None,
'creator': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 1,
'closed_issues': 3,
'state': 'open',
'created_at': '2019-12-02T14:47:10Z',
'updated_at': '2020-01-20T19:34:32Z',
'due_on': None,
'closed_at': None},
'comments': 8,
'created_at': '2019-11-23T08:23:15Z',
'updated_at': '2019-12-03T19:20:21Z',
'closed_at': '2019-12-03T19:20:21Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spreg/pulls/27',
'html_url': 'https://github.com/pysal/spreg/pull/27',
'diff_url': 'https://github.com/pysal/spreg/pull/27.diff',
'patch_url': 'https://github.com/pysal/spreg/pull/27.patch'},
'body': "This PR is to \r\n* complete and publish the documentation website with readthedocs. I have tested it on my fork and it builds successfully https://spreg-wei.readthedocs.io/en/latest/\r\n\r\n* configure travis CI to for dual testing dependency libpysal on pypi and github (allow failure for the most updated github version). The travis error seems to come from `libpysal` weight's `silent_island_warning` as this attribute was renamed to `silence_warnings` but not all occurrences were updated (also reported in https://github.com/pysal/libpysal/issues/204)\r\n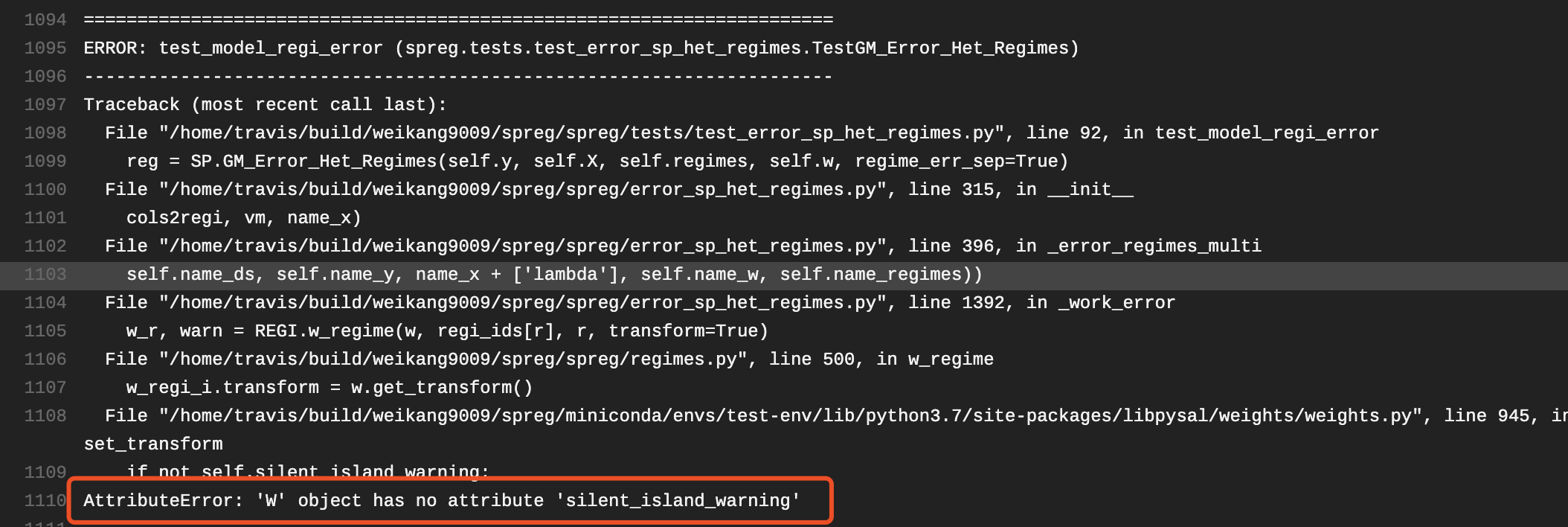\r\n"}],
'spvcm': [{'url': 'https://api.github.com/repos/pysal/spvcm/issues/10',
'repository_url': 'https://api.github.com/repos/pysal/spvcm',
'labels_url': 'https://api.github.com/repos/pysal/spvcm/issues/10/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/spvcm/issues/10/comments',
'events_url': 'https://api.github.com/repos/pysal/spvcm/issues/10/events',
'html_url': 'https://github.com/pysal/spvcm/pull/10',
'id': 539102068,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU0MTM1MTE4',
'number': 10,
'title': 'addressing pysal #1139 & new libpysal.examples schema',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-12-17T14:28:52Z',
'updated_at': '2020-01-24T21:31:51Z',
'closed_at': '2020-01-24T21:28:36Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/spvcm/pulls/10',
'html_url': 'https://github.com/pysal/spvcm/pull/10',
'diff_url': 'https://github.com/pysal/spvcm/pull/10.diff',
'patch_url': 'https://github.com/pysal/spvcm/pull/10.patch'},
'body': "This PR address both pysal/pysal#1139 and the new `libpysal.examples` schema introduced in [`libpysal` v4.2](https://github.com/pysal/libpysal/releases/tag/v4.2.0).\r\n\r\nAs far as the `try`/`except` block in [`spvcm.diagnostics`](https://github.com/pysal/spvcm/blob/63a23adf9fb8bf28e64d4b692018aae15222d816/spvcm/diagnostics.py#L11-L25) (also see below), placing this chunk within the function would have to happen in both [`summarize`](https://github.com/pysal/spvcm/blob/63a23adf9fb8bf28e64d4b692018aae15222d816/spvcm/diagnostics.py#L64) and [`_effective_size`](https://github.com/pysal/spvcm/blob/63a23adf9fb8bf28e64d4b692018aae15222d816/spvcm/diagnostics.py#L451-L457) (without some [minor] refactoring).\r\n\r\n\r\n\r\n```python\r\ntry:\r\n from rpy2.rinterface import RRuntimeError\r\n from rpy2.robjects.packages import importr\r\n from rpy2.robjects.numpy2ri import numpy2ri\r\n import rpy2.robjects as ro\r\n ro.conversion.py2ri = numpy2ri\r\n _coda = importr('coda')\r\n _HAS_CODA = True\r\n _HAS_RPY2 = True\r\nexcept (ImportError, LookupError):\r\n _HAS_CODA = False\r\n _HAS_RPY2 = False\r\nexcept RRuntimeError:\r\n _HAS_CODA = False\r\n _HAS_RPY2 = True\r\n```"}],
'tobler': [{'url': 'https://api.github.com/repos/pysal/tobler/issues/48',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/48/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/48/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/48/events',
'html_url': 'https://github.com/pysal/tobler/pull/48',
'id': 553728339,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY2MDA5ODQ0',
'number': 48,
'title': 'Merge pull request #48 from knaaptime/master',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-22T18:53:04Z',
'updated_at': '2020-01-22T18:53:22Z',
'closed_at': '2020-01-22T18:53:21Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/48',
'html_url': 'https://github.com/pysal/tobler/pull/48',
'diff_url': 'https://github.com/pysal/tobler/pull/48.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/48.patch'},
'body': 'the last pr was built with an older version of sphinx, so had the api docs center-aligned. This is just rea simple rebuild using the latest version'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/47',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/47/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/47/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/47/events',
'html_url': 'https://github.com/pysal/tobler/pull/47',
'id': 551832543,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY0NDcxMTgz',
'number': 47,
'title': 'Merge pull request #47 from jGaboardi/update_docs',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2020-01-18T22:14:30Z',
'updated_at': '2020-01-19T18:06:00Z',
'closed_at': '2020-01-19T07:31:18Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/47',
'html_url': 'https://github.com/pysal/tobler/pull/47',
'diff_url': 'https://github.com/pysal/tobler/pull/47.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/47.patch'},
'body': 'Adding document updates for #46 '},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/46',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/46/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/46/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/46/events',
'html_url': 'https://github.com/pysal/tobler/pull/46',
'id': 546599894,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzYwMjQ2NDE4',
'number': 46,
'title': 'Update installation.rst',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2020-01-08T01:47:57Z',
'updated_at': '2020-01-18T22:17:00Z',
'closed_at': '2020-01-18T22:17:00Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/46',
'html_url': 'https://github.com/pysal/tobler/pull/46',
'diff_url': 'https://github.com/pysal/tobler/pull/46.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/46.patch'},
'body': 'This PR address the following:\r\n * Python 3.5/6 are mentioned in `installation`, but `tobler` supports 3.6/7.\r\n * minor formatting improvements.'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/45',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/45/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/45/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/45/events',
'html_url': 'https://github.com/pysal/tobler/pull/45',
'id': 545469334,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MzM4MjU4',
'number': 45,
'title': 'MNT: release tooling',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2020-01-05T21:29:30Z',
'updated_at': '2020-01-05T22:05:17Z',
'closed_at': '2020-01-05T22:05:17Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/45',
'html_url': 'https://github.com/pysal/tobler/pull/45',
'diff_url': 'https://github.com/pysal/tobler/pull/45.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/45.patch'},
'body': '0.2.0 can be released after this merge.'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/44',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/44/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/44/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/44/events',
'html_url': 'https://github.com/pysal/tobler/pull/44',
'id': 545451812,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MzI1Nzkw',
'number': 44,
'title': 'add pysal integration/import test',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-05T18:41:20Z',
'updated_at': '2020-01-05T21:15:38Z',
'closed_at': '2020-01-05T21:15:38Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/44',
'html_url': 'https://github.com/pysal/tobler/pull/44',
'diff_url': 'https://github.com/pysal/tobler/pull/44.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/44.patch'},
'body': 'during pysal metapackage integration, we only need to test that the packages import, so this adds a simple test that does so.\r\n\r\nthis means we can skip the actual (long running) tests during pysal integration, as they are already run on the subpackages'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/43',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/43/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/43/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/43/events',
'html_url': 'https://github.com/pysal/tobler/pull/43',
'id': 544692290,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU4NzUwMjc2',
'number': 43,
'title': 'update docs',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2020-01-02T18:29:26Z',
'updated_at': '2020-01-02T18:51:36Z',
'closed_at': '2020-01-02T18:51:34Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/43',
'html_url': 'https://github.com/pysal/tobler/pull/43',
'diff_url': 'https://github.com/pysal/tobler/pull/43.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/43.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/40',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/40/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/40/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/40/events',
'html_url': 'https://github.com/pysal/tobler/pull/40',
'id': 537155073,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUyNTYxODk3',
'number': 40,
'title': 'return dfs',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 4,
'created_at': '2019-12-12T18:56:28Z',
'updated_at': '2020-01-02T18:20:53Z',
'closed_at': '2020-01-02T18:20:53Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/40',
'html_url': 'https://github.com/pysal/tobler/pull/40',
'diff_url': 'https://github.com/pysal/tobler/pull/40.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/40.patch'},
'body': 'this enables a more consistent API by ensuring that the interpolation functions all return geodataframes with the target geometry'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/42',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/42/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/42/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/42/events',
'html_url': 'https://github.com/pysal/tobler/pull/42',
'id': 542712431,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU3MTAwNjA4',
'number': 42,
'title': 'Move example data to remotes',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-12-27T01:47:51Z',
'updated_at': '2019-12-29T00:30:40Z',
'closed_at': '2019-12-29T00:30:40Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/42',
'html_url': 'https://github.com/pysal/tobler/pull/42',
'diff_url': 'https://github.com/pysal/tobler/pull/42.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/42.patch'},
'body': 'This will reduce the installation footprint.'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/41',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/41/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/41/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/41/events',
'html_url': 'https://github.com/pysal/tobler/issues/41',
'id': 540461525,
'node_id': 'MDU6SXNzdWU1NDA0NjE1MjU=',
'number': 41,
'title': 'revisit regression approach',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 9,
'created_at': '2019-12-19T17:46:36Z',
'updated_at': '2019-12-20T17:21:25Z',
'closed_at': '2019-12-20T17:21:25Z',
'author_association': 'MEMBER',
'body': "Currently the `linear_model` function does not work as I would expect. Right now, it calls rasterstats to get pixel counts for the source_df, then estimates a model that relates pixel counts to polygon population. Then, it takes that relationship as given (i.e. pixels become non-stochastic weights that relate each pixel value with a certain number of people), ignores the model's error term, and sums up the pixels in the target_df to estimate population. We're like, wayy overshooting using this approach\r\n\r\nInstead we should:\r\n\r\n1) call rasterstats to get pixel counts in source_df\r\n2) estimate a model based on pixel counts and population\r\n3) call rasterstats to get pixel counts in target_df\r\n4) use the model to simulate values in the target_df\r\n\r\nThat should get proper model-based estimation and get us away from the need to create a correspondence table and mask out non-overlapping pixels, etc. (and hopefully speed things up)\r\n\r\nwould you agree @renanxcortes ?"},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/36',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/36/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/36/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/36/events',
'html_url': 'https://github.com/pysal/tobler/issues/36',
'id': 533509825,
'node_id': 'MDU6SXNzdWU1MzM1MDk4MjU=',
'number': 36,
'title': 'generalize regression approach',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-12-05T18:06:35Z',
'updated_at': '2019-12-12T18:58:32Z',
'closed_at': '2019-12-12T18:58:31Z',
'author_association': 'MEMBER',
'body': 'currently, the [function](https://github.com/pysal/tobler/blob/4f8b098ada12f0f5b6ad17719754002e6535e079/tobler/area_weighted/vectorized_raster_interpolation.py#L189) that does the heavy lifting in the regression approach is hardcoded to use pixel counts, but that precludes the ability to specify more complex models (e.g. log-transforming the pixel counts, adding interactions, or including more input data).\r\n\r\ninstead, we should allow the function to take a patsy-style model formulation as a string instead of assuming we know what the user wants in [this line](https://github.com/pysal/tobler/blob/4f8b098ada12f0f5b6ad17719754002e6535e079/tobler/area_weighted/vectorized_raster_interpolation.py#L260) '},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/38',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/38/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/38/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/38/events',
'html_url': 'https://github.com/pysal/tobler/issues/38',
'id': 534645679,
'node_id': 'MDU6SXNzdWU1MzQ2NDU2Nzk=',
'number': 38,
'title': 'License?',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 2,
'created_at': '2019-12-09T01:53:34Z',
'updated_at': '2019-12-12T18:57:59Z',
'closed_at': '2019-12-12T18:57:59Z',
'author_association': 'MEMBER',
'body': "There doesn't appear to be a license in the repo and [the link with README.md](https://github.com/pysal/tobler/blob/master/LICENSE.txt) is broken."},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/34',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/34/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/34/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/34/events',
'html_url': 'https://github.com/pysal/tobler/issues/34',
'id': 533043694,
'node_id': 'MDU6SXNzdWU1MzMwNDM2OTQ=',
'number': 34,
'title': 'wrapper for regression approach',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1499380243,
'node_id': 'MDU6TGFiZWwxNDk5MzgwMjQz',
'url': 'https://api.github.com/repos/pysal/tobler/labels/enhancement',
'name': 'enhancement',
'color': 'a2eeef',
'default': True,
'description': 'New feature or request'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/tobler/milestones/1',
'html_url': 'https://github.com/pysal/tobler/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/tobler/milestones/1/labels',
'id': 4593152,
'node_id': 'MDk6TWlsZXN0b25lNDU5MzE1Mg==',
'number': 1,
'title': '0.1 Release',
'description': '',
'creator': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 3,
'closed_issues': 3,
'state': 'open',
'created_at': '2019-08-21T20:32:25Z',
'updated_at': '2019-12-12T18:57:41Z',
'due_on': None,
'closed_at': None},
'comments': 1,
'created_at': '2019-12-05T00:37:27Z',
'updated_at': '2019-12-12T18:57:42Z',
'closed_at': '2019-12-12T18:57:41Z',
'author_association': 'MEMBER',
'body': 'we should put together a wrapper function that encapsulates the logic [here](https://github.com/pysal/tobler/blob/master/examples/regression_vectorized_raster_example.ipynb) and has a signature similar to `area_interpolate` and `masked_area_interpolate`'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/39',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/39/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/39/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/39/events',
'html_url': 'https://github.com/pysal/tobler/pull/39',
'id': 536667027,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUyMTU5MTk2',
'number': 39,
'title': 'update docs',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 7,
'created_at': '2019-12-11T23:17:07Z',
'updated_at': '2019-12-12T08:25:45Z',
'closed_at': '2019-12-12T08:25:43Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/39',
'html_url': 'https://github.com/pysal/tobler/pull/39',
'diff_url': 'https://github.com/pysal/tobler/pull/39.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/39.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/9',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/9/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/9/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/9/events',
'html_url': 'https://github.com/pysal/tobler/issues/9',
'id': 483636645,
'node_id': 'MDU6SXNzdWU0ODM2MzY2NDU=',
'number': 9,
'title': 'sphinx docs',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1499380240,
'node_id': 'MDU6TGFiZWwxNDk5MzgwMjQw',
'url': 'https://api.github.com/repos/pysal/tobler/labels/documentation',
'name': 'documentation',
'color': '0075ca',
'default': True,
'description': 'Improvements or additions to documentation'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/tobler/milestones/1',
'html_url': 'https://github.com/pysal/tobler/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/tobler/milestones/1/labels',
'id': 4593152,
'node_id': 'MDk6TWlsZXN0b25lNDU5MzE1Mg==',
'number': 1,
'title': '0.1 Release',
'description': '',
'creator': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 3,
'closed_issues': 3,
'state': 'open',
'created_at': '2019-08-21T20:32:25Z',
'updated_at': '2019-12-12T18:57:41Z',
'due_on': None,
'closed_at': None},
'comments': 3,
'created_at': '2019-08-21T20:30:38Z',
'updated_at': '2019-12-12T00:00:33Z',
'closed_at': '2019-12-12T00:00:33Z',
'author_association': 'MEMBER',
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/37',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/37/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/37/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/37/events',
'html_url': 'https://github.com/pysal/tobler/pull/37',
'id': 534584733,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUwNDQzMTc4',
'number': 37,
'title': 'remove shap and xgboost as hard deps',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-08T19:00:50Z',
'updated_at': '2019-12-08T19:11:47Z',
'closed_at': '2019-12-08T19:11:45Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/37',
'html_url': 'https://github.com/pysal/tobler/pull/37',
'diff_url': 'https://github.com/pysal/tobler/pull/37.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/37.patch'},
'body': 'pip really struggles with xgboost, even when its already in the environment. This restores it as an optional dependency'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/35',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/35/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/35/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/35/events',
'html_url': 'https://github.com/pysal/tobler/pull/35',
'id': 533438961,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ5NTEzMzA2',
'number': 35,
'title': 'cosmetic upgrades',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-05T15:55:24Z',
'updated_at': '2019-12-06T17:29:29Z',
'closed_at': '2019-12-06T17:29:27Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/35',
'html_url': 'https://github.com/pysal/tobler/pull/35',
'diff_url': 'https://github.com/pysal/tobler/pull/35.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/35.patch'},
'body': 'this fixes the alignment in the docs and adds a header image'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/33',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/33/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/33/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/33/events',
'html_url': 'https://github.com/pysal/tobler/pull/33',
'id': 533002736,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ5MTUwNjc2',
'number': 33,
'title': 'reorg and add masked_area_interpolate func',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-04T23:26:34Z',
'updated_at': '2019-12-05T00:51:39Z',
'closed_at': '2019-12-05T00:51:39Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/33',
'html_url': 'https://github.com/pysal/tobler/pull/33',
'diff_url': 'https://github.com/pysal/tobler/pull/33.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/33.patch'},
'body': 'this reorganizes the module to match the architecture planning docs and adds a wrapper function for doing raster-masked area weighted interpolation'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/31',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/31/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/31/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/31/events',
'html_url': 'https://github.com/pysal/tobler/pull/31',
'id': 532931560,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ5MDkwMzE0',
'number': 31,
'title': 'Move data into data direcotry for examples',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-04T20:59:57Z',
'updated_at': '2019-12-04T23:03:53Z',
'closed_at': '2019-12-04T23:03:52Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/31',
'html_url': 'https://github.com/pysal/tobler/pull/31',
'diff_url': 'https://github.com/pysal/tobler/pull/31.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/31.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/32',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/32/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/32/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/32/events',
'html_url': 'https://github.com/pysal/tobler/pull/32',
'id': 532931600,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ5MDkwMzQw',
'number': 32,
'title': 'Environment',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-04T21:00:05Z',
'updated_at': '2019-12-04T23:03:28Z',
'closed_at': '2019-12-04T23:03:28Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/32',
'html_url': 'https://github.com/pysal/tobler/pull/32',
'diff_url': 'https://github.com/pysal/tobler/pull/32.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/32.patch'},
'body': 'this adds the missing dependencies to the environment.yml'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/8',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/8/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/8/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/8/events',
'html_url': 'https://github.com/pysal/tobler/issues/8',
'id': 483636576,
'node_id': 'MDU6SXNzdWU0ODM2MzY1NzY=',
'number': 8,
'title': 'decide on working notebooks',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1499380240,
'node_id': 'MDU6TGFiZWwxNDk5MzgwMjQw',
'url': 'https://api.github.com/repos/pysal/tobler/labels/documentation',
'name': 'documentation',
'color': '0075ca',
'default': True,
'description': 'Improvements or additions to documentation'}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/tobler/milestones/1',
'html_url': 'https://github.com/pysal/tobler/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/tobler/milestones/1/labels',
'id': 4593152,
'node_id': 'MDk6TWlsZXN0b25lNDU5MzE1Mg==',
'number': 1,
'title': '0.1 Release',
'description': '',
'creator': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 3,
'closed_issues': 3,
'state': 'open',
'created_at': '2019-08-21T20:32:25Z',
'updated_at': '2019-12-12T18:57:41Z',
'due_on': None,
'closed_at': None},
'comments': 0,
'created_at': '2019-08-21T20:30:30Z',
'updated_at': '2019-12-04T20:42:40Z',
'closed_at': '2019-12-04T20:42:40Z',
'author_association': 'MEMBER',
'body': '- label those that are for docs go into notebooks directory\r\n- those that are for development go into gists\r\n - link from a wiki page (development notebooks)'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/30',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/30/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/30/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/30/events',
'html_url': 'https://github.com/pysal/tobler/pull/30',
'id': 529668280,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ2NTAxNTQ0',
'number': 30,
'title': 'Reorg',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1702532668,
'node_id': 'MDU6TGFiZWwxNzAyNTMyNjY4',
'url': 'https://api.github.com/repos/pysal/tobler/labels/WIP',
'name': 'WIP',
'color': '5da5c1',
'default': False,
'description': 'Work in progress, do not merge'}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-11-28T03:10:43Z',
'updated_at': '2019-12-02T20:22:50Z',
'closed_at': '2019-12-02T20:22:50Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/30',
'html_url': 'https://github.com/pysal/tobler/pull/30',
'diff_url': 'https://github.com/pysal/tobler/pull/30.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/30.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/29',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/29/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/29/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/29/events',
'html_url': 'https://github.com/pysal/tobler/pull/29',
'id': 529007797,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQ1OTY0OTQ5',
'number': 29,
'title': 'fix for quilt dep clash',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-11-26T22:30:56Z',
'updated_at': '2019-11-27T06:27:53Z',
'closed_at': '2019-11-27T06:27:53Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/29',
'html_url': 'https://github.com/pysal/tobler/pull/29',
'diff_url': 'https://github.com/pysal/tobler/pull/29.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/29.patch'},
'body': 'Fixes #28 '},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/28',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/28/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/28/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/28/events',
'html_url': 'https://github.com/pysal/tobler/issues/28',
'id': 528990670,
'node_id': 'MDU6SXNzdWU1Mjg5OTA2NzA=',
'number': 28,
'title': 'quilt3 breakage',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1499380238,
'node_id': 'MDU6TGFiZWwxNDk5MzgwMjM4',
'url': 'https://api.github.com/repos/pysal/tobler/labels/bug',
'name': 'bug',
'color': 'd73a4a',
'default': True,
'description': "Something isn't working"}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-11-26T21:49:19Z',
'updated_at': '2019-11-27T06:27:53Z',
'closed_at': '2019-11-27T06:27:53Z',
'author_association': 'MEMBER',
'body': 'After following our [installation instructions](https://github.com/pysal/tobler/blob/master/README.md#installation), we are getting breakage:\r\n\r\n\r\n```\r\n~/Dropbox/t/tobler/github/tobler/tobler/data.py in <module>\r\n 1 from urllib.parse import unquote, urlparse\r\n 2 \r\n----> 3 import quilt3\r\n 4 \r\n 5 \r\n\r\n~/anaconda3/envs/tobler/lib/python3.7/site-packages/quilt3-3.1.5-py3.7.egg/quilt3/__init__.py in <module>\r\n 5 warnings.filterwarnings("ignore", message="numpy.dtype size changed")\r\n 6 \r\n----> 7 from .api import (\r\n 8 copy,\r\n 9 put,\r\n\r\n~/anaconda3/envs/tobler/lib/python3.7/site-packages/quilt3-3.1.5-py3.7.egg/quilt3/api.py in <module>\r\n 2 from urllib.parse import quote, urlparse, unquote\r\n 3 \r\n----> 4 from .data_transfer import copy_file, get_bytes, delete_url, put_bytes, list_url\r\n 5 from .formats import FormatRegistry\r\n 6 from .search_util import search_api\r\n\r\n~/anaconda3/envs/tobler/lib/python3.7/site-packages/quilt3-3.1.5-py3.7.egg/quilt3/data_transfer.py in <module>\r\n 20 from tqdm import tqdm\r\n 21 \r\n---> 22 from .session import create_botocore_session\r\n 23 from .util import QuiltException, make_s3_url, parse_file_url, parse_s3_url\r\n 24 from . import xattr\r\n\r\n~/anaconda3/envs/tobler/lib/python3.7/site-packages/quilt3-3.1.5-py3.7.egg/quilt3/session.py in <module>\r\n 20 AUTH_PATH = BASE_PATH / \'auth.json\'\r\n 21 CREDENTIALS_PATH = BASE_PATH / \'credentials.json\'\r\n---> 22 VERSION = pkg_resources.require(\'quilt3\')[0].version\r\n 23 \r\n 24 def _load_auth():\r\n\r\n~/anaconda3/envs/tobler/lib/python3.7/site-packages/pkg_resources/__init__.py in require(self, *requirements)\r\n 898 included, even if they were already activated in this working set.\r\n 899 """\r\n--> 900 needed = self.resolve(parse_requirements(requirements))\r\n 901 \r\n 902 for dist in needed:\r\n\r\n~/anaconda3/envs/tobler/lib/python3.7/site-packages/pkg_resources/__init__.py in resolve(self, requirements, env, installer, replace_conflicting, extras)\r\n 789 # Oops, the "best" so far conflicts with a dependency\r\n 790 dependent_req = required_by[req]\r\n--> 791 raise VersionConflict(dist, req).with_context(dependent_req)\r\n 792 \r\n 793 # push the new requirements onto the stack\r\n\r\nContextualVersionConflict: (python-dateutil 2.8.1 (/home/serge/anaconda3/envs/tobler/lib/python3.7/site-packages), Requirement.parse(\'python-dateutil<=2.8.0\'), {\'quilt3\'})\r\n```'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/26',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/26/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/26/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/26/events',
'html_url': 'https://github.com/pysal/tobler/pull/26',
'id': 520344409,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzM4OTQ4MzM1',
'number': 26,
'title': 'raise ioerror',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-11-09T06:36:22Z',
'updated_at': '2019-11-16T23:07:34Z',
'closed_at': '2019-11-16T23:07:34Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/26',
'html_url': 'https://github.com/pysal/tobler/pull/26',
'diff_url': 'https://github.com/pysal/tobler/pull/26.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/26.patch'},
'body': 'right now the error check doesnt actually raise an error. this resolves'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/24',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/24/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/24/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/24/events',
'html_url': 'https://github.com/pysal/tobler/pull/24',
'id': 488724455,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEzNjg3NTk1',
'number': 24,
'title': 'raise IOError if rasters are missing',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-09-03T17:12:28Z',
'updated_at': '2019-11-16T23:06:40Z',
'closed_at': '2019-11-16T23:06:40Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/24',
'html_url': 'https://github.com/pysal/tobler/pull/24',
'diff_url': 'https://github.com/pysal/tobler/pull/24.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/24.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/25',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/25/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/25/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/25/events',
'html_url': 'https://github.com/pysal/tobler/pull/25',
'id': 489448000,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzE0MjY0NTEy',
'number': 25,
'title': 'Rel01',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-09-04T23:15:43Z',
'updated_at': '2019-09-04T23:30:08Z',
'closed_at': '2019-09-04T23:30:07Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/25',
'html_url': 'https://github.com/pysal/tobler/pull/25',
'diff_url': 'https://github.com/pysal/tobler/pull/25.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/25.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/23',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/23/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/23/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/23/events',
'html_url': 'https://github.com/pysal/tobler/pull/23',
'id': 484640289,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwNDc2MzA2',
'number': 23,
'title': 'add xgboost approach in tests',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-08-23T17:42:26Z',
'updated_at': '2019-08-28T17:32:51Z',
'closed_at': '2019-08-28T17:32:51Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/23',
'html_url': 'https://github.com/pysal/tobler/pull/23',
'diff_url': 'https://github.com/pysal/tobler/pull/23.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/23.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/22',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/22/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/22/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/22/events',
'html_url': 'https://github.com/pysal/tobler/pull/22',
'id': 484626018,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwNDY0ODU5',
'number': 22,
'title': 'update readme image from harmonize notebook',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-23T17:02:21Z',
'updated_at': '2019-08-23T17:02:27Z',
'closed_at': '2019-08-23T17:02:27Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/22',
'html_url': 'https://github.com/pysal/tobler/pull/22',
'diff_url': 'https://github.com/pysal/tobler/pull/22.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/22.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/21',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/21/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/21/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/21/events',
'html_url': 'https://github.com/pysal/tobler/pull/21',
'id': 484250141,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwMTcwNzYx',
'number': 21,
'title': 'Add raster regression tests (also small tweak in a notebook)',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-08-22T22:44:27Z',
'updated_at': '2019-08-23T00:37:08Z',
'closed_at': '2019-08-23T00:37:07Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/21',
'html_url': 'https://github.com/pysal/tobler/pull/21',
'diff_url': 'https://github.com/pysal/tobler/pull/21.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/21.patch'},
'body': 'This adds the regression approach test for raster\r\nHopefully, this will work (it worked locally pretty fast with the additional step of tweaking the raster_path which is not present in this PR) and will improve significantly the coverage.'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/20',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/20/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/20/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/20/events',
'html_url': 'https://github.com/pysal/tobler/pull/20',
'id': 484241421,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwMTY0MDAw',
'number': 20,
'title': 'handle "file://" in raster path',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-22T22:14:45Z',
'updated_at': '2019-08-22T22:49:22Z',
'closed_at': '2019-08-22T22:49:22Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/20',
'html_url': 'https://github.com/pysal/tobler/pull/20',
'diff_url': 'https://github.com/pysal/tobler/pull/20.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/20.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/19',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/19/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/19/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/19/events',
'html_url': 'https://github.com/pysal/tobler/pull/19',
'id': 484196551,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwMTI2OTAx',
'number': 19,
'title': 'add quilt dependency',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-22T20:28:09Z',
'updated_at': '2019-08-22T20:28:19Z',
'closed_at': '2019-08-22T20:28:17Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/19',
'html_url': 'https://github.com/pysal/tobler/pull/19',
'diff_url': 'https://github.com/pysal/tobler/pull/19.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/19.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/18',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/18/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/18/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/18/events',
'html_url': 'https://github.com/pysal/tobler/pull/18',
'id': 484193677,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwMTI0ODY2',
'number': 18,
'title': 'extra reqs files',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-22T20:21:57Z',
'updated_at': '2019-08-22T20:22:19Z',
'closed_at': '2019-08-22T20:22:18Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/18',
'html_url': 'https://github.com/pysal/tobler/pull/18',
'diff_url': 'https://github.com/pysal/tobler/pull/18.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/18.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/17',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/17/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/17/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/17/events',
'html_url': 'https://github.com/pysal/tobler/pull/17',
'id': 484187806,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwMTIwMzMz',
'number': 17,
'title': 'update travis',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-22T20:08:25Z',
'updated_at': '2019-08-22T20:17:56Z',
'closed_at': '2019-08-22T20:17:54Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/17',
'html_url': 'https://github.com/pysal/tobler/pull/17',
'diff_url': 'https://github.com/pysal/tobler/pull/17.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/17.patch'},
'body': 'this conforms to the recently adopted pysal/geosnap conventions '},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/16',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/16/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/16/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/16/events',
'html_url': 'https://github.com/pysal/tobler/issues/16',
'id': 484094873,
'node_id': 'MDU6SXNzdWU0ODQwOTQ4NzM=',
'number': 16,
'title': 'turn on travis',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 2,
'created_at': '2019-08-22T16:25:39Z',
'updated_at': '2019-08-22T20:03:45Z',
'closed_at': '2019-08-22T20:03:45Z',
'author_association': 'MEMBER',
'body': 'I think @sjsrey needs to do it as owner'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/15',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/15/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/15/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/15/events',
'html_url': 'https://github.com/pysal/tobler/pull/15',
'id': 483701620,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA5NzI4NjUy',
'number': 15,
'title': 'swap to pytest tests',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-21T23:49:02Z',
'updated_at': '2019-08-21T23:49:10Z',
'closed_at': '2019-08-21T23:49:10Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/15',
'html_url': 'https://github.com/pysal/tobler/pull/15',
'diff_url': 'https://github.com/pysal/tobler/pull/15.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/15.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/14',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/14/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/14/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/14/events',
'html_url': 'https://github.com/pysal/tobler/pull/14',
'id': 483697575,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA5NzI1MzM3',
'number': 14,
'title': 'fix url and keywords from setup.py',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-21T23:31:23Z',
'updated_at': '2019-08-21T23:31:30Z',
'closed_at': '2019-08-21T23:31:30Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/14',
'html_url': 'https://github.com/pysal/tobler/pull/14',
'diff_url': 'https://github.com/pysal/tobler/pull/14.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/14.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/13',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/13/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/13/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/13/events',
'html_url': 'https://github.com/pysal/tobler/pull/13',
'id': 483693627,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA5NzIyMTUy',
'number': 13,
'title': 'tweak badge link',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-21T23:14:45Z',
'updated_at': '2019-08-21T23:14:52Z',
'closed_at': '2019-08-21T23:14:52Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/13',
'html_url': 'https://github.com/pysal/tobler/pull/13',
'diff_url': 'https://github.com/pysal/tobler/pull/13.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/13.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/12',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/12/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/12/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/12/events',
'html_url': 'https://github.com/pysal/tobler/pull/12',
'id': 483659313,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA5NjkzNzU2',
'number': 12,
'title': 'add build and coverage badges in readme',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-21T21:22:11Z',
'updated_at': '2019-08-21T23:09:48Z',
'closed_at': '2019-08-21T23:09:48Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/12',
'html_url': 'https://github.com/pysal/tobler/pull/12',
'diff_url': 'https://github.com/pysal/tobler/pull/12.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/12.patch'},
'body': 'Once coveralls is setup, this facilitates the addition of new tests.'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/7',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/7/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/7/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/7/events',
'html_url': 'https://github.com/pysal/tobler/pull/7',
'id': 483529583,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA5NTg3MTY3',
'number': 7,
'title': 'remove local paths',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-21T16:37:27Z',
'updated_at': '2019-08-21T16:37:33Z',
'closed_at': '2019-08-21T16:37:33Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/7',
'html_url': 'https://github.com/pysal/tobler/pull/7',
'diff_url': 'https://github.com/pysal/tobler/pull/7.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/7.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/5',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/5/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/5/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/5/events',
'html_url': 'https://github.com/pysal/tobler/pull/5',
'id': 482580492,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA4ODI1OTM2',
'number': 5,
'title': 'Drop scanlines (and harmonize) and improve notebooks and include tobler examples notebook (also adds tests and create travis file)',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-08-19T23:55:34Z',
'updated_at': '2019-08-20T21:50:51Z',
'closed_at': '2019-08-20T21:50:51Z',
'author_association': 'COLLABORATOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/5',
'html_url': 'https://github.com/pysal/tobler/pull/5',
'diff_url': 'https://github.com/pysal/tobler/pull/5.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/5.patch'},
'body': "Following the roadmap present in https://github.com/pysal/tobler/wiki/Release-Roadmap this PR pushes the first two points: `move scanlines from master` and `example notebooks with the approaches (actual data)`.\r\n\r\n**Sorry, I forgot to fork this repo and worked in this branch called `release_roadmap`. Once this PR is ok to be merged I'll fork it and work from my local GitHub repo, ok?**"},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/1',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/1/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/1/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/1/events',
'html_url': 'https://github.com/pysal/tobler/issues/1',
'id': 481394686,
'node_id': 'MDU6SXNzdWU0ODEzOTQ2ODY=',
'number': 1,
'title': 'put NLCD as default for raster data',
'user': {'login': 'renanxcortes',
'id': 22593188,
'node_id': 'MDQ6VXNlcjIyNTkzMTg4',
'avatar_url': 'https://avatars2.githubusercontent.com/u/22593188?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/renanxcortes',
'html_url': 'https://github.com/renanxcortes',
'followers_url': 'https://api.github.com/users/renanxcortes/followers',
'following_url': 'https://api.github.com/users/renanxcortes/following{/other_user}',
'gists_url': 'https://api.github.com/users/renanxcortes/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/renanxcortes/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/renanxcortes/subscriptions',
'organizations_url': 'https://api.github.com/users/renanxcortes/orgs',
'repos_url': 'https://api.github.com/users/renanxcortes/repos',
'events_url': 'https://api.github.com/users/renanxcortes/events{/privacy}',
'received_events_url': 'https://api.github.com/users/renanxcortes/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-08-16T00:43:40Z',
'updated_at': '2019-08-20T16:50:18Z',
'closed_at': '2019-08-20T16:50:18Z',
'author_association': 'COLLABORATOR',
'body': 'Currently, raster paths are `none`. We can put as default an online path of the S3 bucket of the NLCD raster available there.'},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/4',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/4/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/4/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/4/events',
'html_url': 'https://github.com/pysal/tobler/pull/4',
'id': 481776161,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA4MjEwNjg2',
'number': 4,
'title': 'default to nlcd data from quilt',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 2,
'created_at': '2019-08-16T20:36:50Z',
'updated_at': '2019-08-19T21:28:11Z',
'closed_at': '2019-08-19T21:28:11Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/4',
'html_url': 'https://github.com/pysal/tobler/pull/4',
'diff_url': 'https://github.com/pysal/tobler/pull/4.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/4.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/tobler/issues/3',
'repository_url': 'https://api.github.com/repos/pysal/tobler',
'labels_url': 'https://api.github.com/repos/pysal/tobler/issues/3/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/tobler/issues/3/comments',
'events_url': 'https://api.github.com/repos/pysal/tobler/issues/3/events',
'html_url': 'https://github.com/pysal/tobler/pull/3',
'id': 481776000,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzA4MjEwNTUy',
'number': 3,
'title': 'keep input crs',
'user': {'login': 'knaaptime',
'id': 4213368,
'node_id': 'MDQ6VXNlcjQyMTMzNjg=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/4213368?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/knaaptime',
'html_url': 'https://github.com/knaaptime',
'followers_url': 'https://api.github.com/users/knaaptime/followers',
'following_url': 'https://api.github.com/users/knaaptime/following{/other_user}',
'gists_url': 'https://api.github.com/users/knaaptime/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/knaaptime/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/knaaptime/subscriptions',
'organizations_url': 'https://api.github.com/users/knaaptime/orgs',
'repos_url': 'https://api.github.com/users/knaaptime/repos',
'events_url': 'https://api.github.com/users/knaaptime/events{/privacy}',
'received_events_url': 'https://api.github.com/users/knaaptime/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-16T20:36:24Z',
'updated_at': '2019-08-16T20:54:52Z',
'closed_at': '2019-08-16T20:54:50Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/tobler/pulls/3',
'html_url': 'https://github.com/pysal/tobler/pull/3',
'diff_url': 'https://github.com/pysal/tobler/pull/3.diff',
'patch_url': 'https://github.com/pysal/tobler/pull/3.patch'},
'body': ''}],
'mapclassify': [{'url': 'https://api.github.com/repos/pysal/mapclassify/issues/55',
'repository_url': 'https://api.github.com/repos/pysal/mapclassify',
'labels_url': 'https://api.github.com/repos/pysal/mapclassify/issues/55/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mapclassify/issues/55/comments',
'events_url': 'https://api.github.com/repos/pysal/mapclassify/issues/55/events',
'html_url': 'https://github.com/pysal/mapclassify/pull/55',
'id': 541364592,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU2MDI2OTM0',
'number': 55,
'title': 'REL: update changelog for release.',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-21T19:59:30Z',
'updated_at': '2020-01-02T19:24:43Z',
'closed_at': '2020-01-02T19:24:43Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/mapclassify/pulls/55',
'html_url': 'https://github.com/pysal/mapclassify/pull/55',
'diff_url': 'https://github.com/pysal/mapclassify/pull/55.diff',
'patch_url': 'https://github.com/pysal/mapclassify/pull/55.patch'},
'body': 'This is the final set of changes for the [2.2.0 release](https://github.com/pysal/mapclassify/releases/tag/untagged-f214146f708a54ba010f).'},
{'url': 'https://api.github.com/repos/pysal/mapclassify/issues/54',
'repository_url': 'https://api.github.com/repos/pysal/mapclassify',
'labels_url': 'https://api.github.com/repos/pysal/mapclassify/issues/54/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mapclassify/issues/54/comments',
'events_url': 'https://api.github.com/repos/pysal/mapclassify/issues/54/events',
'html_url': 'https://github.com/pysal/mapclassify/pull/54',
'id': 541357758,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU2MDIyMzIw',
'number': 54,
'title': '2.2',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-21T18:43:49Z',
'updated_at': '2019-12-21T18:52:31Z',
'closed_at': '2019-12-21T18:52:31Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/mapclassify/pulls/54',
'html_url': 'https://github.com/pysal/mapclassify/pull/54',
'diff_url': 'https://github.com/pysal/mapclassify/pull/54.diff',
'patch_url': 'https://github.com/pysal/mapclassify/pull/54.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/mapclassify/issues/53',
'repository_url': 'https://api.github.com/repos/pysal/mapclassify',
'labels_url': 'https://api.github.com/repos/pysal/mapclassify/issues/53/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mapclassify/issues/53/comments',
'events_url': 'https://api.github.com/repos/pysal/mapclassify/issues/53/events',
'html_url': 'https://github.com/pysal/mapclassify/pull/53',
'id': 541346026,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU2MDE0NDA5',
'number': 53,
'title': '2.2',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-12-21T16:43:03Z',
'updated_at': '2019-12-21T16:46:11Z',
'closed_at': '2019-12-21T16:46:11Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/mapclassify/pulls/53',
'html_url': 'https://github.com/pysal/mapclassify/pull/53',
'diff_url': 'https://github.com/pysal/mapclassify/pull/53.diff',
'patch_url': 'https://github.com/pysal/mapclassify/pull/53.patch'},
'body': ''},
{'url': 'https://api.github.com/repos/pysal/mapclassify/issues/52',
'repository_url': 'https://api.github.com/repos/pysal/mapclassify',
'labels_url': 'https://api.github.com/repos/pysal/mapclassify/issues/52/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mapclassify/issues/52/comments',
'events_url': 'https://api.github.com/repos/pysal/mapclassify/issues/52/events',
'html_url': 'https://github.com/pysal/mapclassify/issues/52',
'id': 522971496,
'node_id': 'MDU6SXNzdWU1MjI5NzE0OTY=',
'number': 52,
'title': 'conda-forge UnsatisfiableError on windows and python 3.7',
'user': {'login': 'martinfleis',
'id': 36797143,
'node_id': 'MDQ6VXNlcjM2Nzk3MTQz',
'avatar_url': 'https://avatars2.githubusercontent.com/u/36797143?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/martinfleis',
'html_url': 'https://github.com/martinfleis',
'followers_url': 'https://api.github.com/users/martinfleis/followers',
'following_url': 'https://api.github.com/users/martinfleis/following{/other_user}',
'gists_url': 'https://api.github.com/users/martinfleis/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/martinfleis/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/martinfleis/subscriptions',
'organizations_url': 'https://api.github.com/users/martinfleis/orgs',
'repos_url': 'https://api.github.com/users/martinfleis/repos',
'events_url': 'https://api.github.com/users/martinfleis/events{/privacy}',
'received_events_url': 'https://api.github.com/users/martinfleis/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-11-14T16:32:52Z',
'updated_at': '2019-11-14T16:45:29Z',
'closed_at': '2019-11-14T16:45:13Z',
'author_association': 'CONTRIBUTOR',
'body': "Hi,\r\n\r\nfor some reason, if I want to install mapclassify with python3.7 on windows from conda-forge, I'll get UnsatisfiableError (https://ci.appveyor.com/project/martinfleis/momepy/builds/28862313). In the environment is nothing else:\r\n\r\n```\r\nname: test\r\nchannels:\r\n - conda-forge\r\ndependencies:\r\n - python=3.7\r\n - mapclassify\r\n```\r\n \r\nIf I unpin python, I get python3.8 but mapclassify 2.0.1. So I sense the issue with scikit-learn, but I have no clue why this happens. The same situation is with pysal.\r\n\r\nEdit: If I keep mapclassify only, you get 2.0.1, if I pin mapclassify to 2.1.1 I get the error."},
{'url': 'https://api.github.com/repos/pysal/mapclassify/issues/49',
'repository_url': 'https://api.github.com/repos/pysal/mapclassify',
'labels_url': 'https://api.github.com/repos/pysal/mapclassify/issues/49/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mapclassify/issues/49/comments',
'events_url': 'https://api.github.com/repos/pysal/mapclassify/issues/49/events',
'html_url': 'https://github.com/pysal/mapclassify/pull/49',
'id': 508159081,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI5MDA3MzM2',
'number': 49,
'title': '[MAINT] updating supported Python versions in setup.py',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1621138649,
'node_id': 'MDU6TGFiZWwxNjIxMTM4NjQ5',
'url': 'https://api.github.com/repos/pysal/mapclassify/labels/maintenance',
'name': 'maintenance',
'color': 'f4dc86',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-10-16T23:59:15Z',
'updated_at': '2019-10-17T11:58:07Z',
'closed_at': '2019-10-17T02:17:29Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/mapclassify/pulls/49',
'html_url': 'https://github.com/pysal/mapclassify/pull/49',
'diff_url': 'https://github.com/pysal/mapclassify/pull/49.diff',
'patch_url': 'https://github.com/pysal/mapclassify/pull/49.patch'},
'body': 'Updating the currently supported Python versions in `setup.py` from `3.5` & `3.6` to `3.6` & `3.7`, as tested in [`.travis.yml`](https://github.com/pysal/mapclassify/blob/master/.travis.yml).'},
{'url': 'https://api.github.com/repos/pysal/mapclassify/issues/46',
'repository_url': 'https://api.github.com/repos/pysal/mapclassify',
'labels_url': 'https://api.github.com/repos/pysal/mapclassify/issues/46/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mapclassify/issues/46/comments',
'events_url': 'https://api.github.com/repos/pysal/mapclassify/issues/46/events',
'html_url': 'https://github.com/pysal/mapclassify/pull/46',
'id': 491877705,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzE2MTYwOTI2',
'number': 46,
'title': 'BUG: RecursiveError in HeadTailBreaks',
'user': {'login': 'martinfleis',
'id': 36797143,
'node_id': 'MDQ6VXNlcjM2Nzk3MTQz',
'avatar_url': 'https://avatars2.githubusercontent.com/u/36797143?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/martinfleis',
'html_url': 'https://github.com/martinfleis',
'followers_url': 'https://api.github.com/users/martinfleis/followers',
'following_url': 'https://api.github.com/users/martinfleis/following{/other_user}',
'gists_url': 'https://api.github.com/users/martinfleis/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/martinfleis/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/martinfleis/subscriptions',
'organizations_url': 'https://api.github.com/users/martinfleis/orgs',
'repos_url': 'https://api.github.com/users/martinfleis/repos',
'events_url': 'https://api.github.com/users/martinfleis/events{/privacy}',
'received_events_url': 'https://api.github.com/users/martinfleis/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 588432236,
'node_id': 'MDU6TGFiZWw1ODg0MzIyMzY=',
'url': 'https://api.github.com/repos/pysal/mapclassify/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 2,
'created_at': '2019-09-10T20:21:00Z',
'updated_at': '2019-09-10T22:44:20Z',
'closed_at': '2019-09-10T22:44:20Z',
'author_association': 'CONTRIBUTOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/mapclassify/pulls/46',
'html_url': 'https://github.com/pysal/mapclassify/pull/46',
'diff_url': 'https://github.com/pysal/mapclassify/pull/46.diff',
'patch_url': 'https://github.com/pysal/mapclassify/pull/46.patch'},
'body': 'Fixes #45 \r\n\r\nImplemented fix suggested by @weikang9009 in #45, including test. \r\n\r\nIn case of multiple identical maximum values, HeadTailBreak no longer raises RecursiveError but ends its search, having multiple values in the last bin (opposed to expected 1 value). '},
{'url': 'https://api.github.com/repos/pysal/mapclassify/issues/45',
'repository_url': 'https://api.github.com/repos/pysal/mapclassify',
'labels_url': 'https://api.github.com/repos/pysal/mapclassify/issues/45/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mapclassify/issues/45/comments',
'events_url': 'https://api.github.com/repos/pysal/mapclassify/issues/45/events',
'html_url': 'https://github.com/pysal/mapclassify/issues/45',
'id': 491718523,
'node_id': 'MDU6SXNzdWU0OTE3MTg1MjM=',
'number': 45,
'title': 'BUG: HeadTailBreaks raise RecursionError',
'user': {'login': 'martinfleis',
'id': 36797143,
'node_id': 'MDQ6VXNlcjM2Nzk3MTQz',
'avatar_url': 'https://avatars2.githubusercontent.com/u/36797143?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/martinfleis',
'html_url': 'https://github.com/martinfleis',
'followers_url': 'https://api.github.com/users/martinfleis/followers',
'following_url': 'https://api.github.com/users/martinfleis/following{/other_user}',
'gists_url': 'https://api.github.com/users/martinfleis/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/martinfleis/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/martinfleis/subscriptions',
'organizations_url': 'https://api.github.com/users/martinfleis/orgs',
'repos_url': 'https://api.github.com/users/martinfleis/repos',
'events_url': 'https://api.github.com/users/martinfleis/events{/privacy}',
'received_events_url': 'https://api.github.com/users/martinfleis/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-09-10T14:42:36Z',
'updated_at': '2019-09-10T22:44:19Z',
'closed_at': '2019-09-10T22:44:19Z',
'author_association': 'CONTRIBUTOR',
'body': 'HeadTailBreaks raises RecursionError if the maximum value within data is there twice (or more). Then it simply locks itself in the loop in `values[values >= mean]` - mean will be always the same and both values will always returned.\r\n\r\nSteps to reproduce:\r\n```\r\ndata = np.random.pareto(2, 1000)\r\ndata = np.append(data, data.max())\r\n\r\nmc.HeadTailBreaks(data)\r\n```\r\n\r\nI assume that once there are only the same values within remaining values, `head_tail_breaks` should stop:\r\n\r\n```\r\ndef head_tail_breaks(values, cuts):\r\n """\r\n head tail breaks helper function\r\n """\r\n values = np.array(values)\r\n mean = np.mean(values)\r\n cuts.append(mean)\r\n if len(values) > 1:\r\n if len(set(values)) > 1: #this seems to fix the issue\r\n return head_tail_breaks(values[values >= mean], cuts)\r\n return cuts\r\n```\r\n\r\nHowever, I am not sure if it is the intended behaviour to stop and keep multiple values in the last bin as it does not reflect the definition of HeadTailBreaks algorithm (but I cannot see another solution). Happy to do a PR if this is how you want to fix that.'},
{'url': 'https://api.github.com/repos/pysal/mapclassify/issues/47',
'repository_url': 'https://api.github.com/repos/pysal/mapclassify',
'labels_url': 'https://api.github.com/repos/pysal/mapclassify/issues/47/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mapclassify/issues/47/comments',
'events_url': 'https://api.github.com/repos/pysal/mapclassify/issues/47/events',
'html_url': 'https://github.com/pysal/mapclassify/pull/47',
'id': 491896699,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzE2MTc2NzQ2',
'number': 47,
'title': 'BUG: UserDefined accepts only list if max not in bins',
'user': {'login': 'martinfleis',
'id': 36797143,
'node_id': 'MDQ6VXNlcjM2Nzk3MTQz',
'avatar_url': 'https://avatars2.githubusercontent.com/u/36797143?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/martinfleis',
'html_url': 'https://github.com/martinfleis',
'followers_url': 'https://api.github.com/users/martinfleis/followers',
'following_url': 'https://api.github.com/users/martinfleis/following{/other_user}',
'gists_url': 'https://api.github.com/users/martinfleis/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/martinfleis/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/martinfleis/subscriptions',
'organizations_url': 'https://api.github.com/users/martinfleis/orgs',
'repos_url': 'https://api.github.com/users/martinfleis/repos',
'events_url': 'https://api.github.com/users/martinfleis/events{/privacy}',
'received_events_url': 'https://api.github.com/users/martinfleis/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 588432236,
'node_id': 'MDU6TGFiZWw1ODg0MzIyMzY=',
'url': 'https://api.github.com/repos/pysal/mapclassify/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-09-10T21:02:42Z',
'updated_at': '2019-09-10T22:01:54Z',
'closed_at': '2019-09-10T22:01:54Z',
'author_association': 'CONTRIBUTOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/mapclassify/pulls/47',
'html_url': 'https://github.com/pysal/mapclassify/pull/47',
'diff_url': 'https://github.com/pysal/mapclassify/pull/47.diff',
'patch_url': 'https://github.com/pysal/mapclassify/pull/47.patch'},
'body': "UserDefined attempts to add max value of given array to bins if that is not covered by given bins. However, it expects that bins are a list (`bins.append(max(y))`), while it could be any array-like. Even mapclassify's own `self.bins` gives array. I have changed this behaviour using np.append to cover all possibilities. \r\n\r\nSteps to replicate the issue:\r\n\r\n```py\r\nx = list(range(1, 1000))\r\ny = list(range(1, 500))\r\nnb = mc.NaturalBreaks(y)\r\nuserdefined = mc.UserDefined(x, nb.bins)\r\n```\r\n\r\n_(That's all from me today, I just happened to work with mapclassify a lot today and found these simple issues. Thank you for this great package!)_"},
{'url': 'https://api.github.com/repos/pysal/mapclassify/issues/44',
'repository_url': 'https://api.github.com/repos/pysal/mapclassify',
'labels_url': 'https://api.github.com/repos/pysal/mapclassify/issues/44/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mapclassify/issues/44/comments',
'events_url': 'https://api.github.com/repos/pysal/mapclassify/issues/44/events',
'html_url': 'https://github.com/pysal/mapclassify/pull/44',
'id': 491692643,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzE2MDEwNDQx',
'number': 44,
'title': 'BUG: avoid deprecation warning in HeadTailBreaks',
'user': {'login': 'martinfleis',
'id': 36797143,
'node_id': 'MDQ6VXNlcjM2Nzk3MTQz',
'avatar_url': 'https://avatars2.githubusercontent.com/u/36797143?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/martinfleis',
'html_url': 'https://github.com/martinfleis',
'followers_url': 'https://api.github.com/users/martinfleis/followers',
'following_url': 'https://api.github.com/users/martinfleis/following{/other_user}',
'gists_url': 'https://api.github.com/users/martinfleis/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/martinfleis/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/martinfleis/subscriptions',
'organizations_url': 'https://api.github.com/users/martinfleis/orgs',
'repos_url': 'https://api.github.com/users/martinfleis/repos',
'events_url': 'https://api.github.com/users/martinfleis/events{/privacy}',
'received_events_url': 'https://api.github.com/users/martinfleis/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 588432236,
'node_id': 'MDU6TGFiZWw1ODg0MzIyMzY=',
'url': 'https://api.github.com/repos/pysal/mapclassify/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 1,
'created_at': '2019-09-10T14:01:10Z',
'updated_at': '2019-09-10T19:24:36Z',
'closed_at': '2019-09-10T17:21:47Z',
'author_association': 'CONTRIBUTOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/mapclassify/pulls/44',
'html_url': 'https://github.com/pysal/mapclassify/pull/44',
'diff_url': 'https://github.com/pysal/mapclassify/pull/44.diff',
'patch_url': 'https://github.com/pysal/mapclassify/pull/44.patch'},
'body': "After recent changes of classifiers' names, HeadTailBreaks returns DeprecationWarning even if a new name if used, because it uses the old one in the function itself. That is changed to use the new name.\r\n\r\nTo reproduce call HeadTailBreaks on any data.\r\n\r\n```\r\ndata = [1, 1, 1, 4, 5, 10]\r\nmc.HeadTailBreaks(data)\r\n```\r\n```\r\n/Users/martin/anaconda3/envs/geo_dev/lib/python3.7/site-packages/mapclassify/classifiers.py:86: DeprecationWarning: Call to deprecated function (or staticmethod) headTail_breaks. (use head_tail_breaks)\r\n return headTail_breaks(values[values >= mean], cuts)\r\n```"},
{'url': 'https://api.github.com/repos/pysal/mapclassify/issues/42',
'repository_url': 'https://api.github.com/repos/pysal/mapclassify',
'labels_url': 'https://api.github.com/repos/pysal/mapclassify/issues/42/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mapclassify/issues/42/comments',
'events_url': 'https://api.github.com/repos/pysal/mapclassify/issues/42/events',
'html_url': 'https://github.com/pysal/mapclassify/issues/42',
'id': 484968052,
'node_id': 'MDU6SXNzdWU0ODQ5NjgwNTI=',
'number': 42,
'title': 'remove docs badge',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-08-25T19:41:53Z',
'updated_at': '2019-09-01T19:59:28Z',
'closed_at': '2019-09-01T19:59:28Z',
'author_association': 'MEMBER',
'body': 'Since `mapclassify` switched from `readthedocs` to GitHub hosted docs (#41) the badges on [`README.md`](https://github.com/pysal/mapclassify/blame/master/README.md#L7) and [`README.rst `](https://github.com/pysal/mapclassify/blame/master/README.rst#L10) no longer functional or necessary.\r\n\r\n- [x] remove doc badge from `README.md`\r\n- [x] remove doc badge from `README.rst`'},
{'url': 'https://api.github.com/repos/pysal/mapclassify/issues/43',
'repository_url': 'https://api.github.com/repos/pysal/mapclassify',
'labels_url': 'https://api.github.com/repos/pysal/mapclassify/issues/43/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mapclassify/issues/43/comments',
'events_url': 'https://api.github.com/repos/pysal/mapclassify/issues/43/events',
'html_url': 'https://github.com/pysal/mapclassify/pull/43',
'id': 484968564,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzEwNzA5ODM3',
'number': 43,
'title': 'Remove doc badge',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-08-25T19:47:56Z',
'updated_at': '2020-01-04T21:14:43Z',
'closed_at': '2019-09-01T15:59:46Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/mapclassify/pulls/43',
'html_url': 'https://github.com/pysal/mapclassify/pull/43',
'diff_url': 'https://github.com/pysal/mapclassify/pull/43.diff',
'patch_url': 'https://github.com/pysal/mapclassify/pull/43.patch'},
'body': 'Addressing #42 due to #41 '},
{'url': 'https://api.github.com/repos/pysal/mapclassify/issues/41',
'repository_url': 'https://api.github.com/repos/pysal/mapclassify',
'labels_url': 'https://api.github.com/repos/pysal/mapclassify/issues/41/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/mapclassify/issues/41/comments',
'events_url': 'https://api.github.com/repos/pysal/mapclassify/issues/41/events',
'html_url': 'https://github.com/pysal/mapclassify/pull/41',
'id': 475443029,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzAzMTk1MDY1',
'number': 41,
'title': 'Docs: moving to project pages on github and off rtd',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-08-01T02:50:46Z',
'updated_at': '2019-08-01T02:53:42Z',
'closed_at': '2019-08-01T02:53:42Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/mapclassify/pulls/41',
'html_url': 'https://github.com/pysal/mapclassify/pull/41',
'diff_url': 'https://github.com/pysal/mapclassify/pull/41.diff',
'patch_url': 'https://github.com/pysal/mapclassify/pull/41.patch'},
'body': ''}],
'splot': [{'url': 'https://api.github.com/repos/pysal/splot/issues/98',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/98/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/98/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/98/events',
'html_url': 'https://github.com/pysal/splot/pull/98',
'id': 551821038,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzY0NDYyOTQw',
'number': 98,
'title': 'Release prep for 1.1.2',
'user': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-18T20:38:36Z',
'updated_at': '2020-01-18T20:44:22Z',
'closed_at': '2020-01-18T20:44:22Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/splot/pulls/98',
'html_url': 'https://github.com/pysal/splot/pull/98',
'diff_url': 'https://github.com/pysal/splot/pull/98.diff',
'patch_url': 'https://github.com/pysal/splot/pull/98.patch'},
'body': '* remove geopandas restrictions from `requirements.txt` due to newest geopandas release\r\n* change version nr to 1.1.2 in `__init__.py` and `setup.py`\r\n* add release notes to `CHANGELOG.md`'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/88',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/88/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/88/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/88/events',
'html_url': 'https://github.com/pysal/splot/issues/88',
'id': 522452767,
'node_id': 'MDU6SXNzdWU1MjI0NTI3Njc=',
'number': 88,
'title': 'Installation instructions; pip install fails on macOS',
'user': {'login': 'martinfleis',
'id': 36797143,
'node_id': 'MDQ6VXNlcjM2Nzk3MTQz',
'avatar_url': 'https://avatars2.githubusercontent.com/u/36797143?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/martinfleis',
'html_url': 'https://github.com/martinfleis',
'followers_url': 'https://api.github.com/users/martinfleis/followers',
'following_url': 'https://api.github.com/users/martinfleis/following{/other_user}',
'gists_url': 'https://api.github.com/users/martinfleis/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/martinfleis/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/martinfleis/subscriptions',
'organizations_url': 'https://api.github.com/users/martinfleis/orgs',
'repos_url': 'https://api.github.com/users/martinfleis/repos',
'events_url': 'https://api.github.com/users/martinfleis/events{/privacy}',
'received_events_url': 'https://api.github.com/users/martinfleis/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1639142764,
'node_id': 'MDU6TGFiZWwxNjM5MTQyNzY0',
'url': 'https://api.github.com/repos/pysal/splot/labels/JOSS%20paper',
'name': 'JOSS paper',
'color': 'bfd4f2',
'default': False,
'description': ''},
{'id': 1353562993,
'node_id': 'MDU6TGFiZWwxMzUzNTYyOTkz',
'url': 'https://api.github.com/repos/pysal/splot/labels/priority:%20high',
'name': 'priority: high',
'color': 'FF5733',
'default': False,
'description': 'needs to be resolved soon'}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/splot/milestones/1',
'html_url': 'https://github.com/pysal/splot/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/splot/milestones/1/labels',
'id': 4298019,
'node_id': 'MDk6TWlsZXN0b25lNDI5ODAxOQ==',
'number': 1,
'title': 'JOSS paper',
'description': 'submit splot as JOSS article',
'creator': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 2,
'closed_issues': 16,
'state': 'open',
'created_at': '2019-05-08T23:06:19Z',
'updated_at': '2020-01-18T20:24:38Z',
'due_on': '2019-07-01T07:00:00Z',
'closed_at': None},
'comments': 3,
'created_at': '2019-11-13T20:23:50Z',
'updated_at': '2020-01-18T20:24:38Z',
'closed_at': '2020-01-18T20:24:38Z',
'author_association': 'CONTRIBUTOR',
'body': "Hi,\r\n\r\nas part of https://github.com/openjournals/joss-reviews/issues/1882 I am trying to simply follow your installation docs and initially I did not succeed. (under macOS 10.15)\r\n\r\n```\r\nconda create -n splot python\r\nconda activate splot\r\npip install splot\r\n```\r\n\r\n```\r\n...\r\nCollecting pyproj\r\n Downloading https://files.pythonhosted.org/packages/8c/7e/c34acb5abca1916ba0a294df4a90935d6d1cfc446ad1c076986bbf0118d3/pyproj-2.4.1.tar.gz (462kB)\r\n |████████████████████████████████| 471kB 2.8MB/s \r\n Installing build dependencies ... done\r\n Getting requirements to build wheel ... error\r\n ERROR: Command errored out with exit status 1:\r\n command: /Users/martin/anaconda3/envs/splot/bin/python /Users/martin/anaconda3/envs/splot/lib/python3.8/site-packages/pip/_vendor/pep517/_in_process.py get_requires_for_build_wheel /var/folders/d0/qyf0yvzd0n9ctn2wr3r4l1vw0000gn/T/tmp889iudeu\r\n cwd: /private/var/folders/d0/qyf0yvzd0n9ctn2wr3r4l1vw0000gn/T/pip-install-4pkixl3a/pyproj\r\n Complete output (1 lines):\r\n ERROR: Minimum supported proj version is 6.2.0, installed version is 5.2.0.\r\n ----------------------------------------\r\nERROR: Command errored out with exit status 1: /Users/martin/anaconda3/envs/splot/bin/python /Users/martin/anaconda3/envs/splot/lib/python3.8/site-packages/pip/_vendor/pep517/_in_process.py get_requires_for_build_wheel /var/folders/d0/qyf0yvzd0n9ctn2wr3r4l1vw0000gn/T/tmp889iudeu Check the logs for full command output.\r\n```\r\n\r\nI am aware that you are saying that it is compatible with 3.5 and 3.6 only (why? because of this issue?). Using `conda create -n splot python=3.6` it goes well. But it can be confusing.\r\n\r\nI am perfectly aware that this is not `splot` issue, but `pyproj`, being dependency of GeoPandas. As installation of `geopandas` from `pip` is notoriously problematic (http://geopandas.org/install.html), I would welcome a bit more information in your docs what to do. I can install `geopandas` from `conda-forge` and then `splot` from `pip`, which works perfectly, but this information could be in the documentation.\r\n\r\nI see that splot is on conda-forge in the same version as on PyPI, why you don't mention this option as well? `conda install -c conda-forge splot` works smoothly.\r\n\r\nI would recommend adding `conda-forge` option to documentation and link to` geopandas` docs for `pip` troubleshooting (as the issue is caused by `geopandas`).\r\n\r\nAlso, I do not understand '_splot supports python 3.5 and 3.6 only_' note as from conda-forge it comes with 3.8."},
{'url': 'https://api.github.com/repos/pysal/splot/issues/90',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/90/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/90/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/90/events',
'html_url': 'https://github.com/pysal/splot/issues/90',
'id': 522492928,
'node_id': 'MDU6SXNzdWU1MjI0OTI5Mjg=',
'number': 90,
'title': 'Usage in readme is a fragment',
'user': {'login': 'martinfleis',
'id': 36797143,
'node_id': 'MDQ6VXNlcjM2Nzk3MTQz',
'avatar_url': 'https://avatars2.githubusercontent.com/u/36797143?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/martinfleis',
'html_url': 'https://github.com/martinfleis',
'followers_url': 'https://api.github.com/users/martinfleis/followers',
'following_url': 'https://api.github.com/users/martinfleis/following{/other_user}',
'gists_url': 'https://api.github.com/users/martinfleis/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/martinfleis/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/martinfleis/subscriptions',
'organizations_url': 'https://api.github.com/users/martinfleis/orgs',
'repos_url': 'https://api.github.com/users/martinfleis/repos',
'events_url': 'https://api.github.com/users/martinfleis/events{/privacy}',
'received_events_url': 'https://api.github.com/users/martinfleis/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1639142764,
'node_id': 'MDU6TGFiZWwxNjM5MTQyNzY0',
'url': 'https://api.github.com/repos/pysal/splot/labels/JOSS%20paper',
'name': 'JOSS paper',
'color': 'bfd4f2',
'default': False,
'description': ''},
{'id': 1353562993,
'node_id': 'MDU6TGFiZWwxMzUzNTYyOTkz',
'url': 'https://api.github.com/repos/pysal/splot/labels/priority:%20high',
'name': 'priority: high',
'color': 'FF5733',
'default': False,
'description': 'needs to be resolved soon'}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/splot/milestones/1',
'html_url': 'https://github.com/pysal/splot/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/splot/milestones/1/labels',
'id': 4298019,
'node_id': 'MDk6TWlsZXN0b25lNDI5ODAxOQ==',
'number': 1,
'title': 'JOSS paper',
'description': 'submit splot as JOSS article',
'creator': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 2,
'closed_issues': 16,
'state': 'open',
'created_at': '2019-05-08T23:06:19Z',
'updated_at': '2020-01-18T20:24:38Z',
'due_on': '2019-07-01T07:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2019-11-13T21:50:18Z',
'updated_at': '2020-01-18T20:24:09Z',
'closed_at': '2020-01-18T20:24:08Z',
'author_association': 'CONTRIBUTOR',
'body': 'Hi,\r\n\r\nthis is a very minor thing, but Usage section in the readme is a strange fragment only. \r\n\r\n> splot supports many different https://github.com/pysal/splot/blob/master/notebooks/mapping_vba.ipynb\r\n\r\nI would assume that it points me to https://github.com/pysal/splot/tree/master/notebooks, or that is not the intention with examples?\r\n\r\ncc openjournals/joss-reviews#1882'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/92',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/92/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/92/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/92/events',
'html_url': 'https://github.com/pysal/splot/issues/92',
'id': 524004399,
'node_id': 'MDU6SXNzdWU1MjQwMDQzOTk=',
'number': 92,
'title': 'JOSS: missing figure captions',
'user': {'login': 'martinfleis',
'id': 36797143,
'node_id': 'MDQ6VXNlcjM2Nzk3MTQz',
'avatar_url': 'https://avatars2.githubusercontent.com/u/36797143?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/martinfleis',
'html_url': 'https://github.com/martinfleis',
'followers_url': 'https://api.github.com/users/martinfleis/followers',
'following_url': 'https://api.github.com/users/martinfleis/following{/other_user}',
'gists_url': 'https://api.github.com/users/martinfleis/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/martinfleis/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/martinfleis/subscriptions',
'organizations_url': 'https://api.github.com/users/martinfleis/orgs',
'repos_url': 'https://api.github.com/users/martinfleis/repos',
'events_url': 'https://api.github.com/users/martinfleis/events{/privacy}',
'received_events_url': 'https://api.github.com/users/martinfleis/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1639142764,
'node_id': 'MDU6TGFiZWwxNjM5MTQyNzY0',
'url': 'https://api.github.com/repos/pysal/splot/labels/JOSS%20paper',
'name': 'JOSS paper',
'color': 'bfd4f2',
'default': False,
'description': ''},
{'id': 1353562993,
'node_id': 'MDU6TGFiZWwxMzUzNTYyOTkz',
'url': 'https://api.github.com/repos/pysal/splot/labels/priority:%20high',
'name': 'priority: high',
'color': 'FF5733',
'default': False,
'description': 'needs to be resolved soon'}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/splot/milestones/1',
'html_url': 'https://github.com/pysal/splot/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/splot/milestones/1/labels',
'id': 4298019,
'node_id': 'MDk6TWlsZXN0b25lNDI5ODAxOQ==',
'number': 1,
'title': 'JOSS paper',
'description': 'submit splot as JOSS article',
'creator': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 2,
'closed_issues': 16,
'state': 'open',
'created_at': '2019-05-08T23:06:19Z',
'updated_at': '2020-01-18T20:24:38Z',
'due_on': '2019-07-01T07:00:00Z',
'closed_at': None},
'comments': 1,
'created_at': '2019-11-17T15:50:19Z',
'updated_at': '2020-01-18T20:23:51Z',
'closed_at': '2020-01-18T20:23:50Z',
'author_association': 'CONTRIBUTOR',
'body': 'Hi,\r\nI believe this is the last issue I am opening as a part of https://github.com/openjournals/joss-reviews/issues/1882. \r\n\r\nFigures in you paper are missing captions. I see that they are in the `paper.md`, but they did not show up in pdf. I guess it is an issue with markdown syntax. Can you check that?'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/96',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/96/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/96/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/96/events',
'html_url': 'https://github.com/pysal/splot/pull/96',
'id': 546592954,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzYwMjQxNDI0',
'number': 96,
'title': '[DOC] update installation instruction',
'user': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1639142764,
'node_id': 'MDU6TGFiZWwxNjM5MTQyNzY0',
'url': 'https://api.github.com/repos/pysal/splot/labels/JOSS%20paper',
'name': 'JOSS paper',
'color': 'bfd4f2',
'default': False,
'description': ''},
{'id': 1353562993,
'node_id': 'MDU6TGFiZWwxMzUzNTYyOTkz',
'url': 'https://api.github.com/repos/pysal/splot/labels/priority:%20high',
'name': 'priority: high',
'color': 'FF5733',
'default': False,
'description': 'needs to be resolved soon'}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-08T01:21:30Z',
'updated_at': '2020-01-18T19:41:14Z',
'closed_at': '2020-01-18T19:41:14Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/splot/pulls/96',
'html_url': 'https://github.com/pysal/splot/pull/96',
'diff_url': 'https://github.com/pysal/splot/pull/96.diff',
'patch_url': 'https://github.com/pysal/splot/pull/96.patch'},
'body': 'Update of installation instructions in documentation\r\n\r\n* adding information about dependencies\r\n* informing users of ways of trouble shooting\r\n* including conda-forge installation instructions\r\n\r\naddresses #88 \r\n\r\n'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/97',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/97/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/97/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/97/events',
'html_url': 'https://github.com/pysal/splot/pull/97',
'id': 551122022,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzYzOTAxNzk1',
'number': 97,
'title': '[DOC] add example links to README.md & figure captions in joss article',
'user': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1639142764,
'node_id': 'MDU6TGFiZWwxNjM5MTQyNzY0',
'url': 'https://api.github.com/repos/pysal/splot/labels/JOSS%20paper',
'name': 'JOSS paper',
'color': 'bfd4f2',
'default': False,
'description': ''},
{'id': 1353562993,
'node_id': 'MDU6TGFiZWwxMzUzNTYyOTkz',
'url': 'https://api.github.com/repos/pysal/splot/labels/priority:%20high',
'name': 'priority: high',
'color': 'FF5733',
'default': False,
'description': 'needs to be resolved soon'}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-16T23:56:03Z',
'updated_at': '2020-01-18T19:41:12Z',
'closed_at': '2020-01-18T19:41:12Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/splot/pulls/97',
'html_url': 'https://github.com/pysal/splot/pull/97',
'diff_url': 'https://github.com/pysal/splot/pull/97.diff',
'patch_url': 'https://github.com/pysal/splot/pull/97.patch'},
'body': 'This PR builds on top of #96 and addresses #90 and #92 \r\n\r\n* add figure captions in `paper.md` through markdown italics formatting\r\n* add links of notebook examples and other material to `README.md` under `Usage` section\r\n* add script lightning talk as documentation under introduction in `README.md`'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/83',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/83/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/83/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/83/events',
'html_url': 'https://github.com/pysal/splot/issues/83',
'id': 502316101,
'node_id': 'MDU6SXNzdWU1MDIzMTYxMDE=',
'number': 83,
'title': '[BUG] vba_choropleth failure',
'user': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 9,
'created_at': '2019-10-03T21:48:52Z',
'updated_at': '2020-01-17T20:38:21Z',
'closed_at': '2020-01-17T20:38:20Z',
'author_association': 'MEMBER',
'body': 'standard run of VBA_choropleth currently failing:\r\n\r\n```---------------------------------------------------------------------------\r\nValueError Traceback (most recent call last)\r\n<ipython-input-5-e2e864f55e9b> in <module>\r\n 8 vba_choropleth(x, y, gdf, rgb_mapclassify=dict(classifier=\'quantiles\'),\r\n 9 alpha_mapclassify=dict(classifier=\'quantiles\'),\r\n---> 10 cmap=\'RdBu\', ax=axs[1])\r\n 11 \r\n 12 # set figure style\r\n\r\n~/code/splot/splot/_viz_value_by_alpha_mpl.py in vba_choropleth(x_var, y_var, gdf, cmap, divergent, revert_alpha, alpha_mapclassify, rgb_mapclassify, ax, legend)\r\n 285 divergent=divergent,\r\n 286 revert_alpha=revert_alpha)\r\n--> 287 gdf.plot(color=rgba, ax=ax)\r\n 288 ax.set_axis_off()\r\n 289 ax.set_aspect(\'equal\')\r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/geopandas/geodataframe.py in plot(self, *args, **kwargs)\r\n 604 from there.\r\n 605 """\r\n--> 606 return plot_dataframe(self, *args, **kwargs)\r\n 607 \r\n 608 plot.__doc__ = plot_dataframe.__doc__\r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/geopandas/plotting.py in plot_dataframe(df, column, cmap, color, ax, cax, categorical, legend, scheme, k, vmin, vmax, markersize, figsize, legend_kwds, classification_kwds, **style_kwds)\r\n 500 figsize=figsize,\r\n 501 markersize=markersize,\r\n--> 502 **style_kwds\r\n 503 )\r\n 504 \r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/geopandas/plotting.py in plot_series(s, cmap, color, ax, figsize, **style_kwds)\r\n 339 values_ = values[poly_idx] if cmap else None\r\n 340 plot_polygon_collection(\r\n--> 341 ax, polys, values_, facecolor=facecolor, cmap=cmap, **style_kwds\r\n 342 )\r\n 343 \r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/geopandas/plotting.py in plot_polygon_collection(ax, geoms, values, color, cmap, vmin, vmax, **kwargs)\r\n 111 kwargs[att] = np.take(kwargs[att], multiindex)\r\n 112 \r\n--> 113 collection = PatchCollection([PolygonPatch(poly) for poly in geoms], **kwargs)\r\n 114 \r\n 115 if values is not None:\r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/matplotlib/collections.py in __init__(self, patches, match_original, **kwargs)\r\n 1803 kwargs[\'antialiaseds\'] = [p.get_antialiased() for p in patches]\r\n 1804 \r\n-> 1805 Collection.__init__(self, **kwargs)\r\n 1806 \r\n 1807 self.set_paths(patches)\r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/matplotlib/collections.py in __init__(self, edgecolors, facecolors, linewidths, linestyles, capstyle, joinstyle, antialiaseds, offsets, transOffset, norm, cmap, pickradius, hatch, urls, offset_position, zorder, **kwargs)\r\n 160 \r\n 161 self._path_effects = None\r\n--> 162 self.update(kwargs)\r\n 163 self._paths = None\r\n 164 \r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/matplotlib/artist.py in update(self, props)\r\n 972 \r\n 973 with cbook._setattr_cm(self, eventson=False):\r\n--> 974 ret = [_update_property(self, k, v) for k, v in props.items()]\r\n 975 \r\n 976 if len(ret):\r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/matplotlib/artist.py in <listcomp>(.0)\r\n 972 \r\n 973 with cbook._setattr_cm(self, eventson=False):\r\n--> 974 ret = [_update_property(self, k, v) for k, v in props.items()]\r\n 975 \r\n 976 if len(ret):\r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/matplotlib/artist.py in _update_property(self, k, v)\r\n 969 raise AttributeError(\'{!r} object has no property {!r}\'\r\n 970 .format(type(self).__name__, k))\r\n--> 971 return func(v)\r\n 972 \r\n 973 with cbook._setattr_cm(self, eventson=False):\r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/matplotlib/collections.py in set_facecolor(self, c)\r\n 676 """\r\n 677 self._original_facecolor = c\r\n--> 678 self._set_facecolor(c)\r\n 679 \r\n 680 def get_facecolor(self):\r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/matplotlib/collections.py in _set_facecolor(self, c)\r\n 659 except AttributeError:\r\n 660 pass\r\n--> 661 self._facecolors = mcolors.to_rgba_array(c, self._alpha)\r\n 662 self.stale = True\r\n 663 \r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/matplotlib/colors.py in to_rgba_array(c, alpha)\r\n 292 result = np.empty((len(c), 4), float)\r\n 293 for i, cc in enumerate(c):\r\n--> 294 result[i] = to_rgba(cc, alpha)\r\n 295 return result\r\n 296 \r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/matplotlib/colors.py in to_rgba(c, alpha)\r\n 175 rgba = None\r\n 176 if rgba is None: # Suppress exception chaining of cache lookup failure.\r\n--> 177 rgba = _to_rgba_no_colorcycle(c, alpha)\r\n 178 try:\r\n 179 _colors_full_map.cache[c, alpha] = rgba\r\n\r\n~/miniconda3/envs/dev/lib/python3.7/site-packages/matplotlib/colors.py in _to_rgba_no_colorcycle(c, alpha)\r\n 238 # float)` and `np.array(...).astype(float)` all convert "0.5" to 0.5.\r\n 239 # Test dimensionality to reject single floats.\r\n--> 240 raise ValueError("Invalid RGBA argument: {!r}".format(orig_c))\r\n 241 # Return a tuple to prevent the cached value from being modified.\r\n 242 c = tuple(c.astype(float))\r\n\r\nValueError: Invalid RGBA argument: 0.0196078431372549```'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/95',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/95/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/95/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/95/events',
'html_url': 'https://github.com/pysal/splot/pull/95',
'id': 545467533,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzU5MzM2OTM4',
'number': 95,
'title': 'BUG: Fix breakage due to mapclassify deprecation',
'user': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2020-01-05T21:13:54Z',
'updated_at': '2020-01-07T23:48:38Z',
'closed_at': '2020-01-07T23:48:38Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/splot/pulls/95',
'html_url': 'https://github.com/pysal/splot/pull/95',
'diff_url': 'https://github.com/pysal/splot/pull/95.diff',
'patch_url': 'https://github.com/pysal/splot/pull/95.patch'},
'body': '[mapclassify 2.2.0](https://github.com/pysal/mapclassify/releases/tag/v2.2.0) removed deprecated class names.'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/93',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/93/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/93/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/93/events',
'html_url': 'https://github.com/pysal/splot/pull/93',
'id': 537804365,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzUzMDk2MjE5',
'number': 93,
'title': 'addressing pysal/pysal#1145 & adapting testing examples',
'user': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 588429868,
'node_id': 'MDU6TGFiZWw1ODg0Mjk4Njg=',
'url': 'https://api.github.com/repos/pysal/splot/labels/bug',
'name': 'bug',
'color': 'ee0701',
'default': True,
'description': None},
{'id': 1353566648,
'node_id': 'MDU6TGFiZWwxMzUzNTY2NjQ4',
'url': 'https://api.github.com/repos/pysal/splot/labels/doc',
'name': 'doc',
'color': '7D3C98',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 0,
'created_at': '2019-12-13T22:24:00Z',
'updated_at': '2019-12-29T00:15:35Z',
'closed_at': '2019-12-29T00:15:30Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/splot/pulls/93',
'html_url': 'https://github.com/pysal/splot/pull/93',
'diff_url': 'https://github.com/pysal/splot/pull/93.diff',
'patch_url': 'https://github.com/pysal/splot/pull/93.patch'},
'body': '* addressing the travis dual testing problem raised in pysal/pysal#1145\r\n* adapting tests to libpysal v4.2 examples schema'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/89',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/89/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/89/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/89/events',
'html_url': 'https://github.com/pysal/splot/pull/89',
'id': 522468317,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzQwNjY0MDk3',
'number': 89,
'title': 'Fix docstring for plot_spatial_weights',
'user': {'login': 'martinfleis',
'id': 36797143,
'node_id': 'MDQ6VXNlcjM2Nzk3MTQz',
'avatar_url': 'https://avatars2.githubusercontent.com/u/36797143?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/martinfleis',
'html_url': 'https://github.com/martinfleis',
'followers_url': 'https://api.github.com/users/martinfleis/followers',
'following_url': 'https://api.github.com/users/martinfleis/following{/other_user}',
'gists_url': 'https://api.github.com/users/martinfleis/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/martinfleis/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/martinfleis/subscriptions',
'organizations_url': 'https://api.github.com/users/martinfleis/orgs',
'repos_url': 'https://api.github.com/users/martinfleis/repos',
'events_url': 'https://api.github.com/users/martinfleis/events{/privacy}',
'received_events_url': 'https://api.github.com/users/martinfleis/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1353566648,
'node_id': 'MDU6TGFiZWwxMzUzNTY2NjQ4',
'url': 'https://api.github.com/repos/pysal/splot/labels/doc',
'name': 'doc',
'color': '7D3C98',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-11-13T20:56:45Z',
'updated_at': '2019-11-13T21:41:39Z',
'closed_at': '2019-11-13T21:41:39Z',
'author_association': 'CONTRIBUTOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/splot/pulls/89',
'html_url': 'https://github.com/pysal/splot/pull/89',
'diff_url': 'https://github.com/pysal/splot/pull/89.diff',
'patch_url': 'https://github.com/pysal/splot/pull/89.patch'},
'body': 'I noticed a minor issue with `plot_spatial_weights` docstring as it uses `Arguments` instead of `Parameters`, so it is not visible in [docs](https://splot.readthedocs.io/en/latest/generated/splot.libpysal.plot_spatial_weights.html#splot.libpysal.plot_spatial_weights).\r\n\r\nJust a side note, this second point in your PR template is no longer valid, right? `pysal/dev` is way behind master.\r\n\r\n> 2. [ ] This pull request is directed to the `pysal/dev` branch.\r\n\r\ncc https://github.com/openjournals/joss-reviews/issues/1882'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/59',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/59/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/59/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/59/events',
'html_url': 'https://github.com/pysal/splot/issues/59',
'id': 444595741,
'node_id': 'MDU6SXNzdWU0NDQ1OTU3NDE=',
'number': 59,
'title': 'JOSS paper submission',
'user': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1639142764,
'node_id': 'MDU6TGFiZWwxNjM5MTQyNzY0',
'url': 'https://api.github.com/repos/pysal/splot/labels/JOSS%20paper',
'name': 'JOSS paper',
'color': 'bfd4f2',
'default': False,
'description': ''},
{'id': 959244121,
'node_id': 'MDU6TGFiZWw5NTkyNDQxMjE=',
'url': 'https://api.github.com/repos/pysal/splot/labels/discussion',
'name': 'discussion',
'color': '38eabe',
'default': False,
'description': 'space for collecting ideas and discuss these'},
{'id': 588429870,
'node_id': 'MDU6TGFiZWw1ODg0Mjk4NzA=',
'url': 'https://api.github.com/repos/pysal/splot/labels/enhancement',
'name': 'enhancement',
'color': '84b6eb',
'default': True,
'description': None},
{'id': 1353562993,
'node_id': 'MDU6TGFiZWwxMzUzNTYyOTkz',
'url': 'https://api.github.com/repos/pysal/splot/labels/priority:%20high',
'name': 'priority: high',
'color': 'FF5733',
'default': False,
'description': 'needs to be resolved soon'},
{'id': 1044069993,
'node_id': 'MDU6TGFiZWwxMDQ0MDY5OTkz',
'url': 'https://api.github.com/repos/pysal/splot/labels/roadmap',
'name': 'roadmap',
'color': 'a85ae8',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'sjsrey',
'id': 118042,
'node_id': 'MDQ6VXNlcjExODA0Mg==',
'avatar_url': 'https://avatars1.githubusercontent.com/u/118042?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/sjsrey',
'html_url': 'https://github.com/sjsrey',
'followers_url': 'https://api.github.com/users/sjsrey/followers',
'following_url': 'https://api.github.com/users/sjsrey/following{/other_user}',
'gists_url': 'https://api.github.com/users/sjsrey/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/sjsrey/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/sjsrey/subscriptions',
'organizations_url': 'https://api.github.com/users/sjsrey/orgs',
'repos_url': 'https://api.github.com/users/sjsrey/repos',
'events_url': 'https://api.github.com/users/sjsrey/events{/privacy}',
'received_events_url': 'https://api.github.com/users/sjsrey/received_events',
'type': 'User',
'site_admin': False},
{'login': 'darribas',
'id': 417363,
'node_id': 'MDQ6VXNlcjQxNzM2Mw==',
'avatar_url': 'https://avatars3.githubusercontent.com/u/417363?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/darribas',
'html_url': 'https://github.com/darribas',
'followers_url': 'https://api.github.com/users/darribas/followers',
'following_url': 'https://api.github.com/users/darribas/following{/other_user}',
'gists_url': 'https://api.github.com/users/darribas/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/darribas/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/darribas/subscriptions',
'organizations_url': 'https://api.github.com/users/darribas/orgs',
'repos_url': 'https://api.github.com/users/darribas/repos',
'events_url': 'https://api.github.com/users/darribas/events{/privacy}',
'received_events_url': 'https://api.github.com/users/darribas/received_events',
'type': 'User',
'site_admin': False},
{'login': 'ljwolf',
'id': 2250995,
'node_id': 'MDQ6VXNlcjIyNTA5OTU=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/2250995?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/ljwolf',
'html_url': 'https://github.com/ljwolf',
'followers_url': 'https://api.github.com/users/ljwolf/followers',
'following_url': 'https://api.github.com/users/ljwolf/following{/other_user}',
'gists_url': 'https://api.github.com/users/ljwolf/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/ljwolf/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/ljwolf/subscriptions',
'organizations_url': 'https://api.github.com/users/ljwolf/orgs',
'repos_url': 'https://api.github.com/users/ljwolf/repos',
'events_url': 'https://api.github.com/users/ljwolf/events{/privacy}',
'received_events_url': 'https://api.github.com/users/ljwolf/received_events',
'type': 'User',
'site_admin': False},
{'login': 'weikang9009',
'id': 7359284,
'node_id': 'MDQ6VXNlcjczNTkyODQ=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/7359284?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/weikang9009',
'html_url': 'https://github.com/weikang9009',
'followers_url': 'https://api.github.com/users/weikang9009/followers',
'following_url': 'https://api.github.com/users/weikang9009/following{/other_user}',
'gists_url': 'https://api.github.com/users/weikang9009/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/weikang9009/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/weikang9009/subscriptions',
'organizations_url': 'https://api.github.com/users/weikang9009/orgs',
'repos_url': 'https://api.github.com/users/weikang9009/repos',
'events_url': 'https://api.github.com/users/weikang9009/events{/privacy}',
'received_events_url': 'https://api.github.com/users/weikang9009/received_events',
'type': 'User',
'site_admin': False},
{'login': 'TaylorOshan',
'id': 8117709,
'node_id': 'MDQ6VXNlcjgxMTc3MDk=',
'avatar_url': 'https://avatars1.githubusercontent.com/u/8117709?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/TaylorOshan',
'html_url': 'https://github.com/TaylorOshan',
'followers_url': 'https://api.github.com/users/TaylorOshan/followers',
'following_url': 'https://api.github.com/users/TaylorOshan/following{/other_user}',
'gists_url': 'https://api.github.com/users/TaylorOshan/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/TaylorOshan/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/TaylorOshan/subscriptions',
'organizations_url': 'https://api.github.com/users/TaylorOshan/orgs',
'repos_url': 'https://api.github.com/users/TaylorOshan/repos',
'events_url': 'https://api.github.com/users/TaylorOshan/events{/privacy}',
'received_events_url': 'https://api.github.com/users/TaylorOshan/received_events',
'type': 'User',
'site_admin': False},
{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
{'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/splot/milestones/1',
'html_url': 'https://github.com/pysal/splot/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/splot/milestones/1/labels',
'id': 4298019,
'node_id': 'MDk6TWlsZXN0b25lNDI5ODAxOQ==',
'number': 1,
'title': 'JOSS paper',
'description': 'submit splot as JOSS article',
'creator': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 2,
'closed_issues': 16,
'state': 'open',
'created_at': '2019-05-08T23:06:19Z',
'updated_at': '2020-01-18T20:24:38Z',
'due_on': '2019-07-01T07:00:00Z',
'closed_at': None},
'comments': 4,
'created_at': '2019-05-15T19:01:16Z',
'updated_at': '2019-11-12T17:06:27Z',
'closed_at': '2019-11-12T17:06:27Z',
'author_association': 'MEMBER',
'body': "@sjsrey and I are planning on submitting `splot` as a [JOSS paper](http://joss.theoj.org)\r\n\r\nI would like to invite you to join in on this project and publication. I leave it up to you to decide wether you think your contribution has been substantial enough to be listed as a co-author. Additionally, there are a couple more tasks to implement before submission in case you would like to up your contribution. Feel free to note down your name to whichever task you are most likely to be able to contribute to. Include your-self and your affiliation in the list of authors.\r\n\r\nDeadline for submission in July 1st.\r\n\r\nAn example submissions can be found [here](https://joss.readthedocs.io/en/latest/submitting.html#example-paper-and-bibliography) and I am also happy to help out with more questions. \r\n\r\n***To-Do's***\r\n\r\n**Package**: (due date June 10)\r\n- [x] test all lines of code not tested, see #57 (@slumnitz)\r\n~- [ ] choropleth option in splot.mapping, see #50~ (integration with mapclassify first)\r\n- [x] Moran scatterplot equal bounds, see #51 (@ljwolf, @slumnitz)\r\n- [ ] (optional?) environment.yml for easy install\r\n- [x] check notebooks (@slumnitz)\r\n- [ ] esda local tests plotting function providing an option for selecting correction procedue for multiple testing (FDR-based (related to https://github.com/pysal/esda/pull/52), Bonferroni correction, etc)? (@weikang9009) \r\n- [x] docs building: readthedocs configuration file migrates from v1 to v2 as suggested in readthedocs that v1 should not be used (similar to [giddy](https://github.com/pysal/giddy/pull/92)) (@weikang9009 https://github.com/pysal/splot/pull/64 )\r\n- [x] move from supporting python 3.5 and 3.6 to python 3.6 and 3.7 as suggested in the last pysal dev meeting? (@weikang9009 @jGaboardi https://github.com/pysal/splot/pull/63)\r\n- [x] set up dual travis tests againist pysal dependencies (pip and github version) (@weikang9009 https://github.com/pysal/splot/pull/69)\r\n(- [ ] mapclassify natural breaks maintenance #65 (@weikang9009 ))\r\n\r\nOnce everything is done: (due date June 12)\r\n- [x] release splot 1.1.0 on pipy (@slumnitz)\r\n- [x] release splot 1.1.0 on Conda-forge (@slumnitz)\r\n\r\n[**`paper.md`**](https://github.com/pysal/splot/tree/master/paper): (due date June 19)\r\n- [x] list of authors and affiliations (@slumnitz, @sjsrey )\r\n- [x] summary for non-specialist audience (@slumnitz)\r\n - [x] statement of need: target audience, problems the software can solve (I could use help here, @ljwolf can help)\r\n - [x] installation instructions\r\n - [ ] example usage for real world analysis problems or reference\r\n- [x] outlook/ roadmap (@slumnitz)\r\n- [x] key references (I could use help here) (@weikang9009)\r\n- [ ] statement of commit history ()\r\n- [x] Acknowledgements (@slumnitz)\r\n\r\nOnce draft is out:\r\n- [x] happy to review (@jGaboardi, )\r\n\r\n**`README.md`**: (due date June 10)\r\n- [x] link to CoC, (@slumnitz)\r\n- [x] description on how to contribute (@slumnitz)\r\n- [x] guidlines for reporting issues and participation (@slumnitz)\r\n- [x] update dependencies (@slumnitz)\r\n- [x] include Conda-forge installation (@slumnitz)\r\n- [x] refer to examples (@slumnitz)\r\n- [x] update installation instructions once release is out\r\n- [x] include DOI once release is out (https://zenodo.org/)\r\n\r\nLooking forward to getting this ready with you! Please feel free to add ideas and other tasks if you see fit :)"},
{'url': 'https://api.github.com/repos/pysal/splot/issues/87',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/87/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/87/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/87/events',
'html_url': 'https://github.com/pysal/splot/pull/87',
'id': 514636030,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzM0MjIyOTQ3',
'number': 87,
'title': 'Fix format for multiple citations in JOSS paper',
'user': {'login': 'leouieda',
'id': 290082,
'node_id': 'MDQ6VXNlcjI5MDA4Mg==',
'avatar_url': 'https://avatars0.githubusercontent.com/u/290082?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/leouieda',
'html_url': 'https://github.com/leouieda',
'followers_url': 'https://api.github.com/users/leouieda/followers',
'following_url': 'https://api.github.com/users/leouieda/following{/other_user}',
'gists_url': 'https://api.github.com/users/leouieda/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/leouieda/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/leouieda/subscriptions',
'organizations_url': 'https://api.github.com/users/leouieda/orgs',
'repos_url': 'https://api.github.com/users/leouieda/repos',
'events_url': 'https://api.github.com/users/leouieda/events{/privacy}',
'received_events_url': 'https://api.github.com/users/leouieda/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1639142764,
'node_id': 'MDU6TGFiZWwxNjM5MTQyNzY0',
'url': 'https://api.github.com/repos/pysal/splot/labels/JOSS%20paper',
'name': 'JOSS paper',
'color': 'bfd4f2',
'default': False,
'description': ''},
{'id': 1353562993,
'node_id': 'MDU6TGFiZWwxMzUzNTYyOTkz',
'url': 'https://api.github.com/repos/pysal/splot/labels/priority:%20high',
'name': 'priority: high',
'color': 'FF5733',
'default': False,
'description': 'needs to be resolved soon'}],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': {'url': 'https://api.github.com/repos/pysal/splot/milestones/1',
'html_url': 'https://github.com/pysal/splot/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/splot/milestones/1/labels',
'id': 4298019,
'node_id': 'MDk6TWlsZXN0b25lNDI5ODAxOQ==',
'number': 1,
'title': 'JOSS paper',
'description': 'submit splot as JOSS article',
'creator': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 2,
'closed_issues': 16,
'state': 'open',
'created_at': '2019-05-08T23:06:19Z',
'updated_at': '2020-01-18T20:24:38Z',
'due_on': '2019-07-01T07:00:00Z',
'closed_at': None},
'comments': 2,
'created_at': '2019-10-30T12:38:59Z',
'updated_at': '2019-10-31T09:12:29Z',
'closed_at': '2019-10-30T20:09:52Z',
'author_association': 'CONTRIBUTOR',
'pull_request': {'url': 'https://api.github.com/repos/pysal/splot/pulls/87',
'html_url': 'https://github.com/pysal/splot/pull/87',
'diff_url': 'https://github.com/pysal/splot/pull/87.diff',
'patch_url': 'https://github.com/pysal/splot/pull/87.patch'},
'body': 'Multiple citations are separated with a `;` instead of `,` which was\r\ncausing the paper to not render properly. Also fixes the missing\r\ncitation of `Arribas-Bel2017` to `arribas-bel2017looking` which I assume\r\nis what was intended (feel free to change if this is wrong).\r\n\r\nRelated to openjournals/joss-reviews#1838\r\n\r\nHello! Please make sure to check all these boxes before submitting a Pull Request\r\n(PR). Once you have checked the boxes, feel free to remove all text except the\r\njustification in point 5. \r\n\r\n1. [x] You have run tests on this submission, either by using [Travis Continuous Integration testing](https://github.com/pysal/pysal/wiki/GitHub-Standard-Operating-Procedures#automated-testing-w-travis-ci) testing or running `nosetests` on your changes?\r\n2. [ ] This pull request is directed to the `pysal/dev` branch.\r\n3. [ ] This pull introduces new functionality covered by\r\n [docstrings](https://en.wikipedia.org/wiki/Docstring#Python) and\r\n [unittests](https://docs.python.org/2/library/unittest.html)? \r\n4. [ ] You have [assigned a\r\n reviewer](https://help.github.com/articles/assigning-issues-and-pull-requests-to-other-github-users/) and added relevant [labels](https://help.github.com/articles/applying-labels-to-issues-and-pull-requests/)\r\n5. [ ] The justification for this PR is: Fixes JOSS reference formatting issues.\r\n'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/86',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/86/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/86/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/86/events',
'html_url': 'https://github.com/pysal/splot/pull/86',
'id': 512772501,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzMyNzIyNzI5',
'number': 86,
'title': 'Joss paper, finalise title',
'user': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1639142764,
'node_id': 'MDU6TGFiZWwxNjM5MTQyNzY0',
'url': 'https://api.github.com/repos/pysal/splot/labels/JOSS%20paper',
'name': 'JOSS paper',
'color': 'bfd4f2',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'jGaboardi',
'id': 8590583,
'node_id': 'MDQ6VXNlcjg1OTA1ODM=',
'avatar_url': 'https://avatars3.githubusercontent.com/u/8590583?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/jGaboardi',
'html_url': 'https://github.com/jGaboardi',
'followers_url': 'https://api.github.com/users/jGaboardi/followers',
'following_url': 'https://api.github.com/users/jGaboardi/following{/other_user}',
'gists_url': 'https://api.github.com/users/jGaboardi/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/jGaboardi/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/jGaboardi/subscriptions',
'organizations_url': 'https://api.github.com/users/jGaboardi/orgs',
'repos_url': 'https://api.github.com/users/jGaboardi/repos',
'events_url': 'https://api.github.com/users/jGaboardi/events{/privacy}',
'received_events_url': 'https://api.github.com/users/jGaboardi/received_events',
'type': 'User',
'site_admin': False},
{'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/splot/milestones/1',
'html_url': 'https://github.com/pysal/splot/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/splot/milestones/1/labels',
'id': 4298019,
'node_id': 'MDk6TWlsZXN0b25lNDI5ODAxOQ==',
'number': 1,
'title': 'JOSS paper',
'description': 'submit splot as JOSS article',
'creator': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 2,
'closed_issues': 16,
'state': 'open',
'created_at': '2019-05-08T23:06:19Z',
'updated_at': '2020-01-18T20:24:38Z',
'due_on': '2019-07-01T07:00:00Z',
'closed_at': None},
'comments': 0,
'created_at': '2019-10-26T01:12:07Z',
'updated_at': '2019-10-26T01:23:18Z',
'closed_at': '2019-10-26T01:23:18Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/splot/pulls/86',
'html_url': 'https://github.com/pysal/splot/pull/86',
'diff_url': 'https://github.com/pysal/splot/pull/86.diff',
'patch_url': 'https://github.com/pysal/splot/pull/86.patch'},
'body': '* finalise paper format and change title to fit one line'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/62',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/62/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/62/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/62/events',
'html_url': 'https://github.com/pysal/splot/pull/62',
'id': 445211187,
'node_id': 'MDExOlB1bGxSZXF1ZXN0Mjc5NzExMTY1',
'number': 62,
'title': '[JOSS] work on `paper.md`',
'user': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'labels': [{'id': 1639142764,
'node_id': 'MDU6TGFiZWwxNjM5MTQyNzY0',
'url': 'https://api.github.com/repos/pysal/splot/labels/JOSS%20paper',
'name': 'JOSS paper',
'color': 'bfd4f2',
'default': False,
'description': ''}],
'state': 'closed',
'locked': False,
'assignee': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False}],
'milestone': {'url': 'https://api.github.com/repos/pysal/splot/milestones/1',
'html_url': 'https://github.com/pysal/splot/milestone/1',
'labels_url': 'https://api.github.com/repos/pysal/splot/milestones/1/labels',
'id': 4298019,
'node_id': 'MDk6TWlsZXN0b25lNDI5ODAxOQ==',
'number': 1,
'title': 'JOSS paper',
'description': 'submit splot as JOSS article',
'creator': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'open_issues': 2,
'closed_issues': 16,
'state': 'open',
'created_at': '2019-05-08T23:06:19Z',
'updated_at': '2020-01-18T20:24:38Z',
'due_on': '2019-07-01T07:00:00Z',
'closed_at': None},
'comments': 6,
'created_at': '2019-05-17T00:18:06Z',
'updated_at': '2019-10-26T01:21:08Z',
'closed_at': '2019-10-26T00:52:54Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/splot/pulls/62',
'html_url': 'https://github.com/pysal/splot/pull/62',
'diff_url': 'https://github.com/pysal/splot/pull/62.diff',
'patch_url': 'https://github.com/pysal/splot/pull/62.patch'},
'body': '* add first outline\r\n* add paragraph 4\r\n\r\n@ljwolf When we started GSOC it seemed to me that splots main need for users was to visualise PySAL objects. Perhaps it therefore makes sense to introduce the need of spatial analysis with a practical example?\r\n\r\n'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/85',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/85/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/85/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/85/events',
'html_url': 'https://github.com/pysal/splot/pull/85',
'id': 502889197,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI0ODg2MjY5',
'number': 85,
'title': '[ENH] change doc badge to latest doc',
'user': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-10-05T00:04:11Z',
'updated_at': '2019-10-05T04:16:13Z',
'closed_at': '2019-10-05T04:16:13Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/splot/pulls/85',
'html_url': 'https://github.com/pysal/splot/pull/85',
'diff_url': 'https://github.com/pysal/splot/pull/85.diff',
'patch_url': 'https://github.com/pysal/splot/pull/85.patch'},
'body': 'Change splot doc badge to latest version instead of stable version for JOSS submission.\r\n\r\nFor some reason the stable version points to the old pysal documentation.'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/84',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/84/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/84/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/84/events',
'html_url': 'https://github.com/pysal/splot/pull/84',
'id': 502754377,
'node_id': 'MDExOlB1bGxSZXF1ZXN0MzI0Nzc2OTUw',
'number': 84,
'title': '[BUG] require geopandas>=0.4.0,<=0.6.0rc1 for vba_choropleth testing',
'user': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': None,
'assignees': [],
'milestone': None,
'comments': 0,
'created_at': '2019-10-04T17:24:58Z',
'updated_at': '2019-10-04T18:43:35Z',
'closed_at': '2019-10-04T18:43:35Z',
'author_association': 'MEMBER',
'pull_request': {'url': 'https://api.github.com/repos/pysal/splot/pulls/84',
'html_url': 'https://github.com/pysal/splot/pull/84',
'diff_url': 'https://github.com/pysal/splot/pull/84.diff',
'patch_url': 'https://github.com/pysal/splot/pull/84.patch'},
'body': 'add `,<=0.6.0rc1` in `requirements.txt` to allow testing on travis\r\n\r\nNote: this is a work-around solution, the bug will be addressed in cooperation with GeoPandas team'},
{'url': 'https://api.github.com/repos/pysal/splot/issues/82',
'repository_url': 'https://api.github.com/repos/pysal/splot',
'labels_url': 'https://api.github.com/repos/pysal/splot/issues/82/labels{/name}',
'comments_url': 'https://api.github.com/repos/pysal/splot/issues/82/comments',
'events_url': 'https://api.github.com/repos/pysal/splot/issues/82/events',
'html_url': 'https://github.com/pysal/splot/issues/82',
'id': 488286893,
'node_id': 'MDU6SXNzdWU0ODgyODY4OTM=',
'number': 82,
'title': '`plot_moran_simulation` weird dimensions',
'user': {'login': 'darribas',
'id': 417363,
'node_id': 'MDQ6VXNlcjQxNzM2Mw==',
'avatar_url': 'https://avatars3.githubusercontent.com/u/417363?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/darribas',
'html_url': 'https://github.com/darribas',
'followers_url': 'https://api.github.com/users/darribas/followers',
'following_url': 'https://api.github.com/users/darribas/following{/other_user}',
'gists_url': 'https://api.github.com/users/darribas/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/darribas/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/darribas/subscriptions',
'organizations_url': 'https://api.github.com/users/darribas/orgs',
'repos_url': 'https://api.github.com/users/darribas/repos',
'events_url': 'https://api.github.com/users/darribas/events{/privacy}',
'received_events_url': 'https://api.github.com/users/darribas/received_events',
'type': 'User',
'site_admin': False},
'labels': [],
'state': 'closed',
'locked': False,
'assignee': {'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False},
'assignees': [{'login': 'slumnitz',
'id': 35147471,
'node_id': 'MDQ6VXNlcjM1MTQ3NDcx',
'avatar_url': 'https://avatars2.githubusercontent.com/u/35147471?v=4',
'gravatar_id': '',
'url': 'https://api.github.com/users/slumnitz',
'html_url': 'https://github.com/slumnitz',
'followers_url': 'https://api.github.com/users/slumnitz/followers',
'following_url': 'https://api.github.com/users/slumnitz/following{/other_user}',
'gists_url': 'https://api.github.com/users/slumnitz/gists{/gist_id}',
'starred_url': 'https://api.github.com/users/slumnitz/starred{/owner}{/repo}',
'subscriptions_url': 'https://api.github.com/users/slumnitz/subscriptions',
'organizations_url': 'https://api.github.com/users/slumnitz/orgs',
'repos_url': 'https://api.github.com/users/slumnitz/repos',
'events_url': 'https://api.github.com/users/slumnitz/events{/privacy}',
'received_events_url': 'https://api.github.com/users/slumnitz/received_events',
'type': 'User',
'site_admin': False}],
'milestone': None,
'comments': 1,
'created_at': '2019-09-02T18:21:09Z',
'updated_at': '2019-09-02T18:24:28Z',
'closed_at': '2019-09-02T18:24:27Z',
'author_association': 'MEMBER',
'body': 'With the following preamble:\r\n\r\n```python\r\n%matplotlib inline\r\nimport matplotlib.pyplot as plt\r\nfrom pysal.viz.splot import esda as esdaplot\r\nfrom pysal.explore import esda\r\nfrom pysal.lib import weights\r\nimport geopandas\r\n\r\ndb = geopandas.read_file("https://github.com/rsbivand/ectqg19-workshop/raw/master/data/lux_regions.gpkg")\r\nw = weights.Queen.from_dataframe(db)\r\nw.transform = "R"\r\n\r\nmi = esda.Moran(db["light_level"], w)\r\n```\r\n\r\nThe following produces this output:\r\n\r\n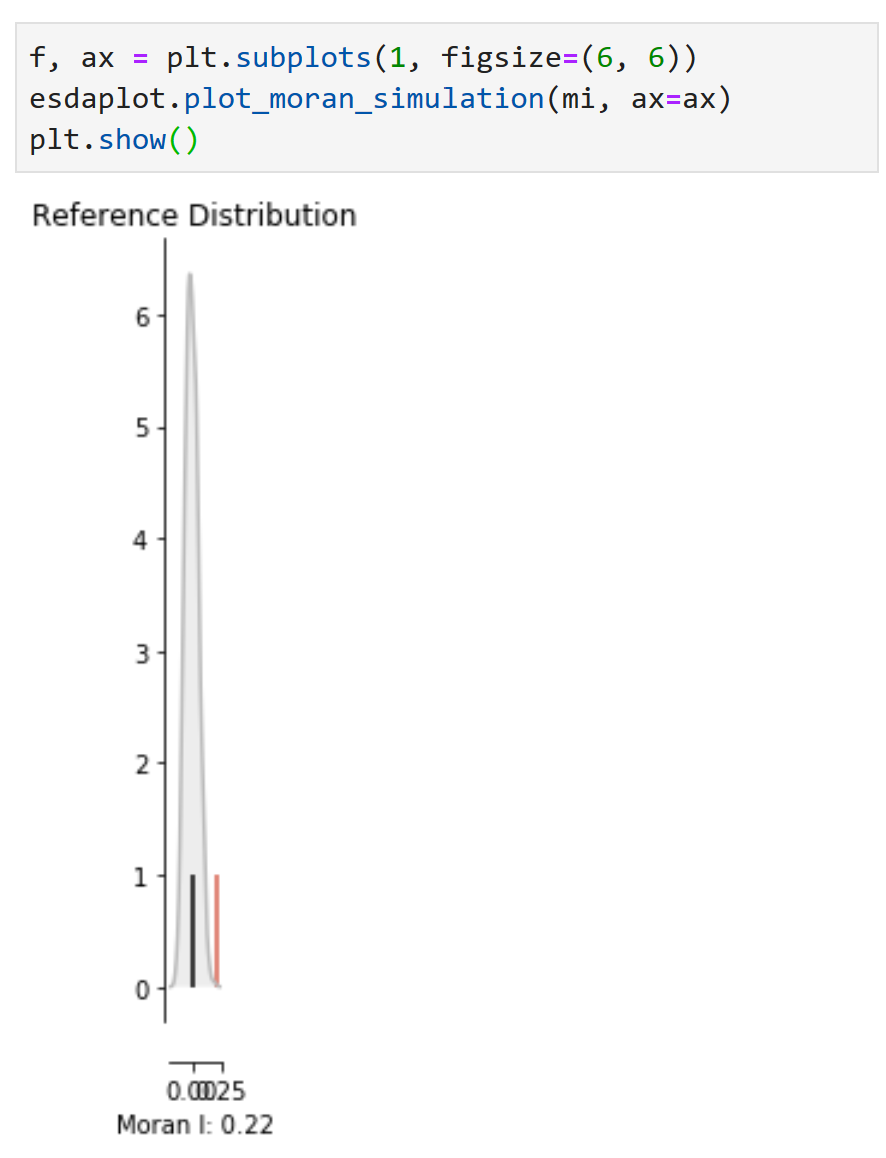\r\n\r\nShouldn\'t the figure by 6x6? Also, why is it so narrow (if you don\'t set up the `ax`, it gives the same image)?'}]}
The issues are pulled since the last release date of the meta package. However, each package that is going into the meta release, has a specific release tag that pins the code making it into the release. We don't want to report the commits post the packages tag date so we have to do some filtering here before building our change log statistics for the meta package.
For now let's pickle the issues and pull records to filter later and not have to rehit github api
In [26]:
import pickle
pickle.dump( issue_details, open( "issue_details.p", "wb" ) )
pickle.dump( pull_details, open("pull_details.p", "wb"))
In [ ]:
Content source: weikang9009/pysal
Similar notebooks: